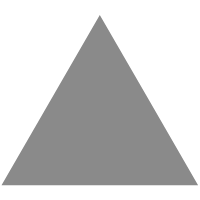
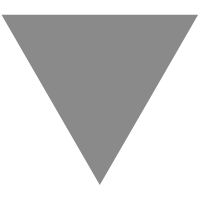
基于node实现文件路径替换 · Issue #24 · Aaaaaaaty/Blog · GitHub
source link: https://github.com/Aaaaaaaty/Blog/issues/24?
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
本次分享一个简易路径替换工具。功能很简单,重点在于掌握:
- 递归遍历文件夹目录
- 正则替换目标内容
- 解压上传文件,返回更新后的压缩文件
源码地址:https://github.com/Aaaaaaaty/Blog/tree/master/fsPathSys
结果对比图:
PS:后端支持匹配js、css、img、background-image的url的对应路径并进行分别替换,当前只是展示方便,前端只传递一个路径将所有匹配的源路径替换为目标路径。
- 前端上传压缩包及需要替换的路径字段
- 后端解压缩
- 递归文件目录,找到.js/.css/.html文件并匹配替换路径
- 压缩整体文件,返回到前端
整体来说可能会遇到的难点在于对正则的使用,以及完成替换后将压缩的文件夹传回本地。以前没怎么写过正则正好借此机会来学习一波,同时对于文件夹(注意不是文件传输!)传输踩了一下坑。毕竟大部分时间做静态服务器我们是只需要返回单个文件不需要以一个文件夹的形式来返回到前端。
解压缩zip
在nodejs文档中发现原生api貌似只支持gzip的解压缩,故引入了第三方插件unzip来解决。
let inp = fs.createReadStream(path)
let extract = unzip.Extract({ path: targetPath })
inp.pipe(extract)
extract.on('error', () => {
cons('解压出错:' + err);
})
extract.on('close', () => {
cons('解压完成');
})
这个插件有一点坑的地方在于它没有说明如何监听'close'、'error'等事件。还是我去看源码里面发现要通过上面的形式来调用才能成功:)
递归文件目录
通过fs模块的stat方法来判断当前路径是文件还是文件夹来决定是否继续遍历。
function fsPathSys(path) { //遍历路径
let stat = fs.statSync(path)
if(stat.isDirectory()) {
fs.readdir(path, isDirectory) //读文件夹
function isDirectory(err, files) {
if(err) {
return err
} else {
files.forEach((item, index) => {
let nowPath = `${path}/${item}`
let stat = fs.statSync(nowPath)
if(!stat.isDirectory()) {
...somthing going on
} else {
fsPathSys(nowPath)
}
})
}
}
}
else {
...
}
}
正则的重点则在于如何匹配到需要的地方,以及替换的顺序也需要有所考量。
本次需要匹配的地方有四个:
- script标签下的src
- link标签下的href
- img标签下的src
- css中background-image下的url
由于目标地址前的关键字src、href可能在不同的标签中,同时最初的想法就是有可能不同类型的文件的存放地址是不同的。故采用的匹配原则是先将script、link、img、background提取出来,然后再分别匹配src、href、url关键字。
//body:要替换的文本
let data = [
{
'type': 'script',
'point': targetUrl
},
{
'type': 'link',
'point': targetUrl
},
{
'type': 'img',
'point': targetUrl
},
{
'type': 'background',
'point': targetUrl
}
]
data.forEach((obj, i) => {
if(obj.type === 'script' || obj.type === 'link' || obj.type === 'img') {
let bodyMatch = body.match(new RegExp(`<${obj.type}.*?>`, 'g'))
if(bodyMatch) {
bodyMatch.forEach((item, index) => {
let itemMatch = item.match(/(src|href)\s*=\s*["|'].*?["|']/g)
if(itemMatch) {
itemMatch.forEach((data, i) => {
let matchItem = data.match(/(["|']).*\//g)[0].replace(/\s/g, '').slice(1)
if(!replaceBody[matchItem]) {
replaceBody[matchItem] = obj.point
}
})
}
})
}
} else if(obj.type === 'background') {
let bodyMatch = body.match(/url\(.*?\)/g)
if(bodyMatch) {
bodyMatch.forEach((item, index) => {
let itemMatch = item.match(/\(.*\//g)[0].replace(/\s/g, '').slice(1)
if(!replaceBody[itemMatch]) {
replaceBody[itemMatch] = obj.point
}
})
}
}
})
其中关于正则的使用可以参考这篇文章JS正则表达式完整教程(略长) 真的是非常详细,我就不班门弄斧了。总的来说上面的代码得到了一个对象,replaceBody。这个对象的key是要替换的路径,value是替换后的路径:
细心的童鞋可能会发现,如果现在直接遍历这个对象进行替换是不是就能大功告成了呢?肯定不是的:)因为替换要有先后顺序,不然会有大麻烦。
例如我们将要替换'../css/'以及'./css/',如果我们先替换后者那么之前的'../css/中的'./css/'也会被换掉从而整体替换失败这并不是我们想要的结果。
目前的做法是将对象中的key排序,长的在前,之后再进行替换。这样至少不会出现上面所提到的情况。
Object.keys(replaceBody).sort((a,b) => b.length - a.length) //对对象排序
另外还需要注意一个小点即在替换'.'的时候,由于'.'在正则中表示通配符。那么此时需要先将所有的'.'替换为'.'再进行下面的操作。
压缩整体文件,返回到前端
考虑到现在要传回前端的是一个文件夹,故要对其进行压缩。采用开启子进程的方式来编写shell命令来压缩文件夹。(node的zlib模块我没找到怎么来压缩文件夹。。有知道的同学欢迎分享)
let dirName = `${filePath}.tar.gz`
exec(`tar -zcvf ${dirName} ${filePath}`, (error, stdout, stderr) => {
if (error) {
cons(`exec error: ${error}`);
return;
}
let out = fs.createReadStream(dirName)
res.writeHead(200, {
'Content-type':'application/octet-stream',
'Content-Disposition': 'attachment; filename=' + dirName.match(/ip_.*/)[0]
})
out.pipe(res)
})
这里的重点是将压缩包用流的形式读取出来如果不在返回头加入'Content-Disposition'字段,返回的文件将是那种类似buffer流的形式,没有了文件夹层级结构等等。。查阅了资料才发现是因为这个头的缘故。
Content-disposition 是 MIME 协议的扩展,MIME 协议指示 MIME 用户代理如何显示附加的文件。
本次实现这个小工具,使作者正则还有文件在后端的压缩解压以及http传输中的细节有了新的认识。源代码在git上欢迎clone~
惯例po作者的博客,不定时更新中——
有问题欢迎在issues下交流。
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK