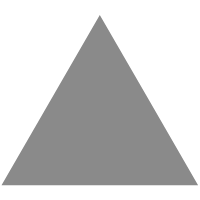
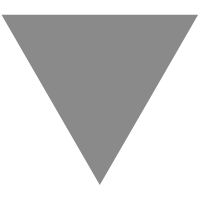
GitHub - sindresorhus/ow: Argument type validation
source link: https://github.com/sindresorhus/ow
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
readme.md
Argument type validation
Install
$ npm install ow
Usage
import ow from 'ow'; const unicorn = input => { ow(input, ow.string.minLength(5)); // … }; unicorn(3); //=> ArgumentError: Expected argument to be of type `string` but received type `number` unicorn('yo'); //=> ArgumentError: Expected string to have a minimum length of `5`, got `yo`
API
ow(value, predicate)
Test if value
matches the provided predicate
.
ow.create(predicate)
Create a reusable validator.
const checkPassword = ow.create(ow.string.minLength(6)); checkPassword('foo'); //=> ArgumentError: Expected string to have a minimum length of `6`, got `foo`
ow.any(...predicate[])
Returns a predicate that verifies if the value matches at least one of the given predicates.
ow('foo', ow.any(ow.string.maxLength(3), ow.number));
ow.{type}
All the below types return a predicate. Every predicate has some extra operators that you can use to test the value even more fine-grained.
Primitives
Built-in types
Typed arrays
int8Array
uint8Array
uint8ClampedArray
int16Array
uint16Array
int32Array
uint32Array
float32Array
float64Array
Structured data
Miscellaneous
Predicates
The following predicates are available on every type.
not
Inverts the following predicates.
m(1, m.number.not.infinite); m('', m.string.not.empty); //=> ArgumentError: [NOT] Expected string to be empty, got ``
is(fn)
Use a custom validation function. Return true
if the value matches the validation, return false
if it doesn't.
m(1, m.number.is(x => x < 10)); m(1, m.number.is(x => x > 10)); //=> ArgumentError: Expected `1` to pass custom validation function
Instead of returning false
, you can also return a custom error message which results in a failure.
const greaterThan = (max: number, x: number) => { return x > max || `Expected \`${x}\` to be greater than \`${max}\``; }; m(5, m.number.is(x => greaterThan(10, x))); //=> ArgumentError: Expected `5` to be greater than `10`
Maintainers
Related
- @sindresorhus/is - Type check values
License
MIT
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK