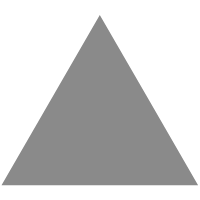
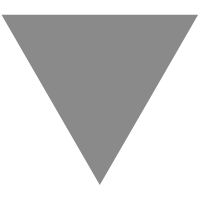
GitHub - taketo1024/SwiftyAlgebra
source link: https://github.com/taketo1024/SwiftyAlgebra
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
README.md
Swifty Algebra
A Swift playground for Abstract Algebra. This project is intended to understand Algebra by implementing abstract concepts and playing with concrete objects such as Numbers, Matrices, Polynomials, etc.
How to Build / Run
Open SwiftyAlgebra.xcworkspace
and press ▶️ to build the framework.
You can run the playgrounds under the project.
Creating Your Own Project
1. Initialize a Package
$ mkdir YourProject
$ cd YourProject
$ swift package init --type executable
2. Edit Package.swift
// swift-tools-version:4.0 // The swift-tools-version declares the minimum version of Swift required to build this package. import PackageDescription let package = Package( name: "YourProject", dependencies: [ // Dependencies declare other packages that this package depends on. - // .package(url: /* package url */, from: "1.0.0"), + .package(url: "https://github.com/taketo1024/SwiftyAlgebra.git", from: "0.1.0"), ], targets: [ // Targets are the basic building blocks of a package. A target can define a module or a test suite. // Targets can depend on other targets in this package, and on products in packages which this package depends on. .target( name: "YourProject", - dependencies: []), + dependencies: ["SwiftyAlgebra", "SwiftyTopology"]), ] )
3. Edit Sources/YourProject/main.swift
import SwiftyAlgebra import SwiftyTopology let S2 = SimplicialComplex.sphere(dim: 2) let H = Homology(S2, ?.self) print(S2.detailDescription) print(H.detailDescription)
4. Run
$ swift run
S^2 {
0: v₁, v₂, v₃, v₀
1: (v₁, v₂), (v₁, v₃), (v₂, v₃), (v₀, v₂), (v₀, v₃), (v₀, v₁)
2: (v₁, v₂, v₃), (v₀, v₂, v₃), (v₀, v₁, v₃), (v₀, v₁, v₂)
}
H(S^2; ?) = {
0 : ?, [v₀],
1 : 0, [],
2 : ?, [(v₀, v₂, v₃) + -1(v₁, v₂, v₃) + -1(v₀, v₁, v₃) + (v₀, v₁, v₂)]
}
You can use this Sample Project for a template.
Using Mathematical Symbols
We make use of mathematical symbols such as sets ?, ?, ?, ? and operators ⊕, ⊗ etc. Copy the folder CodeSnippets
to ~/Library/Xcode/UserData/
then you can quickly input these symbols by the completion of Xcode.
Examples
Rational Numbers
let a = ?(4, 5) // 4/5 let b = ?(3, 2) // 3/2 a + b // 23/10 a * b // 6/5 b / a // 15/8
Matrices (type safe)
typealias M = Matrix<_2, _2, ?> // Matrix of integers with fixed size 2×2. let a = M(1, 2, 3, 4) // [1, 2; 3, 4] let b = M(2, 1, 1, 2) // [2, 1; 1, 2] a + b // [3, 3; 4, 6] a * b // [4, 5; 10, 11] a + b == b + a // true: addition is commutative a * b == b * a // false: multiplication is noncommutative
Permutation (Symmetric Group)
typealias S_5 = Permutation<_5> let s = S_5(cyclic: 0, 1, 2) // cyclic notation let t = S_5([0: 2, 1: 3, 2: 4, 3: 0, 4: 1]) // two-line notation s[1] // 2 t[2] // 4 (s * t)[3] // 3 -> 0 -> 1 (t * s)[3] // 3 -> 3 -> 0
Polynomials
typealias P = Polynominal<?> let f = P(0, 2, -3, 1) // x^3 − 3x^2 + 2x let g = P(6, -5, 1) // x^2 − 5x + 6 f + g // x^3 - 2x^2 - 3x + 6 f * g // x^5 - 8x^4 + 23x^3 - 28x^2 + 12x f % g // 6x - 12 gcd(f, g) // 6x - 12
Integer Quotients, Finite Fields
typealias Z_4 = IntegerQuotientRing<_4> Z_4.printAddTable()
+ | 0 1 2 3
----------------------
0 | 0 1 2 3
1 | 1 2 3 0
2 | 2 3 0 1
3 | 3 0 1 2
typealias F_5 = IntegerQuotientField<_5> F_5.printMulTable()
* | 0 1 2 3 4
--------------------------
0 | 0 0 0 0 0
1 | 0 1 2 3 4
2 | 0 2 4 1 3
3 | 0 3 1 4 2
4 | 0 4 3 2 1
Algebraic Extension
// Construct an algebraic extension over ?: // K = ?(√2) = ?[x]/(x^2 - 2). struct p: _Polynomial { // p = x^2 - 2, as a struct typealias K = ? static let value = Polynomial<?>(-2, 0, 1) } typealias I = PolynomialIdeal<p> // I = (x^2 - 2) typealias K = QuotientField<Polynomial<?>, I> // K = ?[x]/I let a = Polynomial<?>(0, 1).asQuotient(in: K.self) // a = x mod I a * a == 2 // true!
Homology, Cohomology
let S2 = SimplicialComplex.sphere(dim: 2) let H = Homology(S2, ?.self) print("H(S^2; ?) =", H.detailDescription, "\n")
H(S^2; ?) = {
0 : ?, [(v1)],
1 : 0, [],
2 : ?, [-1(v0, v2, v3) + -1(v0, v1, v2) + (v1, v2, v3) + (v0, v1, v3)]
}
let RP2 = SimplicialComplex.realProjectiveSpace(dim: 2) let H = Homology(RP2, ?₂.self) print("H(RP^2; ?₂) =", H.detailDescription, "\n")
H(RP^2; ?₂) = {
0 : ?₂, [(v1)],
1 : ?₂, [(v0, v1) + (v1, v2) + (v0, v3) + (v2, v3)],
2 : ?₂, [(v0, v2, v3) + (v3, v4, v5) + (v2, v3, v5) + (v1, v2, v5) + (v0, v4, v5) + (v1, v3, v4) + (v0, v1, v5) + (v1, v2, v4) + (v0, v2, v4) + (v0, v1, v3)]
}
References
License
Swifty Algebra is licensed under CC0 1.0 Universal.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK