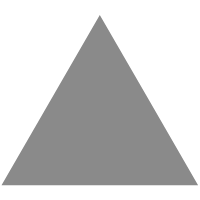
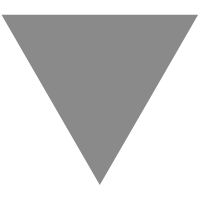
GitHub - GraphQLSwift/GraphQL: The Swift GraphQL implementation for macOS and Li...
source link: https://github.com/GraphQLSwift/GraphQL
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
README.md
GraphQL
The Swift implementation for GraphQL, a query language for APIs created by Facebook.
Looking for help? Find resources from the community.
Graphiti
This repo contains the core GraphQL implementation. For a better experience when creating your types use Graphiti.
Graphiti is a Swift library for building GraphQL schemas/types fast, safely and easily.
Getting Started
An overview of GraphQL in general is available in the README for the Specification for GraphQL. That overview describes a simple set of GraphQL examples that exist as tests in this repository. A good way to get started with this repository is to walk through that README and the corresponding tests in parallel.
Using GraphQL
Add GraphQL to your Package.swift
import PackageDescription let package = Package( dependencies: [ .Package(url: "https://github.com/GraphQLSwift/GraphQL.git", majorVersion: 0), ] )
GraphQL provides two important capabilities: building a type schema, and serving queries against that type schema.
First, build a GraphQL type schema which maps to your code base.
import GraphQL let schema = try GraphQLSchema( query: GraphQLObjectType( name: "RootQueryType", fields: [ "hello": GraphQLField( type: GraphQLString, resolve: { _, _, _, _ in "world" } ) ] ) )
This defines a simple schema with one type and one field, that resolves to a fixed value. More complex examples are included in the Tests directory.
Then, serve the result of a query against that type schema.
let query = "{ hello }" let result = try graphql(schema: schema, request: query) print(result)
Output:
{ "data": { "hello": "world" } }
This runs a query fetching the one field defined. The graphql
function will
first ensure the query is syntactically and semantically valid before executing
it, reporting errors otherwise.
let query = "{ boyhowdy }" let result = try graphql(schema: schema, request: query) print(result)
Output:
{ "errors": [ { "locations": [ { "line": 1, "column": 3 } ], "message": "Cannot query field \"boyhowdy\" on type \"RootQueryType\"." } ] }
Field Execution Strategies
Depending on your needs you can alter the field execution strategies used for field value resolution.
By default the SerialFieldExecutionStrategy
is used for all operation types (query
, mutation
, subscription
).
To use a different strategy simply provide it to the graphql
function:
try graphql( queryStrategy: ConcurrentDispatchFieldExecutionStrategy(), schema: schema, request: query )
The following strategies are available:
SerialFieldExecutionStrategy
ConcurrentDispatchFieldExecutionStrategy
Please note: Not all strategies are applicable for all operation types.
License
This project is released under the MIT license. See LICENSE for details.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK