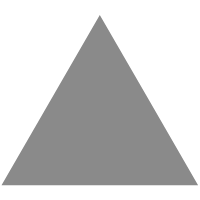
101
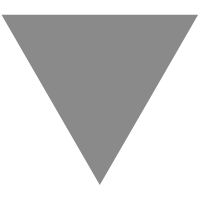
ES6的7个实用技巧 - 一步前端 - SegmentFault
source link: https://segmentfault.com/a/1190000012871249
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
7 Hacks for ES6 Developers
Hack #1 交换元素
利用数组解构
来实现值的互换
let a = 'world', b = 'hello' [a, b] = [b, a] console.log(a) // -> hello console.log(b) // -> world
Hack #2 调试
我们经常使用console.log()
来进行调试,试试console.table()
也无妨。
const a = 5, b = 6, c = 7 console.log({ a, b, c }); console.table({a, b, c, m: {name: 'xixi', age: 27}});
Hack #3 单条语句
ES6时代,操作数组的语句将会更加的紧凑
// 寻找数组中的最大值 const max = (arr) => Math.max(...arr); max([123, 321, 32]) // outputs: 321 // 计算数组的总和 const sum = (arr) => arr.reduce((a, b) => (a + b), 0) sum([1, 2, 3, 4]) // output: 10
Hack #4 数组拼接
展开运算符可以取代concat
的地位了
const one = ['a', 'b', 'c'] const two = ['d', 'e', 'f'] const three = ['g', 'h', 'i'] const result = [...one, ...two, ...three]
Hack #5 制作副本
我们可以很容易的实现数组和对象的浅拷贝
拷贝
const obj = { ...oldObj } const arr = [ ...oldArr ]
拷贝
= 深拷贝
? 浅拷贝
?
好像有些朋友对这里我说的浅拷贝
有些质疑,我也能理解大家所说的。下面数组为例:
// 数组元素为简单数据类型非引用类型 const arr = [1, 2, 3, 4]; const newArr = [...arr];
// 数组元素为引用类型 const person01 = {name: 'name01', age: 1}; const person02 = {name: 'name01', age: 2}; const person03 = {name: 'name03', age: 3}; const arr = [person01, person02, person03]; const newArr = [...arr]; console.log(newArr[0] === person01); // true
第二个 demo 就是我想表达的浅拷贝,若有不同意见欢迎讨论~
Hack #6 命名参数???
解构使得函数声明和函数的调用更加可读
// 我们尝尝使用的写法 const getStuffNotBad = (id, force, verbose) => { ...do stuff } // 当我们调用函数时, 明天再看,尼玛 150是啥,true是啥 getStuffNotBad(150, true, true) // 看完本文你啥都可以忘记, 希望够记住下面的就可以了 const getStuffAwesome = ({id, name, force, verbose}) => { ...do stuff } // 完美 getStuffAwesome({ id: 150, force: true, verbose: true })
Hack #7 Async/Await结合数组解构
数组解构非常赞!结合Promise.all
和解构
和await
会使代码变得更加的简洁
const [user, account] = await Promise.all([ fetch('/user'), fetch('/account') ])
彻底掌握 JS 异步处理 Promise 和 Async-Await
【开发环境推荐】Cloud Studio 是基于浏览器的集成式开发环境,支持绝大部分编程语言,包括 HTML5、PHP、Python、Java、Ruby、C/C++、.NET 小程序等等,无需下载安装程序,一键切换开发环境。 Cloud Studio提供了完整的 Linux 环境,并且支持自定义域名指向,动态计算资源调整,可以完成各种应用的开发编译与部署。
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK