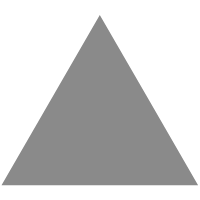
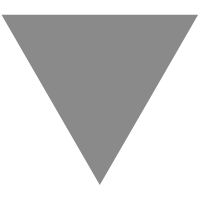
Integrating Neo4j with java – Rohit Khatana – Medium
source link: https://medium.com/@rohitkhatana/integrating-neo4j-with-java-8d602d9351b4
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Integrating Neo4j with java
First, What is Neo4j?
Neo4j is an ACID-compliant transactional database with native graph storage and processing database. And the most important which we like about Neo4j is that it promotes Relationship over Node i.e. Relationship is the first class citizen for Neo4j.
Installing Neo4j:
We will be using Neo4j 3.3 version as it is the stable one right now :)
- Traditional approach: download the package and install (link)
- Or Use docker image like this:
$ docker pull neo4j$ docker run --publish=7474:7474 --publish=7687:7687 \
--volume=$HOME/neo4j/data:/data neo4j:3.3
Let’s create a Neo Application
We will be using neo4j-ogm-core and neo4j-ogm-bolt-driver for interacting with Neo4j.
1.1 Defining Model Entity
Let’s first create a User model which we want to store in Neo4j.
Note: Id field is required for mapping/saving the object
package models;
import org.neo4j.ogm.annotation.GeneratedValue;
import org.neo4j.ogm.annotation.Id;
import org.neo4j.ogm.annotation.NodeEntity;
@NodeEntity
public class User { @Id
@GeneratedValue
Long id; private String name;
public User() {
} // setters & getters
}
@NodeEntity annotation is required to map the model class to OGM(Object graph model).
@Id is required by OGM
@GeneratedValue automatically generates the numeric id.
1.2 Connecting to Neo4j
Now let’s create a Neo4j Session which will handle the interaction with Neo4j:
package dao.neo4j;
import models.User;
import org.neo4j.ogm.config.Configuration;
import org.neo4j.ogm.session.Session;
import org.neo4j.ogm.session.SessionFactory;
public class Neo4jSessionFactory {
static Configuration configuration = new Configuration.Builder()
.uri("bolt://username:password@localhost")
.build();
static SessionFactory sessionFactory =
new SessionFactory(configuration, "models"); static Neo4jSessionFactory factory = new Neo4jSessionFactory();
public static Neo4jSessionFactory getInstance() {
return factory;
}
private Neo4jSessionFactory() {}
public Session getNeo4jSession() {
return sessionFactory.openSession();
}
}
Key points to consider before going ahead:
- Package name: it’s required for meta object scanning by neo-ogm, so give the package name, in which your classes are. In our example we are using package name: models
- And make the session object singleton, it’s upto to you if you want to make it Singleton, but it’s a better practice to follow.
1.3 Interacting with Neo4j
package dao.neo4j;class UserService {
private static final int DEPTH_LIST = 0
private static final int DEPTH_ENTITY = 1
protected Session session = Neo4jSessionFactory.getInstance().getNeo4jSession()
Iterable<User> findAll() {
return session.loadAll(getEntityType(), DEPTH_LIST)
}
Userfind(Long id) {
return session.load(User.class, id, DEPTH_ENTITY)
}
void delete(Long id) {
session.delete(session.load(User.class, id))
}
User createOrUpdate(User entity) {
session.save(entity, DEPTH_ENTITY)
return find(entity.id)
}
}
UserService is used to do CRUD operations on User. CreateOrUpdate will not update the record, if the record does not have the neoId, instead it will create the record, so before calling the method make sure you pass the db object or set the neoId explicitly on the object.
Conclusion:
I hope I explained everything clearly enough for you to understand. If you have any questions, feel free to ask. You can find me on twitter.
Make sure you click on green heart below and follow me for more stories about technology :)
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK