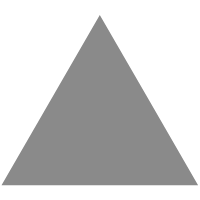
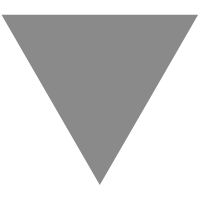
datetime - How to get current time in Python? - Stack Overflow
source link: https://stackoverflow.com/questions/415511/how-to-get-current-time-in-python?rq=1
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
How do I get the current time in Python?
The time
module
The time
module provides functions that tell us the time in "seconds since the epoch" as well as other utilities.
import time
Unix Epoch Time
This is the format you should get timestamps in for saving in databases. It is a simple floating-point number that can be converted to an integer. It is also good for arithmetic in seconds, as it represents the number of seconds since Jan 1, 1970, 00:00:00, and it is memory light relative to the other representations of time we'll be looking at next:
>>> time.time()
1424233311.771502
This timestamp does not account for leap-seconds, so it's not linear - leap seconds are ignored. So while it is not equivalent to the international UTC standard, it is close, and therefore quite good for most cases of record-keeping.
This is not ideal for human scheduling, however. If you have a future event you wish to take place at a certain point in time, you'll want to store that time with a string that can be parsed into a datetime
object or a serialized datetime
object (these will be described later).
time.ctime
You can also represent the current time in the way preferred by your operating system (which means it can change when you change your system preferences, so don't rely on this to be standard across all systems, as I've seen others expect). This is typically user friendly, but doesn't typically result in strings one can sort chronologically:
>>> time.ctime()
'Tue Feb 17 23:21:56 2015'
You can hydrate timestamps into human readable form with ctime
as well:
>>> time.ctime(1424233311.771502)
'Tue Feb 17 23:21:51 2015'
This conversion is also not good for record-keeping (except in text that will only be parsed by humans - and with improved Optical Character Recognition and Artificial Intelligence, I think the number of these cases will diminish).
datetime
module
The datetime
module is also quite useful here:
>>> import datetime
datetime.datetime.now
The datetime.now
is a class method that returns the current time. It uses the time.localtime
without the timezone info (if not given, otherwise see timezone aware below). It has a representation (which would allow you to recreate an equivalent object) echoed on the shell, but when printed (or coerced to a str
), it is in human readable (and nearly ISO) format, and the lexicographic sort is equivalent to the chronological sort:
>>> datetime.datetime.now()
datetime.datetime(2015, 2, 17, 23, 43, 49, 94252)
>>> print(datetime.datetime.now())
2015-02-17 23:43:51.782461
datetime's utcnow
You can get a datetime object in UTC time, a global standard, by doing this:
>>> datetime.datetime.utcnow()
datetime.datetime(2015, 2, 18, 4, 53, 28, 394163)
>>> print(datetime.datetime.utcnow())
2015-02-18 04:53:31.783988
UTC is a time standard that is nearly equivalent to the GMT timezone. (While GMT and UTC do not change for Daylight Savings Time, their users may switch to other timezones, like British Summer Time, during the Summer.)
datetime timezone aware
However, none of the datetime objects we've created so far can be easily converted to various timezones. We can solve that problem with the pytz
module:
>>> import pytz
>>> then = datetime.datetime.now(pytz.utc)
>>> then
datetime.datetime(2015, 2, 18, 4, 55, 58, 753949, tzinfo=<UTC>)
Equivalently, in Python 3 we have the timezone
class with a utc timezone
instance attached, which also makes the object timezone aware (but to convert to another timezone without the handy pytz
module is left as an exercise to the reader):
>>> datetime.datetime.now(datetime.timezone.utc)
datetime.datetime(2015, 2, 18, 22, 31, 56, 564191, tzinfo=datetime.timezone.utc)
And we see we can easily convert to timezones from the original UTC object.
>>> print(then)
2015-02-18 04:55:58.753949+00:00
>>> print(then.astimezone(pytz.timezone('US/Eastern')))
2015-02-17 23:55:58.753949-05:00
You can also make a naive datetime object aware with the pytz
timezone localize
method, or by replacing the tzinfo attribute (with replace
, this is done blindly), but these are more last resorts than best practices:
>>> pytz.utc.localize(datetime.datetime.utcnow())
datetime.datetime(2015, 2, 18, 6, 6, 29, 32285, tzinfo=<UTC>)
>>> datetime.datetime.utcnow().replace(tzinfo=pytz.utc)
datetime.datetime(2015, 2, 18, 6, 9, 30, 728550, tzinfo=<UTC>)
The pytz
module allows us to make our datetime
objects timezone aware and convert the times to the hundreds of timezones available in the pytz
module.
One could ostensibly serialize this object for UTC time and store that in a database, but it would require far more memory and be more prone to error than simply storing the Unix Epoch time, which I demonstrated first.
The other ways of viewing times are much more error-prone, especially when dealing with data that may come from different time zones. You want there to be no confusion as to which timezone a string or serialized datetime object was intended for.
If you're displaying the time with Python for the user, ctime
works nicely, not in a table (it doesn't typically sort well), but perhaps in a clock. However, I personally recommend, when dealing with time in Python, either using Unix time, or a timezone aware UTC datetime
object.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK