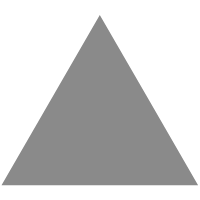
57
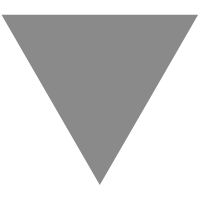
〔总结〕H5常见效果整理汇总资料
source link: https://segmentfault.com/a/1190000012622265?amp%3Butm_medium=referral
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
1.Canvas 实现圆形进度条并显示数字百分比
实现效果
1.首先创建html代码
<canvas id="canvas" width="500" height="500" style="background:#000;"></canvas>
2.创建canvas环境
var canvas = document.getElementById('canvas'), //获取canvas元素 context = canvas.getContext('2d'), //获取画图环境,指明为2d centerX = canvas.width/2, //Canvas中心点x轴坐标 centerY = canvas.height/2, //Canvas中心点y轴坐标 rad = Math.PI*2/100, //将360度分成100份,那么每一份就是rad度 speed = 0.1; //加载的快慢就靠它了
3.绘制5像素宽的运动外圈
//绘制5像素宽的运动外圈 function blueCircle(n){ context.save(); context.strokeStyle = "#fff"; //设置描边样式 context.lineWidth = 5; //设置线宽 context.beginPath(); //路径开始 context.arc(centerX, centerY, 100 , -Math.PI/2, -Math.PI/2 +n*rad, false); //用于绘制圆弧context.arc(x坐标,y坐标,半径,起始角度,终止角度,顺时针/逆时针) context.stroke(); //绘制 context.closePath(); //路径结束 context.restore(); }
4.绘制红色运动圈
function whiteCircle(){ context.save(); context.beginPath(); context.lineWidth = 2; //设置线宽 context.strokeStyle = "red"; context.arc(centerX, centerY, 100 , 0, Math.PI*2, false); context.stroke(); context.closePath(); context.restore(); }
5.百分比文字绘制
function text(n){ context.save(); //save和restore可以保证样式属性只运用于该段canvas元素 context.strokeStyle = "#fff"; //设置描边样式 context.font = "40px Arial"; //设置字体大小和字体 //绘制字体,并且指定位置 context.strokeText(n.toFixed(0)+"%", centerX-25, centerY+10); context.stroke(); //执行绘制 context.restore(); }
6.让它运动起来
(function drawFrame(){ window.requestAnimationFrame(drawFrame); context.clearRect(0, 0, canvas.width, canvas.height); whiteCircle(); text(speed); blueCircle(speed); if(speed > 100) speed = 0; speed += 0.1; }());
完整代码
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>HTML5 Canvas 圆形进度条并显示数字百分比</title> <style> *{margin:0;padding:0;} body{text-align:center;background-color:#000;} </style> </head> <body> <canvas id="canvas" width="500" height="500" style="background:#000;"></canvas> <script> window.onload = function(){ var canvas = document.getElementById('canvas'), //获取canvas元素 context = canvas.getContext('2d'), //获取画图环境,指明为2d centerX = canvas.width/2, //Canvas中心点x轴坐标 centerY = canvas.height/2, //Canvas中心点y轴坐标 rad = Math.PI*2/100, //将360度分成100份,那么每一份就是rad度 speed = 0.1; //加载的快慢就靠它了 //绘制5像素宽的运动外圈 function blueCircle(n){ context.save(); context.strokeStyle = "#fff"; //设置描边样式 context.lineWidth = 5; //设置线宽 context.beginPath(); //路径开始 context.arc(centerX, centerY, 100 , -Math.PI/2, -Math.PI/2 +n*rad, false); //用于绘制圆弧context.arc(x坐标,y坐标,半径,起始角度,终止角度,顺时针/逆时针) context.stroke(); //绘制 context.closePath(); //路径结束 context.restore(); } //绘制红色运动圈 function whiteCircle(){ context.save(); context.beginPath(); context.lineWidth = 2; //设置线宽 context.strokeStyle = "red"; context.arc(centerX, centerY, 100 , 0, Math.PI*2, false); context.stroke(); context.closePath(); context.restore(); } //百分比文字绘制 function text(n){ context.save(); //save和restore可以保证样式属性只运用于该段canvas元素 context.strokeStyle = "#fff"; //设置描边样式 context.font = "40px Arial"; //设置字体大小和字体 //绘制字体,并且指定位置 context.strokeText(n.toFixed(0)+"%", centerX-25, centerY+10); context.stroke(); //执行绘制 context.restore(); } //动画循环 (function drawFrame(){ window.requestAnimationFrame(drawFrame); context.clearRect(0, 0, canvas.width, canvas.height); whiteCircle(); text(speed); blueCircle(speed); if(speed > 100) speed = 0; speed += 0.1; }()); } </script> </body> </html>
2.H5实现树叶飘落
实现如图所示的东西效果(落叶下落):
html代码:
<!DOCTYPE html> <html> <head> <title>HTML5树叶飘落动画</title> <meta charset="utf-8"> <meta name="viewport" content="width=500px, initial-scale=0.64"> <link rel="stylesheet" href="leaves.css" type="text/css"> <script src="leaves.js" type="text/javascript"></script> </head> <body> <div id="container"> <div id="leafContainer"></div> <div id="message"> <em>这是基于webkit的落叶动画</em> </div> </div> </body> </html>
css代码:
body{ background-color: #4E4226; } #container { position: relative; height: 700px; width: 500px; margin: 10px auto; overflow: hidden; border: 4px solid #5C090A; background: #4E4226 url('images/backgroundLeaves.jpg') no-repeat top left; } #leafContainer { position: absolute; width: 100%; height: 100%; } #message{ position: absolute; top: 160px; width: 100%; height: 300px; background:transparent url('images/textBackground.png') repeat-x center; color: #5C090A; font-size: 220%; font-family: 'Georgia'; text-align: center; padding: 20px 10px; -webkit-box-sizing: border-box; -webkit-background-size: 100% 100%; z-index: 1; } em { font-weight: bold; font-style: normal; } #leafContainer > div { position: absolute; width: 100px; height: 100px; -webkit-animation-iteration-count: infinite; -webkit-animation-direction: normal; -webkit-animation-timing-function: linear; } #leafContainer > div > img { position: absolute; width: 100px; height: 100px; -webkit-animation-iteration-count: infinite; -webkit-animation-direction: alternate; -webkit-animation-timing-function: ease-in-out; -webkit-transform-origin: 50% -100%; } @-webkit-keyframes fade{ 0% { opacity: 1; } 95% { opacity: 1; } 100% { opacity: 0; } } @-webkit-keyframes drop{ 0% { -webkit-transform: translate(0px, -50px); } 100% { -webkit-transform: translate(0px, 650px); } } @-webkit-keyframes clockwiseSpin{ 0% { -webkit-transform: rotate(-50deg); } 100% { -webkit-transform: rotate(50deg); } } @-webkit-keyframes counterclockwiseSpinAndFlip { 0% { -webkit-transform: scale(-1, 1) rotate(50deg); } 100% { -webkit-transform: scale(-1, 1) rotate(-50deg); } }
js代码:
const NUMBER_OF_LEAVES = 30; function init(){ var container = document.getElementById('leafContainer'); for (var i = 0; i < NUMBER_OF_LEAVES; i++) { container.appendChild(createALeaf()); } } function randomInteger(low, high){ return low + Math.floor(Math.random() * (high - low)); } function randomFloat(low, high){ return low + Math.random() * (high - low); } function pixelValue(value){ return value + 'px'; } function durationValue(value){ return value + 's'; } function createALeaf(){ var leafDiv = document.createElement('div'); leafDiv.style.top = "-100px"; leafDiv.style.left = pixelValue(randomInteger(0, 500)); leafDiv.style.webkitAnimationName = 'fade, drop'; var fadeAndDropDuration = durationValue(randomFloat(5, 11)); leafDiv.style.webkitAnimationDuration = fadeAndDropDuration + ', ' + fadeAndDropDuration; var leafDelay = durationValue(randomFloat(0, 5)); leafDiv.style.webkitAnimationDelay = leafDelay + ', ' + leafDelay; var image = document.createElement('img'); image.src = 'images/realLeaf' + randomInteger(1, 5) + '.png'; var spinAnimationName = (Math.random() < 0.5) ? 'clockwiseSpin' : 'counterclockwiseSpinAndFlip'; image.style.webkitAnimationName = spinAnimationName; var spinDuration = durationValue(randomFloat(4, 8)); image.style.webkitAnimationDuration = spinDuration; leafDiv.appendChild(image); return leafDiv; } window.addEventListener('load', init, false);
转载地址:http://www.html5tricks.com/css3-fall-leaves.html
3.canvas处理连续帧图片
html5 canvas处理连续帧图片,下面的代码基于IE8以上
<!DOCTYPE html> <html> <head> <meta charset="utf-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0, minimum-scale=1.0, maximum-scale=1.0, user-scalable=no"/> <title>Canvas Demo</title> <script> var canvas = null;//初始化参数 var img = null; var ctx = null; var imageReady = false; window.onload = function() { var canvas = document.getElementById("animation_canvas"); canvas.width = canvas.parentNode.clientWidth; canvas.height = canvas.parentNode.clientHeight; if (!canvas.getContext) { console.log("Canvas not supported. Please install a HTML5 compatible browser."); return; } // get 2D context of canvas and draw rectangel ctx = canvas.getContext("2d"); ctx.fillStyle="black"; ctx.fillRect(0, 0, canvas.width, canvas.height); console.log(canvas.height); img = document.createElement('img'); img.src = "images/ab0.png"; img.onload = loaded(); } //保证只有图像加载后才开始循环动画 function loaded() { imageReady = true; setTimeout( update, 1000/3);//添加3帧每秒间隔计时器 } function redraw() { ctx.fillStyle="black"; ctx.fillRect(0, 0, 460, 460); ctx.drawImage(img, 0, 0, 232, 180); } //为了让图片以规定的速度动画,我们必须追踪已经经过的时间,然后根据分配给每帧的时间播放帧。基本步骤是: //1、按每秒几帧设置动画速度(msPerFrame)。 //2、当你循环游戏时,计算一下自最后一帧以后已经经过了多少时间(delta)。 //3、如果已经经过的时间足够把动画帧播完,那么播放这一帧并设置累积delta为0。 //4、如果已经经过的时间不够,那么记住(累积)delta时间(acDelta)。 var frame = 0; var lastUpdateTime = 0; var acDelta = 0; var msPerFrame = 200; function update() { requestAnimFrame(update); var delta = Date.now() - lastUpdateTime; //console.log(Date.now(),lastUpdateTime); if (acDelta > msPerFrame){ acDelta = 0; redraw(); img.src='images/ab'+frame+'.png'; frame++; if(frame >= 3) frame = 0; //当绘制后且帧推进完,计时器就会重置。 }else{ acDelta += delta; } lastUpdateTime = Date.now(); } //requestAnimFrame的作用基本上就是setTimeout,但浏览器知道你正在渲染帧,所以它可以优化绘制循环,以及如何与剩下的页面回流。 //在某些情况下,setTimeout比requestAnimFrame更好用,特别是对于手机。 //以下是在不同的浏览器上调用requestAnimFrame的情况也不同,标准的检测方法如下: window.requestAnimFrame = (function(){ return window.requestAnimationFrame || window.webkitRequestAnimationFrame || window.mozRequestAnimationFrame || window.oRequestAnimationFrame || window.msRequestAnimationFrame || function( callback ){ window.setTimeout(callback, 1000 / 3); //如果requestAnimFrame支持不可用,还是可以用回内置的setTimeout。 }; })(); </script> </head> <body style="position:absolute;margin:0;padding:0;width:100%;height:100%;"> <canvas id="animation_canvas"></canvas> </body> </html>
后续逐渐添加
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK