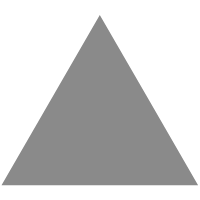
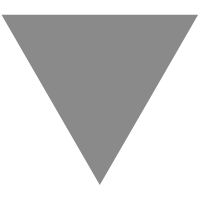
An Intro to Javascript Proxy Objects – Camp Vanilla
source link: https://blog.campvanilla.com/advanced-guide-javascript-proxy-objects-introduction-301c0fce9432?gi=476e36c6da80
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
An Intro to Javascript Proxy Objects
Change the way you interact with Objects
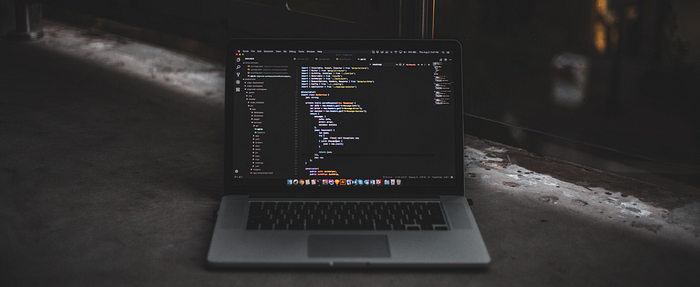
Proxies are Middleware for Javascript Objects
… or at least that’s sort of the tl;dr version for it.
Proxies were introduced in ES6 to allow you to provide custom functionality to basic operations that can be performed on an Object
. For example, get
is a basic Object
operation.
const obj = {
val: 10
};console.log(obj.val);
Here, the console.log()
statement performs a get
operation on the object obj
to get the value of the key val
.
Another basic Object operation is set
.
const obj = {
val: 10
};obj.val2 = 20;
Here, a set
operation is performed to set a new key to the object obj
.
How do I create a Proxy?
const proxiedObject = new Proxy(initialObj, handler);
Calling the Proxy constructor, new Proxy()
, will return an object that has the values contained in initialObj
but whose basic operations like get
and set
, now have some custom logic specified by the handler
object.
Let’s take an example to understand this,
Now, if we had not made a Proxy Object, calling console.log(proxiedObj.name)
on Line 14 would’ve logged “Foo Bar” to the console.
But now that we’ve made a Proxy, the get
operation has some custom logic that we have defined on Line 3.
Calling console.log(proxiedObj.name)
will now actually log “A value has been accessed” to the console!
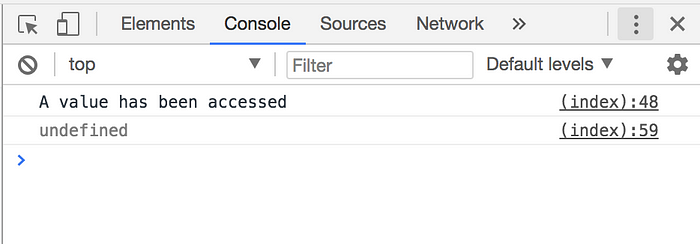
To the careful eye, you’d notice that there are actually two logs in the console. “A value has been accessed” and undefined. Why? 🤔
The default implementation of the get
operator is to return the value stored in that key in the Object. Since we overrode it to just log a statement, the value is never returned, and hence the console.log()
statement on line14 logs undefined
.
Let’s fix that!
The get
operator takes two parameters — the object itself and the property being accessed.
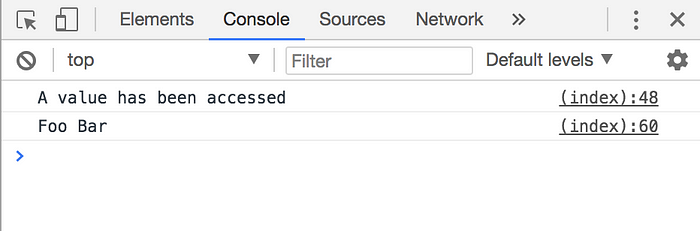
That’s better! 😄
This custom override that we provided for get
is called a trap
(loosely based on the concept of operating system traps). The handler object is basically an object with a set of traps that would trigger whenever an object property is being accessed.
Let’s add a trap for set
as well. We’ll do the same thing — any time a value is set, we’ll log the property being modified, as well as the value being set for that key.
The set
operator takes three arguments — the object, the property being accessed and the value being set for that property.
Here, the access being made at Line 18 will trigger the function defined at Line 6, which will log the property being accessed and the value being set.
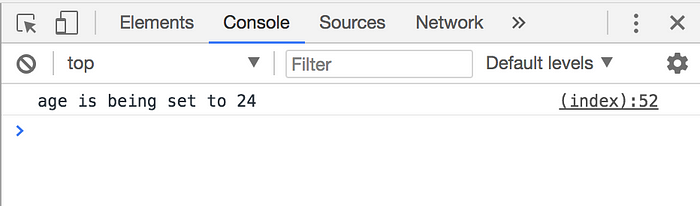
A Real-world Example
Say we have an object that defines a person
const person = {
id: 1,
name: 'Foo Bar'
};
What if we want to make the id
property of this object a private property. No one should be able to access this property via person.id
, and if someone does, we need to throw an error. How would we do this?
Proxies to the rescue! 🎉👩🚒
All we need to do is create a Proxy of this object, and override the get
operator to prevent us from accessing the id
property!
Here, in the trap we’ve created for get
, we check if the property being accessed is the id
property, and if so, we throw an error. Otherwise, we return the value as usual.
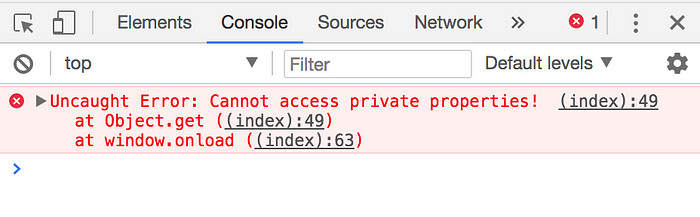
Another neat use case for this is validations. By setting a set
trap, we can add custom validation before we set the value. If the value does not conform to the validation, we can throw an error!
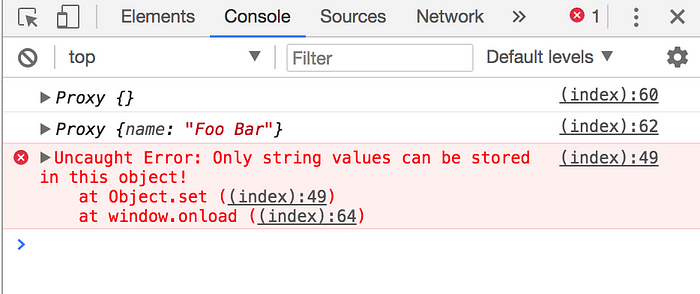
In the above examples, we’ve seen the get
and the set
traps. There are actually a lot more traps that can be set. You can find the entire list here.
Proxy Objects only just came into my radar after going through this article about them, and I can already see the usefulness of them in the code I write everyday!
If you’ve every used Proxies before, either in a project or at work, I would love to hear about it! 😄
~Fin~
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK