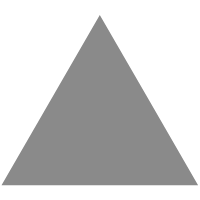
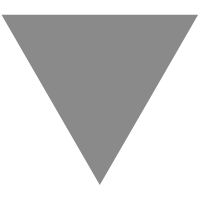
Getting started with Kotlin on Android – Sourcerer Blog
source link: https://blog.sourcerer.io/getting-started-with-kotlin-on-android-6242a5f6fd57?gi=a5d38edc32ee
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Getting started with Kotlin on Android
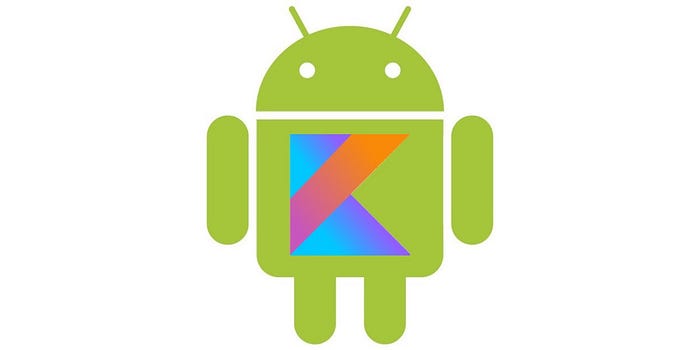
[Today’s random Sourcerer profile: https://sourcerer.io/wanghuaili]
Since Swift 1.0 hit the iOS development world toward the end of 2014, modern and expressive programming languages have become more and more popular. Android developers, however, have had a bit of a wait, but the time has come. Welcome, Kotlin.
Kotlin is new, fun, concise, developer-friendly, and easy to adopt. It is developed by JetBrains and is being thoroughly embraced by Google, via a strong partnership. Google’s support has surely had a positive effect on the ascension of Kotlin, as it is now finding its way into the codebase of Android apps such as Pinterest, Lyft, Netflix, Slack, and many more. Kotlin is a legitimate, forward-thinking programming language that offers a fantastic alternative to old, familiar Java.
Kotlin in Android Studio
With the release of Android Studio 3.0, Kotlin is pre-bundled and one click away. Let’s get started on a project using Android Studio 3.0.
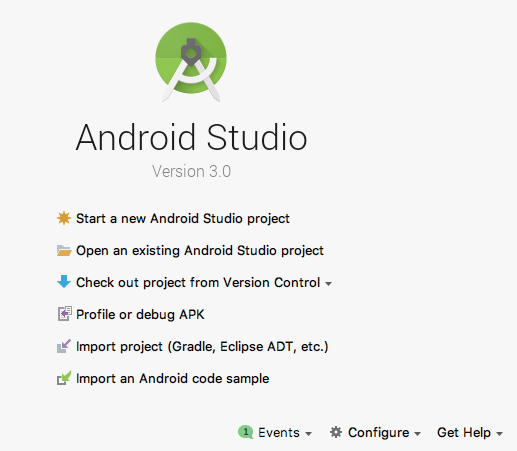
First, we’ll be starting a new Android Studio project at the top of the selection list. As in the image below, simply check the Include Kotlin support box. Yup, that’s it.
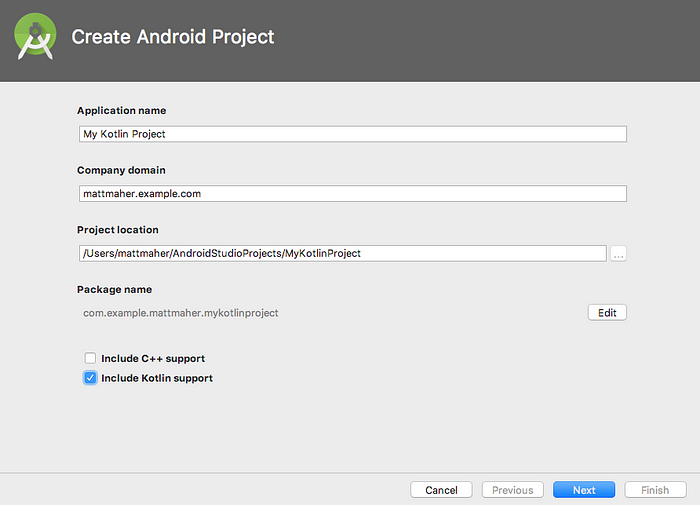
Then, continue with the project creation process as normal. With Android Studio 3.0, we’re all set.
In the next image, we can see that the new project is very familiar, but the syntax of MainActivity is a little different — more on that in a bit.
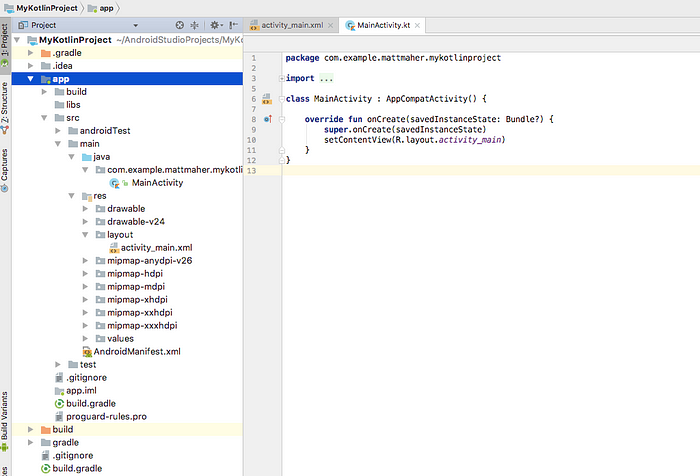
Prior to Android Studio 3.0, we need to install the Kotlin plugin, which is still pretty quick and easy.
For earlier versions of Android Studio, the Include Kotlin support box won’t appear, so we’ll create the new project with standard Java support.
Then, use the following path to install the Kotlin plugin: File / Settings / Plugins / Install JetBrains Plugin. Search for Kotlin, install it, and restart Android Studio. Next, as in the below image, go to Code, and select Convert Java File to Kotlin File. This will convert the file and code from Java to Kotlin.
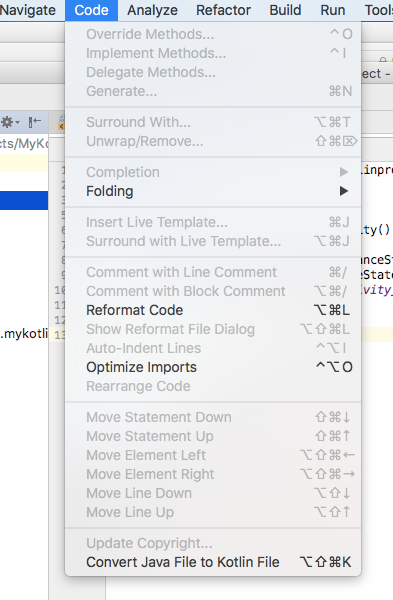
After converting, type a bit into the file, and Android Studio will show a prompt to configure for Kotlin. Tap Configure and Sync the project. The following images show the configuration process.
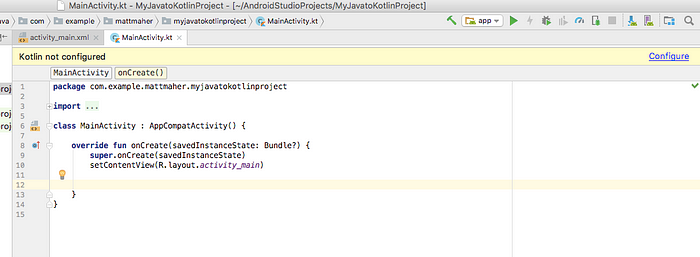
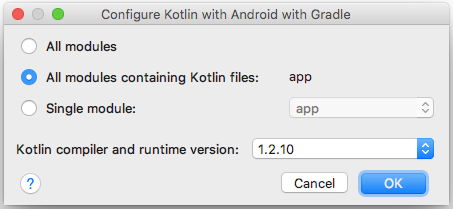
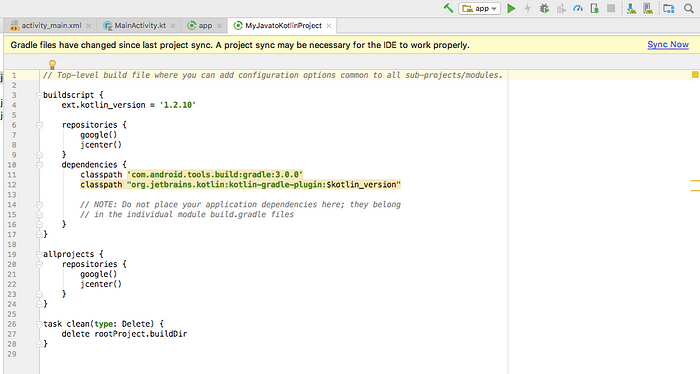
As we’ve discussed, an Android Studio project that supports Kotlin will still support Java — very key point. Notice MainActivity’s .kt file extension. That’s Kotlin! Now, for fun, proceed to add a new file in the Java directory. As we can see, options for both Java and Kotlin are at the top of the selection list.
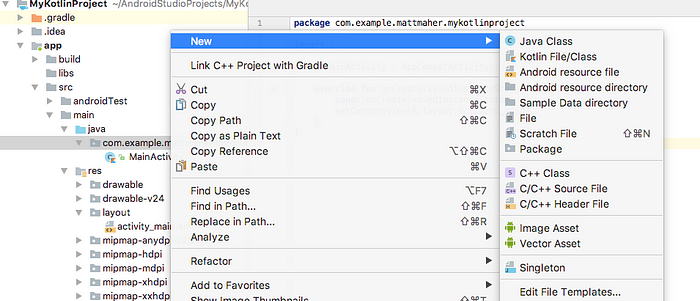
Now, for some Kotlin
Kotlin is a statically-typed programming language. Simply, this means that variables, as opposed to values are typed. It is fully interoperable with Java, as we’ve covered, which makes introducing it into an Android project a breeze. Code, in Kotlin, reads very well, and it is generally more concise — far less boilerplate code — really, less code. Kotlin is also type-safe and null-safe. The NullPointerException that is such a lovely part of Java programming is a thing of the past, aside from a few possible situations.
A good first step for getting a feel for Kotlin and its syntax is to do a bit of comparison with the familiar Java.
A look at Kotlin with Examples
If going through the process of converting a Java file to Kotlin, it’s interesting to watch the onCreate() method change.
In Java:
@Overrideprotected void onCreate(Bundle savedInstanceState) {super.onCreate(savedInstanceState);setContentView(R.layout.activity_main);}
In Kotlin:
override fun onCreate(savedInstanceState: Bundle?) {super.onCreate(savedInstanceState)setContentView(R.layout.activity_main)}
Surely, it’s familiar, but it is also a little bit more concise.
Java’s static is conveyed as val in Kotlin, and a variable would use var. Let’s mess with some stings in Kotlin.
var sport = “Football”sport = “Baseball”println(sport) // Baseballval game = “Chess”println(game) // Chess
Now, let’s do the same string manipulation and printing in Java.
String sport = “Football”;sport = “Baseball”;System.out.println(sport); // Baseballfinal String game = “Chess”;System.out.println(game); // Chess
As an aside, copying the above code in Java and pasting into a Kotlin file will trigger a generous offer from Android Studio to convert the code to Kotlin — free of charge.
Among the things to notice in the difference between the Kotlin and Java code is Kotlin’s ability to understand that a variable is a string without having to explicitly state it in the declaration.
Next, we’ll look at a method that takes an argument and returns a value in Java.
int calcPoints(int touchdownCount) {int pointsTotal = touchdownCount * 7;return pointsTotal;}
Now, here is the same method in Kotlin:
fun calcPoints(touchdownCount: Int): Int {val pointsTotal = touchdownCount * 7return pointsTotal}
The Kotlin code almost starts to look a little like Swift! The well-stated fun at the start of the method, the argument name and type, and it is all followed by the return type.
Here, the method takes the number of touchdowns scored by a team and returns the number of points earned — assuming an extra point was scored.
Below, we’ll wire the calcPoints() and messWithStrings() methods into the app. Additionally, let’s feed another method that will print a string that is fed by the methods and a switch.
First, here is the Java version of the code:
public class MainActivity extends AppCompatActivity {
@Overrideprotected void onCreate(Bundle savedInstanceState) {super.onCreate(savedInstanceState);setContentView(R.layout.activity_main);int points = calcPoints(4);String offenseType;switch (points) {case 14:case 13:case 12:case 11:case 10:case 9:case 8:offenseType = “Pretty good”;break;case 7:case 6:case 5:case 4:case 3:case 2:case 1:offenseType = “Not bad”;break;case 0:offenseType = “Not very productive”;break;default:offenseType = “Very good”;}
printOffenseType(offenseType);messWithStrings();}
int calcPoints(int touchdownCount) {int pointsTotal = touchdownCount * 7;return pointsTotal;}
void printOffenseType(String offenseString) {System.out.println(offenseString);}
void messWithStrings() {String sport = “Football”;sport = “Baseball”;System.out.println(sport);final String game = “Chess”;System.out.println(game);}}
As always, it’s familiar. The football team has a pretty good offense. Let’s go right to the Kotlin.
class MainActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {super.onCreate(savedInstanceState)setContentView(R.layout.activity_main)var points = calcPoints(4)var offenseType = when (points) {in 8..14 -> “Pretty good”in 1..7 -> “Not bad”0 -> “Not very productive”else -> “Very good”}printOffenseType(offenseType)messWithStrings()}
fun calcPoints(touchdownCount: Int): Int {val pointsTotal = touchdownCount * 7return pointsTotal}
fun printOffenseType(offenseString: String) {println(offenseString)}
fun messWithStrings() {var sport = “Football”sport = “Baseball”println(sport)val game = “Chess”println(game)}}
Now we’re talking! Having a look at onCreate(), we see some of the syntax magic of Kotlin. We’re able to avoid the manual labor that was required in the Java code. offenseType is determined quickly, cleanly, and clearly.
Going further
Kotlin Koans is an awesome resource for learning the Kotlin language. Additionally, here is Kotlin’s own online IDE that packed full of awesome lessons that will get developers rolling quickly.
Seeing Google, major apps, and other developers adopt Kotlin with such vigor greatly enhances its stature in the future of Android development. After all, look at what’s happened with Swift in the iOS world. It’s a blast working with these wonderful new languages, watching them grow, and seeing their adoption spread. As Mr. Bob Dylan said a few times, “Times, they are a changin’.”
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK