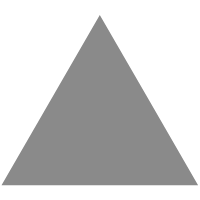
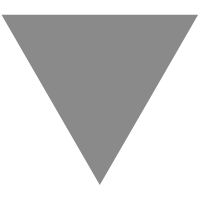
Golang深入浅出之-Goroutine泄漏检测与避免:pprof与debug包
source link: https://blog.51cto.com/u_16701217/10676009
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
在Go语言中,goroutine是轻量级线程,但如果管理不当,可能会导致goroutine泄漏,进而消耗大量系统资源。本文将介绍如何使用pprof
和debug
包来检测和避免goroutine泄漏,以及常见问题和解决方案。

Goroutine泄漏常见问题
- 忘记关闭通道(channel) :当goroutine持续监听一个未关闭的通道时,它将永久运行。
- 无限循环:在goroutine中,如果存在无条件的无限循环,该goroutine将永远不会退出。
- 依赖外部条件:goroutine可能依赖于某个外部条件来决定何时退出,如果这个条件永远不满足,goroutine也会泄漏。
使用pprof检测泄漏
net/http/pprof
包提供了一个HTTP服务器,用于收集和分析性能数据,包括goroutine信息。
import _ "net/http/pprof" // 引入pprof
func main() {
go func() {
http.ListenAndServe(":6060", nil) // 启动pprof服务器
}()
// ... 你的业务代码 ...
}
运行程序后,访问http://localhost:6060/debug/pprof/goroutine?debug=2
,可以看到详细的goroutine堆栈信息。
使用debug
包避免泄漏
runtime/debug
包提供了一些实用函数,可以帮助我们检查和清理goroutine。
SetGCPercent
通过设置垃圾回收的百分比,我们可以控制何时进行垃圾回收,从而间接影响goroutine的行为。
import "runtime/debug"
func main() {
debug.SetGCPercent(50) // 设置为50%
// ... 你的业务代码 ...
}
FreeOSMemory
FreeOSMemory
函数可以强制进行垃圾回收并释放操作系统内存,有助于发现因泄漏导致的内存增长。
import "runtime/debug"
func main() {
// ... 你的业务代码 ...
debug.FreeOSMemory() // 强制释放内存
}
避免泄漏的实践策略
- 使用
sync.WaitGroup
:确保在所有goroutine完成后,主程序能正确等待它们结束。 - 避免无限循环:在循环中添加退出条件,或者使用
context.Context
来取消goroutine。 - 关闭通道:当不再需要goroutine时,及时关闭通道以通知goroutine退出。
Goroutine泄漏是一个严重问题,但通过引入pprof
和debug
包,我们可以有效地检测和避免它。理解这些工具的使用,结合良好的编程实践,可以确保Go程序的健康运行。在编写并发代码时,时刻注意goroutine的生命周期管理,是避免泄漏的关键。
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK