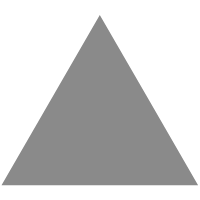
6
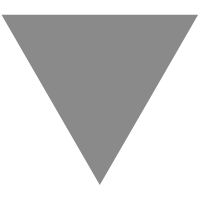
Python文件与目录操作:面试中的高频考点
source link: https://blog.51cto.com/u_16701217/10510710
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Python文件与目录操作:面试中的高频考点
精选 原创Python文件与目录操作是编程面试中不可或缺的一部分,涵盖文件的读写、目录的遍历、权限管理等核心知识点。本文将深入浅出地剖析相关面试题,揭示常见问题与易错点,并提供实用的代码示例,助您在面试中游刃有余。
1. 文件基本操作
面试题:读取文件内容、写入文件、追加内容到文件。
易错点与避免策略:
- 忘记关闭文件:在完成文件操作后,务必使用
file.close()
方法关闭文件,或者使用with open()
语句自动关闭,以防止资源泄露。 - 忽视异常处理:在文件读写过程中,可能出现文件不存在、无权限访问等问题。应使用
try-except
结构捕获FileNotFoundError
、PermissionError
等异常。
代码示例:
# 读取文件内容
def read_file(filename):
try:
with open(filename, 'r') as file:
content = file.read()
return content
except FileNotFoundError:
print(f"File '{filename}' not found.")
return None
# 写入文件(覆盖原有内容)
def write_file(filename, content):
try:
with open(filename, 'w') as file:
file.write(content)
except PermissionError:
print(f"No permission to write to file '{filename}'.")
# 追加内容到文件
def append_to_file(filename, content):
try:
with open(filename, 'a') as file:
file.write(content)
except PermissionError:
print(f"No permission to append to file '{filename}'.")
content = read_file('input.txt')
write_file('output.txt', 'New content')
append_to_file('log.txt', '\nAdditional log entry')
2. 目录遍历与文件查找
面试题:递归遍历目录下的所有文件和子目录,以及按特定条件(如文件扩展名)筛选文件。
易错点与避免策略:
- 忽略特殊文件(如
.
和..
):在遍历目录时,应使用os.path.isfile()
、os.path.isdir()
等函数判断是否为有效文件或目录,避免处理.
和..
等特殊条目。 - 忽视异常处理:在遍历目录树时,可能遇到无权限访问的目录。应适当处理
PermissionError
异常,确保程序稳定运行。
代码示例:
import os
def list_files_recursively(directory, extension=None):
for root, dirs, files in os.walk(directory):
for file in files:
if extension is None or file.endswith(extension):
yield os.path.join(root, file)
for file_path in list_files_recursively('.', '.txt'):
print(file_path)
3. 文件与目录权限管理
面试题:检查文件或目录的权限,以及修改文件或目录的所有者、组和其他权限。
易错点与避免策略:
- 混淆权限位与权限字符串:理解并正确使用
os.stat().st_mode
返回的权限位(如0o755
)与chmod
命令接受的权限字符串(如'rw-r-xr--'
)之间的转换。 - 忘记导入
pwd
和grp
模块:在处理用户和组信息时,需要导入这两个模块以获取用户名和组名。
代码示例:
import os
import pwd
import grp
def get_permissions(path):
stats = os.stat(path)
mode = stats.st_mode & 0o777
return oct(mode)
def change_owner(path, user, group):
uid = pwd.getpwnam(user).pw_uid
gid = grp.getgrnam(group).gr_gid
os.chown(path, uid, gid)
permissions = get_permissions('/path/to/file')
print(permissions)
change_owner('/path/to/directory', 'new_user', 'new_group')
综上所述,掌握Python文件与目录操作的常见面试题、识别并规避易错点,辅以实战代码示例,将使您在面试中展现出扎实的文件系统管理能力。持续磨练这些技能,您将在编程求职道路上更进一步。
- 赞
- 收藏
- 评论
- 分享
- 举报
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK