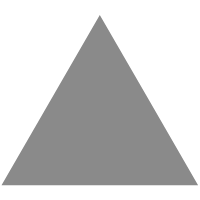
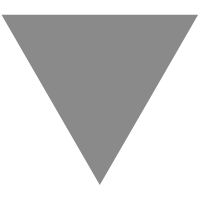
前端代码规范 - 编辑器&代码风格
source link: https://blog.51cto.com/react/10518894
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
前端代码规范 - 编辑器&代码风格
精选 原创本文是前端代码规范系列文章,将涵盖前端领域各方面规范整理,其他完整文章可前往主页查阅~
在如今快速发展的前端工程领域,团队成员较多时导致编码风格百花齐放,如何保持代码的一致性和可读性成为了开发过程中不可忽视的挑战。
为了应对这种复杂性,现在的工程中存在着越来越多的配置文件,这些文件和工具帮助我们自动化和标准化开发流程。比如有如下配置文件:
editorconfig
Prettier
ESLint
: Vue/Reactstylelint
ignore
:gitignore/eslintignoretsconfig.json
vscode
:settings.json
本文将介绍如何利用这些工具和配置文件,优化前端开发流程,实现代码风格的统一和提高开发效率。
需要注意的是这些配置建议基于个人的开发经验,但每个项目和团队都有其独特性。所有的配置建议都应当根据具体的项目需求和团队习惯来调整,以确保最佳适配。
.editorconfig
配置
.editorconfig
帮助开发者在不同的编辑器和IDE中保持一致的编码风格。它是一个简单的文件,用来定义项目的编码规范,如缩进大小、行尾风格等。这个文件应该放在项目的根目录中,各种编辑器和IDE都能识别这个文件。
示例配置:
# .editorconfig
root = true
[*]
charset = utf-8 # 字符编码 utf-8
indent_style = space # 输入的 tab 都用空格代替
indent_size = 2 # 一个 tab 用 2 个空格代替
end_of_line = lf # 换行符使用 unix 的换行符 \n
trim_trailing_whitespace = true # 去掉每行末尾的空格
insert_final_newline = true # 文件末尾都加一个空行
[*.md]
trim_trailing_whitespace = false # .md 文件不去掉每行末尾的空格
这个配置设定了大多数文件采用utf-8
编码,使用空格作为缩进字符,每个缩进级别2个空格,行尾风格为lf
,自动去除行尾空白,以及文件末尾自动添加新行。对于Markdown文件(*.md
),不去除行尾空白,因为在Markdown中,两个空格可以产生一个新的行。
Prettier
配置
Prettier
是一个流行的代码格式化工具,它支持许多语言和框架。通过Prettier
,可以确保所有开发人员按照同一套规则格式化代码,减少因格式问题造成的代码冲突。
安装Prettier:
npm install --save-dev --save-exact prettier
示例配置(.prettierrc.js
或prettier.config.js
):
/**
* Prettier 标准配置
*/
module.exports = {
// 在 ES5 中有效的结尾逗号(对象,数组等)
trailingComma: 'none',
// 不使用缩进符,而使用空格
useTabs: false,
// Tab 用两个空格代替
tabWidth: 2,
// 仅在语法可能出现错误的时候才会添加分号
semi: false,
// 使用单引号
singleQuote: true,
// 在 Vue 文件中缩进脚本和样式标签。
vueIndentScriptAndStyle: true,
// 一行最多 100 字符
printWidth: 100,
// 对象的 key 仅在必要时用引号
quoteProps: 'as-needed',
// JSX 不使用单引号,而使用双引号
jsxSingleQuote: false,
// 大括号内的首尾需要空格
bracketSpacing: true,
// JSX 标签的反尖括号需要换行
jsxBracketSameLine: false,
// 箭头函数,只有一个参数的时候,也需要括号
arrowParens: 'always',
// 每个文件格式化的范围是文件的全部内容
rangeStart: 0,
rangeEnd: Infinity,
// 不需要写文件开头的 @prettier
requirePragma: false,
// 不需要自动在文件开头插入 @prettier
insertPragma: false,
// 使用默认的折行标准
proseWrap: 'preserve',
// 根据显示样式决定 HTML 要不要折行
htmlWhitespaceSensitivity: 'css',
// 换行符使用 LF
endOfLine: 'lf'
}
这里配置了包括不使用缩进符、使用两个空格作为Tab、不添加分号、使用单引号、限制一行最多100个字符、根据需要使用引号等代码规范。
ESLint
配置
ESLint
是一个插件化的JavaScript/TypeScript代码检查工具,它不仅可以检查代码错误,还可以检查代码风格。通过ESLint
,可以更加细致地规范代码风格,并保证代码质量。
安装ESLint及相关插件:
npm install --save-dev eslint eslint-config-prettier eslint-plugin-prettier
Vue项目示例配置(.eslintrc.js
):
module.exports = {
root: true,
parserOptions: {
ecmaVersion: 6,
parser: "@typescript-eslint/parser",
sourceType: "module",
ecmaFeatures: {
// 支持装饰器
legacyDecorators: true
}
},
// 全局变量
globals: {
window: true,
document: true,
wx: true
},
// 兼容环境
env: {
browser: true
},
// 插件
extends: ['plugin:vue/essential', '@vue/standard', '@vue/typescript', 'standard-with-typescript'],
rules: {
// 缩进检查
indent: [
'error',
2,
{
SwitchCase: 1,
ignoredNodes: ['TemplateLiteral > *']
}
],
// 末尾不加分号,只有在有可能语法错误时才会加分号
semi: 0,
// 箭头函数需要有括号 (a) => {}
'arrow-parens': 0,
// 关闭不允许回调未定义的变量
'standard/no-callback-literal': 0,
// 关闭以避免 ts 报错
'dot-notation': 0,
// 关闭副作用的 new
'no-new': 0,
// 关闭每行最大长度小于 80
'max-len': 0,
// 关闭要求 require() 出现在顶层模块作用域中
'global-require': 0,
// 关闭关闭类方法中必须使用 this
'class-methods-use-this': 0,
// 关闭禁止对原生对象或只读的全局对象进行赋值
'no-global-assign': 0,
// 关闭禁止对关系运算符的左操作数使用否定操作符
'no-unsafe-negation': 0,
// 关闭禁止使用 console
'no-console': 0,
// 关闭禁止末尾空行
'eol-last': 0,
// 关闭强制在注释中 // 或 /* 使用一致的空格
'spaced-comment': 0,
// 关闭禁止对 function 的参数进行重新赋值
'no-param-reassign': 0,
// 关闭全等 === 校验
eqeqeq: 0,
// 关闭强制使用骆驼拼写法命名约定
camelcase: 0,
// 最后一个分号和右括号之间的空格
'declaration-block-semicolon-newline-after': 0,
// 在声明块中要求或禁止尾部分号
'declaration-block-trailing-semicolon': 0,
// promise async
'no-async-promise-executor': 0,
// 允许代码中存在制表符
'no-tabs': 0,
// 允许在Vue组件中修改props的值
'vue/no-mutating-props': 0,
// 允许在case语句中声明变量
'no-case-declarations': 0,
// 强制使用一致的换行符风格 (linebreak-style)
'linebreak-style': ['error', 'unix'],
// 禁止使用拖尾逗号
'comma-dangle': ['error', 'never'],
// 函数括号前面不加空格
'space-before-function-paren': ['error', 'never']
}
}
React项目示例配置(.eslintrc.js
):
module.exports = {
root: true,
extends: [require.resolve('@umijs/fabric/dist/eslint')],
globals: {
React: 'readable'
},
rules: {
// 末尾不加分号,只有在有可能语法错误时才会加分号
semi: 0,
'@typescript-eslint/semi': 0,
// 箭头函数需要有括号 (a) => {}
'arrow-parens': 0,
// 两个空格缩进, switch 语句中的 case 为 1 个空格
indent: [
'error',
2,
{
SwitchCase: 1
}
],
// 关闭不允许回调未定义的变量
'standard/no-callback-literal': 0,
// 关闭副作用的 new
'no-new': 'off',
// 关闭每行最大长度小于 80
'max-len': 0,
// 函数括号前面不加空格
'space-before-function-paren': ['error', 'never'],
// 关闭要求 require() 出现在顶层模块作用域中
'global-require': 0,
// 关闭关闭类方法中必须使用this
'class-methods-use-this': 0,
// 关闭禁止对原生对象或只读的全局对象进行赋值
'no-global-assign': 0,
// 关闭禁止对关系运算符的左操作数使用否定操作符
'no-unsafe-negation': 0,
// 关闭禁止使用 console
'no-console': 0,
// 关闭禁止末尾空行
'eol-last': 0,
// 关闭强制在注释中 // 或 /* 使用一致的空格
'spaced-comment': 0,
// 关闭禁止对 function 的参数进行重新赋值
'no-param-reassign': 0,
// 强制使用一致的换行符风格 (linebreak-style)
'linebreak-style': 0,
// 关闭全等 === 校验
eqeqeq: 0,
// 禁止使用拖尾逗号(即末尾不加逗号)
'comma-dangle': ['error', 'never'],
// 关闭强制使用骆驼拼写法命名约定
camelcase: 0,
//最后一个分号和右括号之间的空格
'declaration-block-semicolon-newline-after': 0,
//在声明块中要求或禁止尾部分号
'declaration-block-trailing-semicolon': 0,
//对程序状态没有影响的未使用表达式表示逻辑错误
'no-unused-expressions': 1,
// 允许存在未使用的表达式
'@typescript-eslint/no-unused-expressions': 0,
// 强制在类的成员之间有一个空行
'lines-between-class-members': 1,
// 允许存在嵌套的三元表达式
'no-nested-ternary': 0,
// 允许在TypeScript中存在变量重复声明
'@typescript-eslint/no-shadow': 0
}
}
stylelint
配置
stylelint
专注于样式文件,帮助开发者避免错误并保持样式代码的一致性。
module.exports = {
root: true,
extends: ['stylelint-config-standard', 'stylelint-config-sass-guidelines'],
rules: {
'color-hex-length': null,
'max-nesting-depth': null,
'selector-class-pattern': null,
'selector-pseudo-class-no-unknown': null,
'selector-no-qualifying-type': null,
'selector-max-compound-selectors': null,
'no-descending-specificity': null,
'property-no-vendor-prefix': null,
'value-no-vendor-prefix': null,
'scss/at-mixin-pattern': null,
'scss/at-import-partial-extension-blacklist': null,
'function-parentheses-newline-inside': null,
'declaration-property-value-blacklist': null,
'no-empty-source': null,
'order/properties-alphabetical-order': null,
'font-family-no-missing-generic-family-keyword': null,
'scss/at-import-partial-extension-blacklist': null,
'selector-max-id': null,
'selector-pseudo-element-no-unknown': [true, {
'ignorePseudoElements': ['v-deep']
}]
}
}
ignore
文件配置
.gitignore
和.eslintignore
等ignore文件,帮助我们定义哪些文件或目录可以被git或ESLint忽略,以避免不必要的文件跟踪或检查。
gitignore
.DS_Store
node_modules
/dist
# local env files
.env.local
.env.*.local
# Log files
npm-debug.log*
yarn-debug.log*
yarn-error.log*
# Editor directories and files
.idea
*.suo
*.ntvs*
*.njsproj
*.sln
*.sw?
eslintignore
node_modules
dist/
test/
lib/
es/
logs/
tsconfig.json
配置
对于使用TypeScript的项目,tsconfig.json
配置文件指定了编译选项和项目设置,是TypeScript项目的核心配置文件。
{
"compilerOptions": {
"target": "esnext",
"module": "esnext",
"strict": false,
"jsx": "preserve",
"importHelpers": true,
"moduleResolution": "node",
"experimentalDecorators": true,
"esModuleInterop": true,
"allowSyntheticDefaultImports": true,
"noImplicitAny": false,
"strictNullChecks": true,
"sourceMap": true,
"skipLibCheck": true,
"baseUrl": ".",
"types": [
"webpack-env"
],
"paths": {
"@/*": [
"src/*"
]
},
"lib": [
"esnext",
"dom",
"dom.iterable",
"scripthost"
]
},
"include": [
"src/**/*.ts",
"src/**/*.tsx",
"src/**/*.vue",
"tests/**/*.ts",
"tests/**/*.tsx"
],
"exclude": [
"node_modules"
]
}
vscode
配置
通过settings.json
,可以为VS Code编辑器定制一系列工作区级别的设置,包括但不限于编辑器行为、插件配置等。
{
"editor.tabSize": 2,
"editor.formatOnSave": false,
"eslint.alwaysShowStatus": true,
"eslint.validate": [
"javascript",
"javascriptreact",
"vue",
],
"tslint.autoFixOnSave": false,
"tslint.alwaysShowRuleFailuresAsWarnings": true,
"stylelint.autoFixOnSave": false,
"vetur.format.defaultFormatter.html": "prettier",
"vetur.format.defaultFormatterOptions": {
"prettier": {
"semi": false,
"singleQuote": true
}
},
"[typescript]": {
"editor.defaultFormatter": "esbenp.prettier-vscode"
},
"[html]": {
"editor.defaultFormatter": "vscode.html-language-features"
},
"[jsonc]": {
"editor.defaultFormatter": "esbenp.prettier-vscode"
},
"[json]": {
"editor.defaultFormatter": "esbenp.prettier-vscode"
},
"[vue]": {
"editor.defaultFormatter": "octref.vetur"
},
"[javascript]": {
"editor.defaultFormatter": "vscode.typescript-language-features"
},
"[javascriptreact]": {
"editor.defaultFormatter": "esbenp.prettier-vscode"
},
"workbench.commandPalette.experimental.suggestCommands": false,
"vue.inlayHints.optionsWrapper": false
}
在本文我们探讨了如何通过现代前端开发中的各种配置文件来标准化和优化开发流程。这些工具和文件包括.editorconfig
、Prettier
、ESLint
、stylelint
、忽略文件(如.gitignore
和.eslintignore
)以及tsconfig.json
和VS Code的settings.json
,为我们提供了实现代码一致性、可读性和自动化的强大支持。
虽然文中提供了基于实践的建议配置,但最终的实现应根据具体的项目需求和团队习惯进行灵活调整。 正确利用这些工具和配置可以显著提高前端开发的效率和质量,帮助团队维护一个清晰且高效的开发环境。
看完本文如果觉得有用,记得点个赞支持,收藏起来说不定哪天就用上啦~
专注前端开发,分享前端相关技术干货,公众号:南城大前端(ID: nanchengfe)
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK