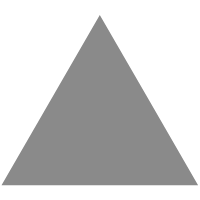
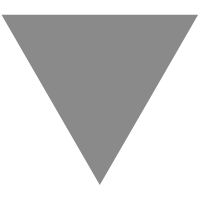
API with NestJS #148. Understanding the injection scopes
source link: https://wanago.io/2024/04/01/api-nestjs-injection-scopes/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.

- 1. API with NestJS #1. Controllers, routing and the module structure
- 2. API with NestJS #2. Setting up a PostgreSQL database with TypeORM
- 3. API with NestJS #3. Authenticating users with bcrypt, Passport, JWT, and cookies
- 4. API with NestJS #4. Error handling and data validation
- 5. API with NestJS #5. Serializing the response with interceptors
- 6. API with NestJS #6. Looking into dependency injection and modules
- 7. API with NestJS #7. Creating relationships with Postgres and TypeORM
- 8. API with NestJS #8. Writing unit tests
- 9. API with NestJS #9. Testing services and controllers with integration tests
- 10. API with NestJS #10. Uploading public files to Amazon S3
- 11. API with NestJS #11. Managing private files with Amazon S3
- 12. API with NestJS #12. Introduction to Elasticsearch
- 13. API with NestJS #13. Implementing refresh tokens using JWT
- 14. API with NestJS #14. Improving performance of our Postgres database with indexes
- 15. API with NestJS #15. Defining transactions with PostgreSQL and TypeORM
- 16. API with NestJS #16. Using the array data type with PostgreSQL and TypeORM
- 17. API with NestJS #17. Offset and keyset pagination with PostgreSQL and TypeORM
- 18. API with NestJS #18. Exploring the idea of microservices
- 19. API with NestJS #19. Using RabbitMQ to communicate with microservices
- 20. API with NestJS #20. Communicating with microservices using the gRPC framework
- 21. API with NestJS #21. An introduction to CQRS
- 22. API with NestJS #22. Storing JSON with PostgreSQL and TypeORM
- 23. API with NestJS #23. Implementing in-memory cache to increase the performance
- 24. API with NestJS #24. Cache with Redis. Running the app in a Node.js cluster
- 25. API with NestJS #25. Sending scheduled emails with cron and Nodemailer
- 26. API with NestJS #26. Real-time chat with WebSockets
- 27. API with NestJS #27. Introduction to GraphQL. Queries, mutations, and authentication
- 28. API with NestJS #28. Dealing in the N + 1 problem in GraphQL
- 29. API with NestJS #29. Real-time updates with GraphQL subscriptions
- 30. API with NestJS #30. Scalar types in GraphQL
- 31. API with NestJS #31. Two-factor authentication
- 32. API with NestJS #32. Introduction to Prisma with PostgreSQL
- 33. API with NestJS #33. Managing PostgreSQL relationships with Prisma
- 34. API with NestJS #34. Handling CPU-intensive tasks with queues
- 35. API with NestJS #35. Using server-side sessions instead of JSON Web Tokens
- 36. API with NestJS #36. Introduction to Stripe with React
- 37. API with NestJS #37. Using Stripe to save credit cards for future use
- 38. API with NestJS #38. Setting up recurring payments via subscriptions with Stripe
- 39. API with NestJS #39. Reacting to Stripe events with webhooks
- 40. API with NestJS #40. Confirming the email address
- 41. API with NestJS #41. Verifying phone numbers and sending SMS messages with Twilio
- 42. API with NestJS #42. Authenticating users with Google
- 43. API with NestJS #43. Introduction to MongoDB
- 44. API with NestJS #44. Implementing relationships with MongoDB
- 45. API with NestJS #45. Virtual properties with MongoDB and Mongoose
- 46. API with NestJS #46. Managing transactions with MongoDB and Mongoose
- 47. API with NestJS #47. Implementing pagination with MongoDB and Mongoose
- 48. API with NestJS #48. Definining indexes with MongoDB and Mongoose
- 49. API with NestJS #49. Updating with PUT and PATCH with MongoDB and Mongoose
- 50. API with NestJS #50. Introduction to logging with the built-in logger and TypeORM
- 51. API with NestJS #51. Health checks with Terminus and Datadog
- 52. API with NestJS #52. Generating documentation with Compodoc and JSDoc
- 53. API with NestJS #53. Implementing soft deletes with PostgreSQL and TypeORM
- 54. API with NestJS #54. Storing files inside a PostgreSQL database
- 55. API with NestJS #55. Uploading files to the server
- 56. API with NestJS #56. Authorization with roles and claims
- 57. API with NestJS #57. Composing classes with the mixin pattern
- 58. API with NestJS #58. Using ETag to implement cache and save bandwidth
- 59. API with NestJS #59. Introduction to a monorepo with Lerna and Yarn workspaces
- 60. API with NestJS #60. The OpenAPI specification and Swagger
- 61. API with NestJS #61. Dealing with circular dependencies
- 62. API with NestJS #62. Introduction to MikroORM with PostgreSQL
- 63. API with NestJS #63. Relationships with PostgreSQL and MikroORM
- 64. API with NestJS #64. Transactions with PostgreSQL and MikroORM
- 65. API with NestJS #65. Implementing soft deletes using MikroORM and filters
- 66. API with NestJS #66. Improving PostgreSQL performance with indexes using MikroORM
- 67. API with NestJS #67. Migrating to TypeORM 0.3
- 68. API with NestJS #68. Interacting with the application through REPL
- 69. API with NestJS #69. Database migrations with TypeORM
- 70. API with NestJS #70. Defining dynamic modules
- 71. API with NestJS #71. Introduction to feature flags
- 72. API with NestJS #72. Working with PostgreSQL using raw SQL queries
- 73. API with NestJS #73. One-to-one relationships with raw SQL queries
- 74. API with NestJS #74. Designing many-to-one relationships using raw SQL queries
- 75. API with NestJS #75. Many-to-many relationships using raw SQL queries
- 76. API with NestJS #76. Working with transactions using raw SQL queries
- 77. API with NestJS #77. Offset and keyset pagination with raw SQL queries
- 78. API with NestJS #78. Generating statistics using aggregate functions in raw SQL
- 79. API with NestJS #79. Implementing searching with pattern matching and raw SQL
- 80. API with NestJS #80. Updating entities with PUT and PATCH using raw SQL queries
- 81. API with NestJS #81. Soft deletes with raw SQL queries
- 82. API with NestJS #82. Introduction to indexes with raw SQL queries
- 83. API with NestJS #83. Text search with tsvector and raw SQL
- 84. API with NestJS #84. Implementing filtering using subqueries with raw SQL
- 85. API with NestJS #85. Defining constraints with raw SQL
- 86. API with NestJS #86. Logging with the built-in logger when using raw SQL
- 87. API with NestJS #87. Writing unit tests in a project with raw SQL
- 88. API with NestJS #88. Testing a project with raw SQL using integration tests
- 89. API with NestJS #89. Replacing Express with Fastify
- 90. API with NestJS #90. Using various types of SQL joins
- 91. API with NestJS #91. Dockerizing a NestJS API with Docker Compose
- 92. API with NestJS #92. Increasing the developer experience with Docker Compose
- 93. API with NestJS #93. Deploying a NestJS app with Amazon ECS and RDS
- 94. API with NestJS #94. Deploying multiple instances on AWS with a load balancer
- 95. API with NestJS #95. CI/CD with Amazon ECS and GitHub Actions
- 96. API with NestJS #96. Running unit tests with CI/CD and GitHub Actions
- 97. API with NestJS #97. Introduction to managing logs with Amazon CloudWatch
- 98. API with NestJS #98. Health checks with Terminus and Amazon ECS
- 99. API with NestJS #99. Scaling the number of application instances with Amazon ECS
- 100. API with NestJS #100. The HTTPS protocol with Route 53 and AWS Certificate Manager
- 101. API with NestJS #101. Managing sensitive data using the AWS Secrets Manager
- 102. API with NestJS #102. Writing unit tests with Prisma
- 103. API with NestJS #103. Integration tests with Prisma
- 104. API with NestJS #104. Writing transactions with Prisma
- 105. API with NestJS #105. Implementing soft deletes with Prisma and middleware
- 106. API with NestJS #106. Improving performance through indexes with Prisma
- 107. API with NestJS #107. Offset and keyset pagination with Prisma
- 108. API with NestJS #108. Date and time with Prisma and PostgreSQL
- 109. API with NestJS #109. Arrays with PostgreSQL and Prisma
- 110. API with NestJS #110. Managing JSON data with PostgreSQL and Prisma
- 111. API with NestJS #111. Constraints with PostgreSQL and Prisma
- 112. API with NestJS #112. Serializing the response with Prisma
- 113. API with NestJS #113. Logging with Prisma
- 114. API with NestJS #114. Modifying data using PUT and PATCH methods with Prisma
- 115. API with NestJS #115. Database migrations with Prisma
- 116. API with NestJS #116. REST API versioning
- 117. API with NestJS #117. CORS – Cross-Origin Resource Sharing
- 118. API with NestJS #118. Uploading and streaming videos
- 119. API with NestJS #119. Type-safe SQL queries with Kysely and PostgreSQL
- 120. API with NestJS #120. One-to-one relationships with the Kysely query builder
- 121. API with NestJS #121. Many-to-one relationships with PostgreSQL and Kysely
- 122. API with NestJS #122. Many-to-many relationships with Kysely and PostgreSQL
- 123. API with NestJS #123. SQL transactions with Kysely
- 124. API with NestJS #124. Handling SQL constraints with Kysely
- 125. API with NestJS #125. Offset and keyset pagination with Kysely
- 126. API with NestJS #126. Improving the database performance with indexes and Kysely
- 127. API with NestJS #127. Arrays with PostgreSQL and Kysely
- 128. API with NestJS #128. Managing JSON data with PostgreSQL and Kysely
- 129. API with NestJS #129. Implementing soft deletes with SQL and Kysely
- 130. API with NestJS #130. Avoiding storing sensitive information in API logs
- 131. API with NestJS #131. Unit tests with PostgreSQL and Kysely
- 132. API with NestJS #132. Handling date and time in PostgreSQL with Kysely
- 133. API with NestJS #133. Introducing database normalization with PostgreSQL and Prisma
- 134. API with NestJS #134. Aggregating statistics with PostgreSQL and Prisma
- 135. API with NestJS #135. Referential actions and foreign keys in PostgreSQL with Prisma
- 136. API with NestJS #136. Raw SQL queries with Prisma and PostgreSQL range types
- 137. API with NestJS #137. Recursive relationships with Prisma and PostgreSQL
- 138. API with NestJS #138. Filtering records with Prisma
- 139. API with NestJS #139. Using UUID as primary keys with Prisma and PostgreSQL
- 140. API with NestJS #140. Using multiple PostgreSQL schemas with Prisma
- 141. API with NestJS #141. Getting distinct records with Prisma and PostgreSQL
- 142. API with NestJS #142. A video chat with WebRTC and React
- 143. API with NestJS #143. Optimizing queries with views using PostgreSQL and Kysely
- 144. API with NestJS #144. Creating CLI applications with the Nest Commander
- 145. API with NestJS #145. Securing applications with Helmet
- 146. API with NestJS #146. Polymorphic associations with PostgreSQL and Prisma
- 147. API with NestJS #147. The data types to store money with PostgreSQL and Prisma
- 148. API with NestJS #148. Understanding the injection scopes
When a NestJS application starts, it creates instances of various classes, such as controllers and services. By default, NestJS treats those classes as singletons, where a particular class has only one instance. NestJS then shares the single instance of each provider across the entire application’s lifetime, creating a singleton provider scope.
If you want to know more about the Singleton pattern, check out JavaScript design patterns #1. Singleton and the Module
The singleton scope fits most use cases. Thanks to creating just one instance of each provider and sharing it with all consumers, NestJS can cache them and increase performance. However, in some cases, we might want to change the default behavior.
The request scope
To change the default provider scope, we must provide the scope property to the @Injectable() decorator.
logged-in-user.service.ts
import { Injectable, Scope } from '@nestjs/common'; @Injectable({ scope: Scope.REQUEST }) export class LoggedInUserService { // ... |
Thanks to adding scope: Scope.REQUEST, our LoggedInUserService is initialized every time a user makes an HTTP request handled by a controller that uses this service.
Using the request object
The request object contains information about the HTTP request made to our API. Since our service is request-scoped, we can access the request object.
logged-in-user.service.ts
import { Inject, Injectable, Scope } from '@nestjs/common'; import { REQUEST } from '@nestjs/core'; import { Request } from 'express'; @Injectable({ scope: Scope.REQUEST }) export class LoggedInUserService { constructor(@Inject(REQUEST) private request: Request) {} |
It’s very common to implement authentication using JSON Web Tokens using the Passport library. With this approach, the information about the logged-in user is attached to the request object.
If you want to know more about authentication with NestJS, check out API with NestJS #3. Authenticating users with bcrypt, Passport, JWT, and cookies
To access the information about the user, we should create an interface that extends the Request interface imported from the Express library.
request-with-user.interface.ts
import { Request } from 'express'; import { Prisma } from '@prisma/client'; export interface RequestWithUser extends Request { user: Prisma.UserGetPayload<{ include: { address: true } }>; |
Using the UserGetPayload type from Prisma we can tell TypeScript that user property includes the address fetched through a relationship.
We’ve used this interface in other parts of our application before.
articles.controller.ts
import { Body, Controller, Post, UseGuards, } from '@nestjs/common'; import { ArticlesService } from './articles.service'; import { CreateArticleDto } from './dto/create-article.dto'; import { JwtAuthenticationGuard } from '../authentication/jwt-authentication.guard'; import { RequestWithUser } from '../authentication/request-with-user.interface'; @Controller('articles') export default class ArticlesController { constructor(private readonly articlesService: ArticlesService) {} @Post() @UseGuards(JwtAuthenticationGuard) create(@Body() article: CreateArticleDto, @Req() request: RequestWithUser) { return this.articlesService.create(article, request.user.id); // ... |
In the above case, the user property is always defined thanks to using the JwtAuthenticationGuard. If we want our LoggedInUserService to handle a situation when the user is not logged in, we can modify the RequestWithUser and make the user property optional. One way to do that would be to use the type-fest library.
logged-in-user.service.ts
import { Inject, Injectable, Scope } from '@nestjs/common'; import { REQUEST } from '@nestjs/core'; import { SetOptional } from 'type-fest'; import { RequestWithUser } from '../authentication/request-with-user.interface'; @Injectable({ scope: Scope.REQUEST }) export class LoggedInUserService { constructor( @Inject(REQUEST) private readonly request: SetOptional<RequestWithUser, 'user'>, getAddress() { return this.request.user?.address; |
We can now use our service in a controller, for example.
articles.controller.ts
import { Body, Controller, Post, Query, UseGuards, } from '@nestjs/common'; import { ArticlesService } from './articles.service'; import { CreateArticleDto } from './dto/create-article.dto'; import { JwtAuthenticationGuard } from '../authentication/jwt-authentication.guard'; import { RequestWithUser } from '../authentication/request-with-user.interface'; import { ArticlesSearchParamsDto } from './dto/articles-search-params.dto'; import { LoggedInUserService } from '../users/logged-in-user.service'; @Controller('articles') export default class ArticlesController { constructor( private readonly articlesService: ArticlesService, private readonly loggedInUserService: LoggedInUserService, @Get() getAll(@Query() searchParams: ArticlesSearchParamsDto) { console.log(this.loggedInUserService.getAddress()); return this.articlesService.search(searchParams); @Post() @UseGuards(JwtAuthenticationGuard) create(@Body() article: CreateArticleDto, @Req() request: RequestWithUser) { console.log(this.loggedInUserService.getAddress()); return this.articlesService.create(article, request.user.id); // ... |
We need to remember, though, that the request.user property will not be defined if we don’t use the JwtAuthenticationGuard, which requires the user to be logged in.
The scope hierarchy
A significant downside to having a request-scoped provider is that a controller that depends on a request-scoped provider is also request-scoped. Therefore, the ArticlesController is request-scoped because it uses the LoggedInUserService, which is request-scoped.
Using request-scoped providers will affect our application’s performance. Even though NestJS relies on cache under the hood, it still has to create an instance of each request-scoped provider. Therefore, we should use request-scoped providers sparingly if performance is one of our priorities.
The transient scope
When implementing logging, it’s a good practice to create a separate instance of the Logger class for each of our services. This way, we can provide the context to the Logger constructor.
articles.service.ts
import { Injectable, Logger } from '@nestjs/common'; import { PrismaService } from '../database/prisma.service'; import { ArticleNotFoundException } from './article-not-found.exception'; @Injectable() export class ArticlesService { logger = new Logger(ArticlesService.name) constructor(private readonly prismaService: PrismaService) {} async getById(id: number) { const article = await this.prismaService.article.findUnique({ where: { if (!article) { this.logger.warn('Tried to get an article that does not exist'); throw new ArticleNotFoundException(id); return article; // ... |
Thanks to this approach, we see that a particular log comes from the ArticlesService.
If you want to know more about logging in NestJS, check out API with NestJS #113. Logging with Prisma or API with NestJS #50. Introduction to logging with the built-in logger and TypeORM
With the default singleton scope, a single provider instance is shared across the entire application. However, with the transient scope, each provider receives a dedicated instance. We can use this to create a smart logger service.
logger.service.ts
import { Inject, Injectable, Logger, Scope } from '@nestjs/common'; import { INQUIRER } from '@nestjs/core'; import { Class } from 'type-fest'; @Injectable({ scope: Scope.TRANSIENT }) export class LoggerService { logger: Logger; constructor(@Inject(INQUIRER) private parentClass: Class<unknown>) { this.logger = new Logger(this.parentClass.constructor.name); |
Thanks to using @Inject(INQUIRER), we can access the parent class that used our LoggerService. Because of that, we no longer need to manually provide the name of each class every time we want to use the logger.
articles.service.ts
import { Injectable } from '@nestjs/common'; import { PrismaService } from '../database/prisma.service'; import { ArticleNotFoundException } from './article-not-found.exception'; import { LoggerService } from '../logger/logger.service'; @Injectable() export class ArticlesService { constructor( private readonly prismaService: PrismaService, private readonly loggerService: LoggerService async getById(id: number) { const article = await this.prismaService.article.findUnique({ where: { if (!article) { this.loggerService.logger.warn('Tried to get an article that does not exist'); throw new ArticleNotFoundException(id); return article; // ... |
NestJS creates an instance of the loggerService specifically for the ArticlesService. The loggerService is aware that it was created specifically for the ArticlesService and creates a new instance of the Logger.
Summary
In this article, we’ve discussed injection scopes and their use. With the request scope, we can create services and controllers that are reinitialized for each request. This can come in handy when we want to react to request headers, including the JSON Web Token. However, to avoid performance issues, we need to avoid overusing request-scoped providers.
With transient-scoped providers, we can create a dedicated instance for each consumer. This can be useful when we want to configure our service differently for various consumers. To understand that, we created a logger service that is configured based on the provider that imports it.
The knowledge of various injection scopes can definitely come in handy when dealing with various more advanced use cases in NestJS.
Series Navigation<< API with NestJS #147. The data types to store money with PostgreSQL and PrismaRecommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK