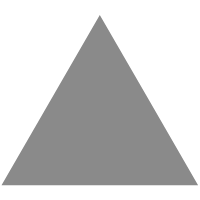
5
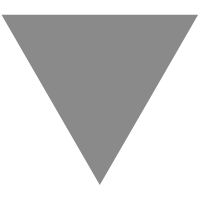
深入探讨 C++ 中的接口类封装技巧
source link: https://www.51cto.com/article/785134.html
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
深入探讨 C++ 中的接口类封装技巧
作者:Andy 2024-04-01 13:05:13
在实际编程中,合理地设计和使用接口类,能够使我们的代码更加清晰和易于理解,提高我们的编程效率和质量。
在C++编程中,接口类的封装是实现多态性和抽象性的重要手段之一。通过定义抽象基类和纯虚函数,可以实现统一的接口,让不同的派生类共享相同的接口,从而提高代码的灵活性和可维护性。
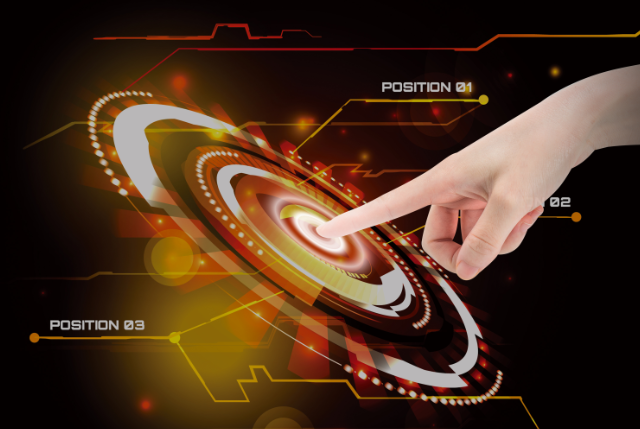
1. 使用纯虚函数
纯虚函数是在基类中声明的虚函数,它没有函数体,需要在派生类中进行实现。通过定义纯虚函数,我们可以定义一个抽象的接口,让不同的派生类实现自己的行为。
// 接口类
class Shape {
public:
// 纯虚函数
virtual double area() const = 0;
virtual double perimeter() const = 0;
virtual void draw() const = 0;
};
2. 接口类作为参数类型
接口类可以作为函数的参数类型,实现多态性。通过传递接口类的引用或指针,我们可以接受任何实现了该接口的对象,从而实现对不同对象的统一操作。
void printInfo(const Shape& shape) {
cout << "Area: " << shape.area() << endl;
cout << "Perimeter: " << shape.perimeter() << endl;
}
int main() {
Circle circle(5.0);
printInfo(circle); // 通过接口类的引用调用
return 0;
}
3. 使用接口类指针实现工厂模式
工厂模式是一种常见的设计模式,通过工厂函数返回接口类的指针,根据不同的需求返回不同的派生类实例,从而实现对象的创建与封装。
// 工厂函数
Shape* createShape(const string& type) {
if (type == "circle") {
return new Circle(5.0);
} else if (type == "rectangle") {
return new Rectangle(4.0, 6.0);
} else {
return nullptr;
}
}
int main() {
Shape* shapePtr = createShape("circle");
printInfo(*shapePtr);
delete shapePtr; // 清理内存
return 0;
}
通过以上代码示例,我们可以看到在C++中实现接口类的封装技巧。接口类的设计可以让我们更好地实现代码的抽象和多态,提高代码的可维护性和可扩展性。同时,通过工厂模式,我们可以实现对象的封装和创建过程的解耦,使代码更加灵活和易于维护。
在实际编程中,合理地设计和使用接口类,能够使我们的代码更加清晰和易于理解,提高我们的编程效率和质量。
责任编辑:赵宁宁
来源:
AI让生活更美好
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK