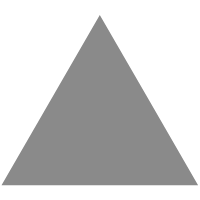
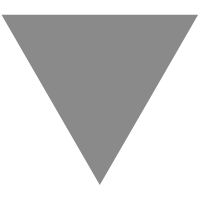
Micro frontends: Shell vs. Micro Apps
source link: https://blog.bitsrc.io/micro-frontends-shell-vs-micro-apps-5ad809a9b85a
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Micro frontends: Shell vs. Micro Apps
The two most common approaches for Micro frontend implementation
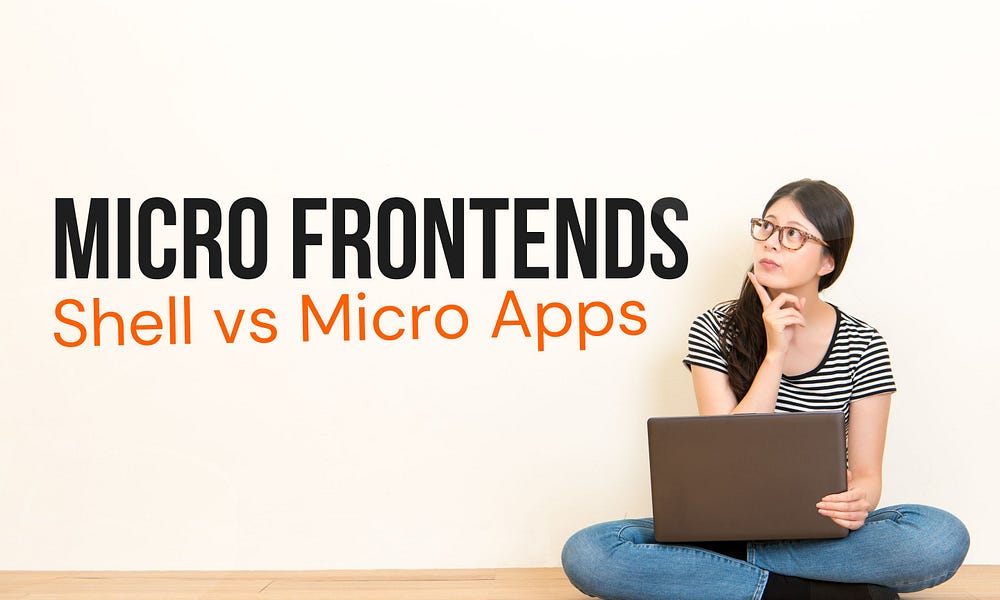
In the ever-evolving web development landscape, micro frontends have emerged as the most popular approach to large-scale, feature-rich frontend development.
At the heart of this architectural style are two distinct models: the Shell application and Micro Apps. Once you break the frontend monolith into more manageable pieces, each presents a unique strategy to compose them into the final application.
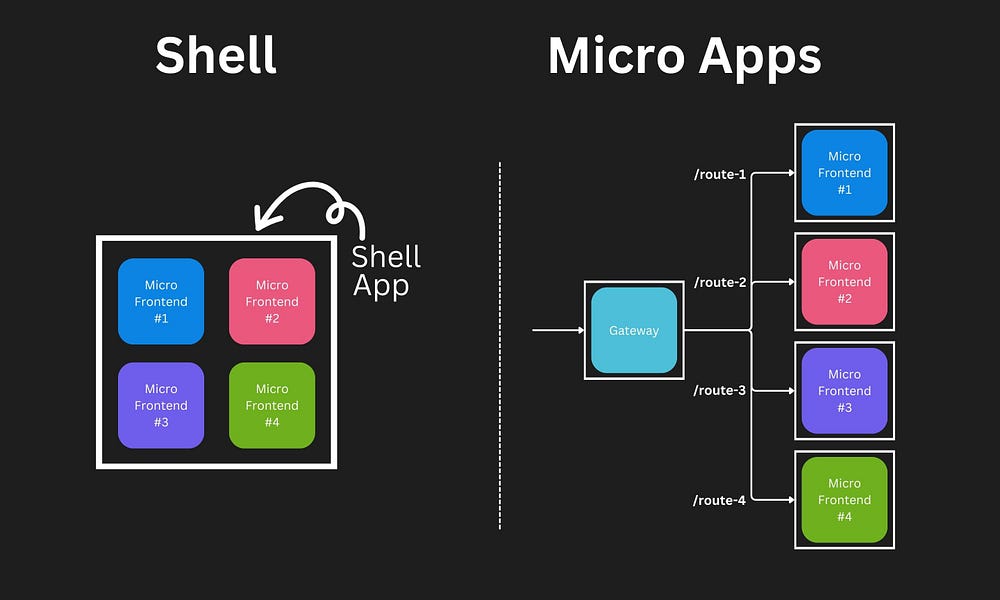
The idea behind the Shell app is pretty straightforward. First, we need to decompose the frontend into more manageable pieces. Then, we can create a wrapper application called the shell and combine these pieces within it. Typically, we do it at build time so that the outcome is more predictable.
The next approach to creating multiple micro frontends is by dividing them into multiple applications, owning a URL route, and serving them through a gateway proxy server. The easiest way to think of it as separate applications having their own URL path.
However, breaking a large frontend application into micro frontends has three main challenges.
Component Reuse
In frontends we must reuse UI components. It helps maintain UI consistency and inherit a common UI style across the application. But the challenge is that, unlike sharing UI components from a single package or a folder path, now you have multiple micro frontends owning different components, making it way more complex to implement a component reusing strategy.
If you are using a monorepo setup, you can still share packages from a common location. Still, it requires a context-aware build process, where once you modify any reusable component, you need to know its impact across different micro frontends.
This becomes further difficult with a polyrepo setup where you are either constrained to package shared components or put them into a separate repository. Either way, it makes the build process even more complex over time.
Splitting Micro Frontends
One of the most common challenges with micro frontends is deciding how to divide it upfront. Several factors may help in your decision, such as the different functional areas of the application, the complexity of each section, and the nature of your frontend team.
The challenge is that all three of these factors tend to change over time, where you need flexibility in changing the micro frontend structure. You may need to split some of the micro frontend into more pieces over time, create new micro frontends, or even combine several, which requires additional effort.
Managing Dependencies
You must still handle their dependencies once you break your frontend into pieces. In fact, it gets even more complex since you now have different pieces depending on different versions of internal and third-party dependencies.
For internal dependencies, you need to establish a solid process to keep your micro frontends up to date with the latest changes coming from peer teams. For third-party dependencies, you not only need a way to keep them up-to-date but also require an approach not to duplicate dependencies, which impact the overall bundle size of the application. You must look out for managing package peer dependencies and ensure their versions are in sync across micro frontends.
Considering all these factors, you need a robust set of tooling to handle your micro frontend platform where you can establish autonomy across your different micro frontend teams with high productivity.
Let’s explore how we can implement Shell vs Micro Apps addressing these challenges using Bit.
Micro Frontends with Shell
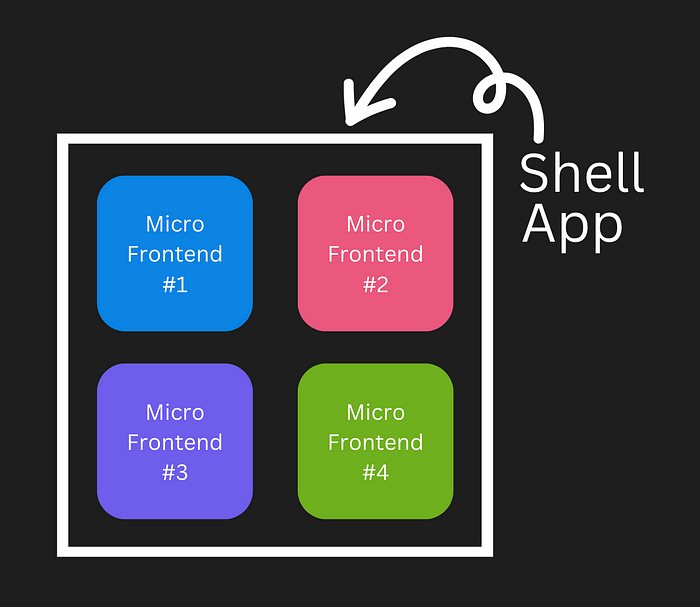
Bit helps you streamline how you break your frontend UI components. Since a component is a first-class citizen in Bit, where it’s stored in Bit cloud, you get the benefits of reusing components across micro frontends. Depending on your team structure, you can also group them into scopes where you can provide access control for each scope.
To understand further about the composable model and nature of Bit components, refer to the Thinking Bit.
You can use the Bit Harmony Quickstart to create a micro frontend shell. Run the following command to create a Harmony project.
bit new harmony my-project --env bitdev.symphony/envs/symphony-env --default-scope my-org.my-project
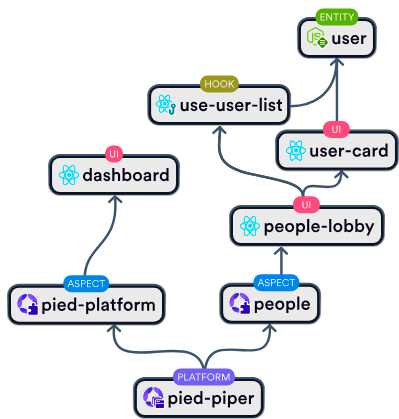
This command creates several components to illustrate the composable micro frontend architecture with a shell app.
In the platform browser runtime component, you can see how the shell uses different panels to render the components and the routes that trigger them.
import { SymphonyPlatformAspect, type SymphonyPlatformBrowser } from '@bitdev/symphony.symphony-platform';
import { Dashboard, Panel } from '@pied/pied-piper.ui.dashboard';
import { PiedTheme } from '@pied/design.pied-theme';
import type { PiedPlatformConfig } from './pied-platform-config.js';
import { PanelSlot } from './panel.js';
import { WelcomeCard } from './welcome-card.js';
export class PiedPlatformBrowser {
constructor(
private config: PiedPlatformConfig,
private panelSlot: PanelSlot
) {}
/**
* register a panel to the platform.
*/
registerPanel(panels: Panel[]) {
this.panelSlot.register(panels);
return this;
}
/**
* list the panels in the platform.
*/
listPanels() {
return this.panelSlot.flatValues();
}
static dependencies = [SymphonyPlatformAspect];
static defaultConfig: PiedPlatformConfig = {};
static async provider(
[symphonyPlatform]: [SymphonyPlatformBrowser],
config: PiedPlatformConfig,
[panelSlot]: [PanelSlot]
) {
const piedPlatform = new PiedPlatformBrowser(config, panelSlot);
symphonyPlatform.registerRoute([
{
path: '/',
component: () => {
const panels = piedPlatform.listPanels();
return <Dashboard panels={panels} />;
}
}
]);
piedPlatform.registerPanel([
{
component: () => {
return <WelcomeCard />;
}
}
]);
symphonyPlatform.registerTheme((props) => {
return <PiedTheme {...props} />;
});
return piedPlatform;
}
}
export default PiedPlatformBrowser;
Micro Apps
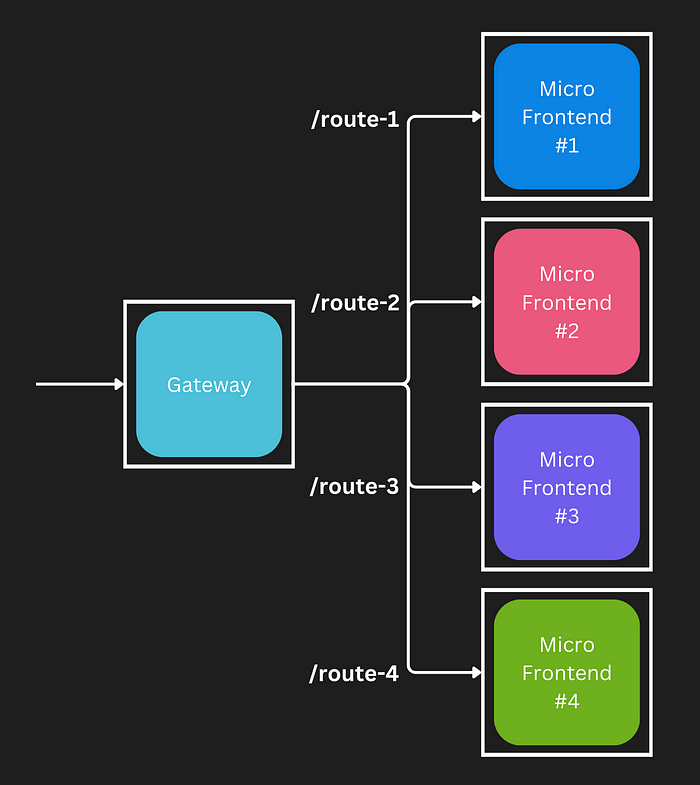
You can use the Bit Platform Quickstart to create the skeleton for micro apps. Run the following command to create a platform project.
bit new platform my-project --env bitdev.node/node-env --default-scope my-org.my-project
This command creates several components by default to demonstrate using micro frontends with composable architecture.
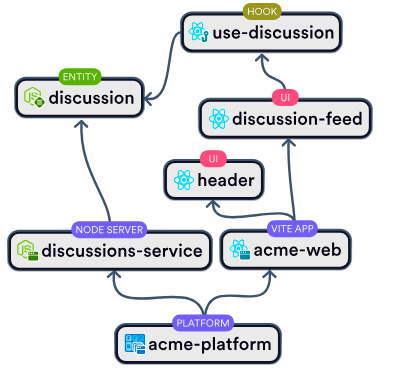
After running the command, it creates a platform app component where you can define your micro frontend components and route configuration.
import { Platform } from '@bitdev/platforms.platform';
const UserServer = import.meta.resolve('@bitdev/node.examples.user-server');
const DiscussionServer = import.meta.resolve('@acme/discussions.discussions-server');
const AcmeWeb = import.meta.resolve('@acme/acme.acme-web');
const PlatformGateway = import.meta.resolve('@bitdev/symphony.backends.gateway-server');
export const AcmePlatform = Platform.from({
name: 'acme-platform',
frontends: {
// main frontend application for the platform
main: AcmeWeb,
// define other micro frontends
},
// define backend services
});
export default AcmePlatform;
It also creates a Gateway server to proxy URL routes to the required micro frontend.
import type { GatewayServer } from '@bitdev/symphony.backends.gateway-server';
export class MyServer implements GatewayServer {
name = 'my-gateway';
run(context: BackendContext) {
const app = express();
// proxy requests here...
app.listen(context.port, () => {
console.log(listening to port ${context.port} );
});
}
};
Conclusion
As you can see, there are two main strategies to implement micro frontends. And it requires a robust toolset to reduce the overheads and gain the required productivity for the development teams.
Bit provides a comprehensive toolset, addressing most of the micro frontend challenges. And, using its quick starts, you can create your project structure in one go.
And there we have it. I hope you have found this useful. Thank you for reading!
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK