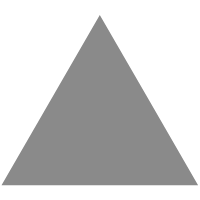
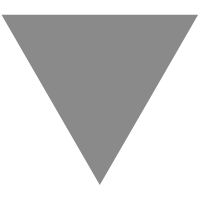
Understanding Python's dataclass Decorator
source link: https://dzone.com/articles/understanding-pythons-dataclass-decorator
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Understanding Python's dataclass Decorator
This tutorial explores the advantages, usage, ordering, immutability, and default value of Python's dataclass decorator.
@dataclass
is a decorator which is part of the Python dataclasses
module. When the @dataclass
decorator is used, it automatically generates special methods such as:
_ _ init _ _.
: Constructor to initialize fields_ _ repr _ _
: String representation of the object_ _ eq _ _
: Equality comparison between objects_ _ hash_ _
: Enables use as dictionary keys (if values are hashable)
Along with the methods listed above, the @dataclass
decorator has two important attributes.
- Order: If
True
, (the default isFalse
),__lt__()
,__le__()
,__gt__()
, and__ge__()
methods will be generated; i.e.,@dataclass (order = True)
. - Immutability: Fields can be made immutable using the
frozen=True
parameter; i.e.,@dataclass(frozen=True)
.
In a nutshell, the primary goal of the @dataclass
decorator is to simplify the creation of classes.
Advantages of the dataclass Decorator
Using the dataclass
decorator has several advantages:
- Boilerplate reduction: It reduces the amount of boilerplate code needed for classes by automatically generating common special methods.
- Readability: It improves the readability of the code by making it more concise and focused on the data representation.
- Default values: You can provide default values for attributes directly in the class definition, reducing the need for explicit
__init__()
methods. - Immutability:By combining
@dataclass
with thefrozen=True
option, you can create immutable data classes, ensuring that instances cannot be modified after creation.
Usage
from dataclasses import dataclass
@dataclass
class Person:
name: str
age: int
In this example, the Person
class is annotated with @dataclass
, and two fields (name
and age
), are declared. The __init__()
, __repr__()
, __eq__()
, and __hash__()
methods are automatically generated. Here's an explanation of how to use each generated method:
__init__(self, ...)
: The__init__
method is automatically generated with parameters corresponding to the annotated attributes. You can create instances of the class by providing values for the attributes.
person = Person('Sam', 45)
__repr__(self) -> str
: The__repr__
method returns a string representation of the object, useful for debugging and logging. When you print an object or use it in an f-string, the__repr__
method is called.
person # Person(name='Sam', age=45)
__eq__(self, other) -> bool
: The__eq__
method checks for equality between two objects based on their attributes. It is used when you compare objects using the equality operator(==)
.
# Usage
person1 = Person('Sam', 45)
person1
person2 = Person('Sam', 46)
person2
print(person1 == person2) # False.
__hash__(self) -> int
: The__hash__
method generates a hash value for the object, allowing instances to be used in sets and dictionaries. It is required when the class is used as a key in a dictionary or an element in a set.
Ordering
If you include the order=True
option, additional ordering methods (__lt__
, __le__
, __gt__
, and __ge__
) are generated. These methods allow instances to be compared using less than, less than or equal, greater than, and greater than or equal operators. If you perform a comparison on the Person
object without order
, TypeError
will be thrown.
print(person1 < person2)
# // TypeError: '<' not supported between instances of 'Person' and 'Person'
After adding ordering, we can perform comparisons.
@dataclass(order=True)
class Person:
name: str
age: int
# Usage
person1 = Person('Sam', 45)
person1
person2 = Person('Sam', 46)
person2
print(person1 < person2) # False.
order
is False
by default, meaning comparison methods are not generated unless explicitly enabled. Comparisons are based on field values, not object identities.
Immutability
@dataclass
can be made immutable using the frozen=True
attribute; the default is False
.
@dataclass
class Person:
name: str
age: int
person = Person('Sam', 45)
person.name = 'Sam2'
person # Person(name='Sam2', age=45)
In the code above, we are able to reassign values to the Person
name field. After adding frozen=True
, the exception will be thrown and reassignment is not allowed.
@dataclass(frozen=True)
class Person:
name: str
age: int
person = Person('Sam', 45)
person.name = 'Sam2'
# FrozenInstanceError: cannot assign to field 'name'
Be aware of performance implications: frozen=True
adds a slight overhead because of additional checks for immutability.
Default Value
Using the dataclasses
module, we can assign the default value to the fields in the class definition.
from dataclasses import dataclass, field
@dataclass
class Person:
name: str
age: int = field(default=20)
# Usage
person = Person('Sam')
person # Person(name='Sam', age=20)
Default values can be of any data type, including other data classes or mutable objects. They are evaluated only once when the class is defined, not each time an instance is created.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK