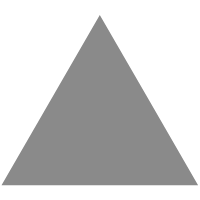
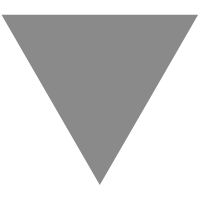
How to Convert Camel Case to Snake Case in PHP ?
source link: https://www.geeksforgeeks.org/how-to-convert-camel-case-to-snake-case-in-php/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
How to Convert Camel Case to Snake Case in PHP ?
Given a Camel Case String, the task is to convert the Camel Case String to a Snake Case String in PHP.
Examples:
Input: GeeksForGeeks
Output: geeks_for_geeks
Input: WelcomeToGfg
Output: welcome_to_gfg
Camel case uses capital letters at the beginning of each word except the first one, while snake case separates words with underscores and uses all lowercase letters.
Approach 1: Using Manual Conversion
The simplest way to convert camel case to snake case is to do it manually by iterating through each character of the string and inserting an underscore before each capital letter.
<?php function camelToSnake( $camelCase ) { $result = '' ; for ( $i = 0; $i < strlen ( $camelCase ); $i ++) { $char = $camelCase [ $i ]; if (ctype_upper( $char )) { $result .= '_' . strtolower ( $char ); } else { $result .= $char ; } } return ltrim( $result , '_' ); } // Driver code $camelCase = 'WelcomeToGeeksForGeeks' ; $snakeCase = camelToSnake( $camelCase ); echo $snakeCase ; ?> |
welcome_to_geeks_for_geeks
Approach 2: Using Regular Expressions
Regular expressions provide a concise way to achieve the conversion from camel case to snake case.
<?php function camelToSnake( $camelCase ) { $pattern = '/(?<=\\w)(?=[A-Z])|(?<=[a-z])(?=[0-9])/' ; $snakeCase = preg_replace( $pattern , '_' , $camelCase ); return strtolower ( $snakeCase ); } // Driver code $camelCase = 'WelcomeToGeeksForGeeks' ; $snakeCase = camelToSnake( $camelCase ); echo $snakeCase ; ?> |
welcome_to_geeks_for_geeks
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK