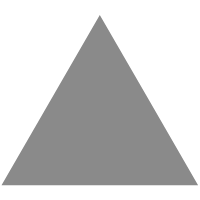
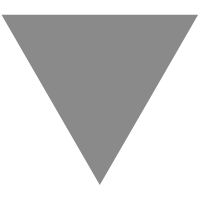
API with NestJS #138. Filtering records with Prisma
source link: https://wanago.io/2023/12/18/api-nestjs-prisma-sql-filtering/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.

December 18, 2023
- 1. API with NestJS #1. Controllers, routing and the module structure
- 2. API with NestJS #2. Setting up a PostgreSQL database with TypeORM
- 3. API with NestJS #3. Authenticating users with bcrypt, Passport, JWT, and cookies
- 4. API with NestJS #4. Error handling and data validation
- 5. API with NestJS #5. Serializing the response with interceptors
- 6. API with NestJS #6. Looking into dependency injection and modules
- 7. API with NestJS #7. Creating relationships with Postgres and TypeORM
- 8. API with NestJS #8. Writing unit tests
- 9. API with NestJS #9. Testing services and controllers with integration tests
- 10. API with NestJS #10. Uploading public files to Amazon S3
- 11. API with NestJS #11. Managing private files with Amazon S3
- 12. API with NestJS #12. Introduction to Elasticsearch
- 13. API with NestJS #13. Implementing refresh tokens using JWT
- 14. API with NestJS #14. Improving performance of our Postgres database with indexes
- 15. API with NestJS #15. Defining transactions with PostgreSQL and TypeORM
- 16. API with NestJS #16. Using the array data type with PostgreSQL and TypeORM
- 17. API with NestJS #17. Offset and keyset pagination with PostgreSQL and TypeORM
- 18. API with NestJS #18. Exploring the idea of microservices
- 19. API with NestJS #19. Using RabbitMQ to communicate with microservices
- 20. API with NestJS #20. Communicating with microservices using the gRPC framework
- 21. API with NestJS #21. An introduction to CQRS
- 22. API with NestJS #22. Storing JSON with PostgreSQL and TypeORM
- 23. API with NestJS #23. Implementing in-memory cache to increase the performance
- 24. API with NestJS #24. Cache with Redis. Running the app in a Node.js cluster
- 25. API with NestJS #25. Sending scheduled emails with cron and Nodemailer
- 26. API with NestJS #26. Real-time chat with WebSockets
- 27. API with NestJS #27. Introduction to GraphQL. Queries, mutations, and authentication
- 28. API with NestJS #28. Dealing in the N + 1 problem in GraphQL
- 29. API with NestJS #29. Real-time updates with GraphQL subscriptions
- 30. API with NestJS #30. Scalar types in GraphQL
- 31. API with NestJS #31. Two-factor authentication
- 32. API with NestJS #32. Introduction to Prisma with PostgreSQL
- 33. API with NestJS #33. Managing PostgreSQL relationships with Prisma
- 34. API with NestJS #34. Handling CPU-intensive tasks with queues
- 35. API with NestJS #35. Using server-side sessions instead of JSON Web Tokens
- 36. API with NestJS #36. Introduction to Stripe with React
- 37. API with NestJS #37. Using Stripe to save credit cards for future use
- 38. API with NestJS #38. Setting up recurring payments via subscriptions with Stripe
- 39. API with NestJS #39. Reacting to Stripe events with webhooks
- 40. API with NestJS #40. Confirming the email address
- 41. API with NestJS #41. Verifying phone numbers and sending SMS messages with Twilio
- 42. API with NestJS #42. Authenticating users with Google
- 43. API with NestJS #43. Introduction to MongoDB
- 44. API with NestJS #44. Implementing relationships with MongoDB
- 45. API with NestJS #45. Virtual properties with MongoDB and Mongoose
- 46. API with NestJS #46. Managing transactions with MongoDB and Mongoose
- 47. API with NestJS #47. Implementing pagination with MongoDB and Mongoose
- 48. API with NestJS #48. Definining indexes with MongoDB and Mongoose
- 49. API with NestJS #49. Updating with PUT and PATCH with MongoDB and Mongoose
- 50. API with NestJS #50. Introduction to logging with the built-in logger and TypeORM
- 51. API with NestJS #51. Health checks with Terminus and Datadog
- 52. API with NestJS #52. Generating documentation with Compodoc and JSDoc
- 53. API with NestJS #53. Implementing soft deletes with PostgreSQL and TypeORM
- 54. API with NestJS #54. Storing files inside a PostgreSQL database
- 55. API with NestJS #55. Uploading files to the server
- 56. API with NestJS #56. Authorization with roles and claims
- 57. API with NestJS #57. Composing classes with the mixin pattern
- 58. API with NestJS #58. Using ETag to implement cache and save bandwidth
- 59. API with NestJS #59. Introduction to a monorepo with Lerna and Yarn workspaces
- 60. API with NestJS #60. The OpenAPI specification and Swagger
- 61. API with NestJS #61. Dealing with circular dependencies
- 62. API with NestJS #62. Introduction to MikroORM with PostgreSQL
- 63. API with NestJS #63. Relationships with PostgreSQL and MikroORM
- 64. API with NestJS #64. Transactions with PostgreSQL and MikroORM
- 65. API with NestJS #65. Implementing soft deletes using MikroORM and filters
- 66. API with NestJS #66. Improving PostgreSQL performance with indexes using MikroORM
- 67. API with NestJS #67. Migrating to TypeORM 0.3
- 68. API with NestJS #68. Interacting with the application through REPL
- 69. API with NestJS #69. Database migrations with TypeORM
- 70. API with NestJS #70. Defining dynamic modules
- 71. API with NestJS #71. Introduction to feature flags
- 72. API with NestJS #72. Working with PostgreSQL using raw SQL queries
- 73. API with NestJS #73. One-to-one relationships with raw SQL queries
- 74. API with NestJS #74. Designing many-to-one relationships using raw SQL queries
- 75. API with NestJS #75. Many-to-many relationships using raw SQL queries
- 76. API with NestJS #76. Working with transactions using raw SQL queries
- 77. API with NestJS #77. Offset and keyset pagination with raw SQL queries
- 78. API with NestJS #78. Generating statistics using aggregate functions in raw SQL
- 79. API with NestJS #79. Implementing searching with pattern matching and raw SQL
- 80. API with NestJS #80. Updating entities with PUT and PATCH using raw SQL queries
- 81. API with NestJS #81. Soft deletes with raw SQL queries
- 82. API with NestJS #82. Introduction to indexes with raw SQL queries
- 83. API with NestJS #83. Text search with tsvector and raw SQL
- 84. API with NestJS #84. Implementing filtering using subqueries with raw SQL
- 85. API with NestJS #85. Defining constraints with raw SQL
- 86. API with NestJS #86. Logging with the built-in logger when using raw SQL
- 87. API with NestJS #87. Writing unit tests in a project with raw SQL
- 88. API with NestJS #88. Testing a project with raw SQL using integration tests
- 89. API with NestJS #89. Replacing Express with Fastify
- 90. API with NestJS #90. Using various types of SQL joins
- 91. API with NestJS #91. Dockerizing a NestJS API with Docker Compose
- 92. API with NestJS #92. Increasing the developer experience with Docker Compose
- 93. API with NestJS #93. Deploying a NestJS app with Amazon ECS and RDS
- 94. API with NestJS #94. Deploying multiple instances on AWS with a load balancer
- 95. API with NestJS #95. CI/CD with Amazon ECS and GitHub Actions
- 96. API with NestJS #96. Running unit tests with CI/CD and GitHub Actions
- 97. API with NestJS #97. Introduction to managing logs with Amazon CloudWatch
- 98. API with NestJS #98. Health checks with Terminus and Amazon ECS
- 99. API with NestJS #99. Scaling the number of application instances with Amazon ECS
- 100. API with NestJS #100. The HTTPS protocol with Route 53 and AWS Certificate Manager
- 101. API with NestJS #101. Managing sensitive data using the AWS Secrets Manager
- 102. API with NestJS #102. Writing unit tests with Prisma
- 103. API with NestJS #103. Integration tests with Prisma
- 104. API with NestJS #104. Writing transactions with Prisma
- 105. API with NestJS #105. Implementing soft deletes with Prisma and middleware
- 106. API with NestJS #106. Improving performance through indexes with Prisma
- 107. API with NestJS #107. Offset and keyset pagination with Prisma
- 108. API with NestJS #108. Date and time with Prisma and PostgreSQL
- 109. API with NestJS #109. Arrays with PostgreSQL and Prisma
- 110. API with NestJS #110. Managing JSON data with PostgreSQL and Prisma
- 111. API with NestJS #111. Constraints with PostgreSQL and Prisma
- 112. API with NestJS #112. Serializing the response with Prisma
- 113. API with NestJS #113. Logging with Prisma
- 114. API with NestJS #114. Modifying data using PUT and PATCH methods with Prisma
- 115. API with NestJS #115. Database migrations with Prisma
- 116. API with NestJS #116. REST API versioning
- 117. API with NestJS #117. CORS – Cross-Origin Resource Sharing
- 118. API with NestJS #118. Uploading and streaming videos
- 119. API with NestJS #119. Type-safe SQL queries with Kysely and PostgreSQL
- 120. API with NestJS #120. One-to-one relationships with the Kysely query builder
- 121. API with NestJS #121. Many-to-one relationships with PostgreSQL and Kysely
- 122. API with NestJS #122. Many-to-many relationships with Kysely and PostgreSQL
- 123. API with NestJS #123. SQL transactions with Kysely
- 124. API with NestJS #124. Handling SQL constraints with Kysely
- 125. API with NestJS #125. Offset and keyset pagination with Kysely
- 126. API with NestJS #126. Improving the database performance with indexes and Kysely
- 127. API with NestJS #127. Arrays with PostgreSQL and Kysely
- 128. API with NestJS #128. Managing JSON data with PostgreSQL and Kysely
- 129. API with NestJS #129. Implementing soft deletes with SQL and Kysely
- 130. API with NestJS #130. Avoiding storing sensitive information in API logs
- 131. API with NestJS #131. Unit tests with PostgreSQL and Kysely
- 132. API with NestJS #132. Handling date and time in PostgreSQL with Kysely
- 133. API with NestJS #133. Introducing database normalization with PostgreSQL and Prisma
- 134. API with NestJS #134. Aggregating statistics with PostgreSQL and Prisma
- 135. API with NestJS #135. Referential actions and foreign keys in PostgreSQL with Prisma
- 136. API with NestJS #136. Raw SQL queries with Prisma and PostgreSQL range types
- 137. API with NestJS #137. Recursive relationships with Prisma and PostgreSQL
- 138. API with NestJS #138. Filtering records with Prisma
Filtering records is one of the essential skills to have when working with SQL databases. In this article, we’ll implement various examples using NestJS and Prisma to show how to filter the data in different cases. Thanks to that, we will learn how to find precisely the data we need quickly and easily.
Implementing a search feature
In this series, we’ve often filtered records by providing the exact value we are looking for.
articles.service.ts
import { Injectable } from '@nestjs/common'; import { PrismaService } from '../database/prisma.service'; import { ArticleNotFoundException } from './article-not-found.exception'; @Injectable() export class ArticlesService { constructor(private readonly prismaService: PrismaService) {} // ... async getById(id: number) { const article = await this.prismaService.article.findUnique({ where: { if (!article) { throw new ArticleNotFoundException(id); return article; |
Besides searching for a row with a specific value, we can use various filtering operators. One of the most straightforward ones is contains.
articles.service.ts
import { Injectable } from '@nestjs/common'; import { PrismaService } from '../database/prisma.service'; @Injectable() export class ArticlesService { constructor(private readonly prismaService: PrismaService) {} searchByText(query: string) { return this.prismaService.article.findMany({ where: { content: { contains: query, mode: 'insensitive', // ... |
Thanks to adding mode: 'insensitive', our search is case-insensitive.
Let’s allow the users to search for the articles by sending a query parameter.
articles-search-service.ts
import { IsNotEmpty, IsOptional, IsString } from 'class-validator'; export class ArticlesSearchParamsDto { @IsOptional() @IsNotEmpty() @IsString() textSearch?: string | null; |
We can now use it in our controller.
articles.controller.ts
import { Controller, Get, Query } from '@nestjs/common'; import { ArticlesService } from './articles.service'; import { ArticlesSearchParamsDto } from './dto/articles-search-params.dto'; @Controller('articles') export default class ArticlesController { constructor(private readonly articlesService: ArticlesService) {} @Get() getAll(@Query() { textSearch }: ArticlesSearchParamsDto) { if (textSearch) { return this.articlesService.searchByText(textSearch); return this.articlesService.getAll(); // ... |
Thanks to this, the users can now make GET requests that filter the articles by the content.

Combining multiple filtering conditions
We can apply multiple search conditions in a single query.
Using the OR operator
For example, let’s search for articles containing a particular piece of text in the title or the content.
articles.service.ts
import { Injectable } from '@nestjs/common'; import { PrismaService } from '../database/prisma.service'; @Injectable() export class ArticlesService { constructor(private readonly prismaService: PrismaService) {} searchByText(query: string) { return this.prismaService.article.findMany({ where: { content: { contains: query, mode: 'insensitive', title: { contains: query, mode: 'insensitive', // ... |
Above, we are passing an array of conditions to the OR operator. Thanks to that, we include the article if either the content or the title matches the query.
Using the AND operator
We can also match articles that fulfill multiple conditions. Let’s allow the users to get the articles based on the number of upvotes.
articles-search-params.dto.ts
import { IsNotEmpty, IsNumber, IsOptional, IsString } from 'class-validator'; import { Type } from 'class-transformer'; export class ArticlesSearchParamsDto { @IsOptional() @IsNotEmpty() @IsString() textSearch?: string | null; @Type(() => Number) @IsOptional() @IsNumber() upvotesGreaterThan?: number | null; |
We need the AND operator to require the articles to fulfill more than one condition.
articles.service.ts
import { Injectable } from '@nestjs/common'; import { PrismaService } from '../database/prisma.service'; @Injectable() export class ArticlesService { constructor(private readonly prismaService: PrismaService) {} search(textSearch: string, upvotesGreaterThan: number) { return this.prismaService.article.findMany({ where: { AND: [ content: { contains: textSearch, mode: 'insensitive', upvotes: { gt: upvotesGreaterThan, // ... |
With the above approach, a particular article needs to have a specific text in its content, and have a particular number of upvotes.
We can take it a step further and combine the AND and OR operators.
articles.service.ts
import { Injectable } from '@nestjs/common'; import { PrismaService } from '../database/prisma.service'; @Injectable() export class ArticlesService { constructor(private readonly prismaService: PrismaService) {} search(textSearch: string, upvotesGreaterThan: number) { return this.prismaService.article.findMany({ where: { AND: [ content: { contains: textSearch, mode: 'insensitive', title: { contains: textSearch, mode: 'insensitive', upvotes: { gt: upvotesGreaterThan, // ... |
There is one issue with this approach, though. We should have the following cases:
- the user didn’t provide any search params,
- they provided only the text search param,
- they provided only the upvotes param,
- they provided both the text search param and the upvotes param.
To do that, we need to get a bit creative.
articles.service.ts
import { Injectable } from '@nestjs/common'; import { PrismaService } from '../database/prisma.service'; import { Prisma } from '@prisma/client'; import { ArticlesSearchParamsDto } from './dto/articles-search-params.dto'; @Injectable() export class ArticlesService { constructor(private readonly prismaService: PrismaService) {} search({ textSearch, upvotesGreaterThan }: ArticlesSearchParamsDto) { const searchInputs: Prisma.ArticleWhereInput[] = []; if (textSearch) { searchInputs.push({ content: { contains: textSearch, mode: 'insensitive', title: { contains: textSearch, mode: 'insensitive', if (typeof upvotesGreaterThan === 'number') { searchInputs.push({ upvotes: { gt: upvotesGreaterThan, if (!searchInputs.length) { return this.getAll(); if (searchInputs.length === 1) { return this.prismaService.article.findMany({ where: searchInputs[0], return this.prismaService.article.findMany({ where: { AND: searchInputs, getAll() { return this.prismaService.article.findMany(); // ... |
With this approach, we treat the search params differently based on how many of them the user provided:
- if they didn’t provide any, we call the getAll method,
- if they provided a single param, we don’t use the AND operator,
- we use the AND operator only if the user provided more than one search param.
This solution keeps our controller clean and simple because we only need to call the search method.
articles.controller.ts
import { Controller, Get, Query } from '@nestjs/common'; import { ArticlesService } from './articles.service'; import { ArticlesSearchParamsDto } from './dto/articles-search-params.dto'; @Controller('articles') export default class ArticlesController { constructor(private readonly articlesService: ArticlesService) {} @Get() getAll(@Query() searchParams: ArticlesSearchParamsDto) { return this.articlesService.search(searchParams); // ... |
Filtering on relationships
We can also use Prisma to filter the related records. Let’s allow the users to filter the articles by the category name.
articles-search-params.dto.ts
import { IsNotEmpty, IsNumber, IsOptional, IsString } from 'class-validator'; import { Type } from 'class-transformer'; export class ArticlesSearchParamsDto { @IsOptional() @IsNotEmpty() @IsString() textSearch?: string | null; @Type(() => Number) @IsOptional() @IsNumber() upvotesGreaterThan?: number | null; @IsOptional() @IsNotEmpty() @IsString() categoryName?: string | null; |
A single article can have multiple categories. Let’s use the some operator to get articles where at least one category has a particular name.
this.prismaService.article.findMany({ where: { categories: { some: { name: categoryName, |
The official documentation mentions more operators that can come in handy with relationships, such as every, or none.
Let’s add this filter to our search method.
articles.service.ts
import { BadRequestException, Injectable } from '@nestjs/common'; import { PrismaService } from '../database/prisma.service'; import { CreateArticleDto } from './dto/create-article.dto'; import { Prisma } from '@prisma/client'; import { PrismaError } from '../database/prisma-error.enum'; import { ArticleNotFoundException } from './article-not-found.exception'; import { UpdateArticleDto } from './dto/update-article.dto'; import { ArticlesSearchParamsDto } from './dto/articles-search-params.dto'; @Injectable() export class ArticlesService { constructor(private readonly prismaService: PrismaService) {} search({ textSearch, upvotesGreaterThan, categoryName, }: ArticlesSearchParamsDto) { const searchInputs: Prisma.ArticleWhereInput[] = []; if (categoryName) { searchInputs.push({ categories: { some: { name: categoryName, // ... return this.prismaService.article.findMany({ where: { AND: searchInputs, // ... |
Negating filters
Another helpful technique allows us to negate certain filters. For example, let’s enable the users to filter out the articles written by an author with a particular name.
articles-search-params.dto.ts
import { IsNotEmpty, IsNumber, IsOptional, IsString } from 'class-validator'; import { Type } from 'class-transformer'; export class ArticlesSearchParamsDto { @IsOptional() @IsNotEmpty() @IsString() textSearch?: string | null; @Type(() => Number) @IsOptional() @IsNumber() upvotesGreaterThan?: number | null; @IsOptional() @IsNotEmpty() @IsString() categoryName?: string | null; @IsOptional() @IsNotEmpty() @IsString() authorNameToAvoid?: string; |
To negate a particular filter, we can use the NOT operator.
this.prismaService.article.findMany({ where: { NOT: { author: { name: authorNameToAvoid, |
We can add it to our search method.
articles.service.ts
import { Injectable } from '@nestjs/common'; import { PrismaService } from '../database/prisma.service'; import { Prisma } from '@prisma/client'; import { ArticlesSearchParamsDto } from './dto/articles-search-params.dto'; @Injectable() export class ArticlesService { constructor(private readonly prismaService: PrismaService) {} search({ textSearch, upvotesGreaterThan, categoryName, authorNameToAvoid, }: ArticlesSearchParamsDto) { const searchInputs: Prisma.ArticleWhereInput[] = []; if (authorNameToAvoid) { searchInputs.push({ NOT: { author: { name: authorNameToAvoid, // ... return this.prismaService.article.findMany({ where: { AND: searchInputs, // ... |
Summary
In this article, we’ve learned how to filter records when using Prisma by implementing a search feature. By doing that, we learned how to implement conditional filtering when using the AND operator. While we included various real-life scenarios, Prisma mentions other useful operators in its official documentation. However, going through the examples from this article can give you a solid grasp of what Prisma can do.
Series Navigation<< API with NestJS #137. Recursive relationships with Prisma and PostgreSQL
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK