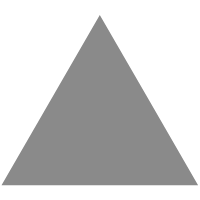
3
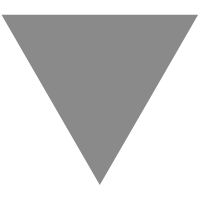
JAVAEE免费工程师教程之springboot综合案例
source link: https://blog.51cto.com/teayear/7887219
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
day04_springboot综合案例
查询所有用户执行流程

编写UserMapper接口
public interface UserMapper {
// 查询所有用户
List<UserInfo> findAllUsers();
}
编写UserService
public interface UserService extends UserDetailsService {
/**
* 查询所有用户
* @return
*/
List<UserInfo> findAllUsers(Integer page,Integer size);
}
@Service("userService")
public class UserServiceImpl implements UserService {
@Autowired
private UserMapper userMapper;
/**
* 查询所有用户
* @return
*/
@Override
public List<UserInfo> findAllUsers(Integer page,Integer size) {
PageHelper.startPage(page,size);
return this.userMapper.findAllUsers();
}
}
编写UserController
/**
* @Dese: 用户相关操作
*/
@Controller
@RequestMapping("user")
public class UserController {
@Autowired
private UserService userService;
/**
* 查询所有
* @param page
* @param size
* @param model
* @return
*/
@GetMapping("findAll")
public String findAll(@RequestParam(value = "page", defaultValue = "1") Integer page ,
@RequestParam(value = "size",defaultValue = "5") Integer size,
Model model){
PageHelper.startPage(page,size);
List<UserInfo> list = this.userService.findAll();
PageInfo pageInfo = new PageInfo(list);
model.addAttribute("pageInfo",pageInfo);
return "user-list";
}
}
编写UserMapper.xml
<!--查询所有用户-->
<select id="findAllUsers" resultType="UserInfo">
select * from users
</select>
修改UserInfo类
@Data
public class UserInfo {
private String id;
private String username;
private String email;
private String password;
private String phoneNum;
private int status;
private String statusStr;
private List<Role> roles;
public String getStatusStr() {
if (status == 0){
statusStr = "关闭";
}else if (status == 1){
statusStr = "开启";
}
return statusStr;
}
}

用户添加执行流程

编写UserMapper接口
public interface UserMapper {
/**
* 新增用户
* @param userInfo
*/
void save(UserInfo userInfo);
}
编写UserService
public interface UserService extends UserDetailsService {
/**
* 新增用户
* @param userInfo
*/
void save(UserInfo userInfo);
}
@Service
public class UserServiceImpl implements UserService {
@Autowired
private UserMapper userMapper;
@Autowired
private BCryptPasswordEncoder bCryptPasswordEncoder;
/**
* 新增用户
*/
@Override
public void save(UserInfo userInfo) {
//对密码进行加密处理
userInfo.setPassword(bCryptPasswordEncoder.encode(userInfo.getPassword()));
this.userMapper.save(userInfo);
}
}
编写UserController
@Controller
@RequestMapping("user")
public class UserController {
@Autowired
private UserService userService;
/**
* 新增用户
* @param userInfo
* @return
*/
@PostMapping("save")
public String save(UserInfo userInfo){
this.userService.save(userInfo);
return "redirect:findAll";
}
}
编写UserMapper.xml
<!--新增用户-->
<insert id="save">
insert into users(email,username,password,phoneNum,status)
values(#{email},#{username},#{password},#{phoneNum},#{status})
</insert>


Spring Security 密码加密
测试Spring Security 密码加密
public class BCryptPasswordEncoderUtil {
public static void main(String[] args) {
//第一次加密都不一样,通常情况下是无法逆向解密的
String encode = new BCryptPasswordEncoder().encode("520");
System.out.println(encode);
// 可以使用 new BCryptPasswordEncoder().matches()
// 方法来验证用户输入的密码是否与存储在数据库中的已加密密码匹配
boolean matches = new BCryptPasswordEncoder().matches("520", encode);
System.out.println(matches);
}
}
/**
* 新增用户
* @param userInfo
* @return
*/
@Override
public void save(UserInfo userInfo) {
//用户进行加密
userInfo.setPassword( new BCryptPasswordEncoder().encode(userInfo.getPassword()) );
this.userMapper.save(userInfo);
}
注意事项:登录认证时用户必须得有角色才能登录成功,添加用户后给出角色,才能登录
<!--登录认证-->
<resultMap id="ByUserNameAnRole" type="UserInfo" autoMapping="true">
<id property="id" column="uid"/>
<!--映射role-->
<collection property="roles" ofType="Role" javaType="List" autoMapping="true">
<id property="id" column="rid"/>
</collection>
</resultMap>
<select id="findUserByUserName" resultMap="ByUserNameAnRole">
SELECT
*,
u.id as uid,
r.id as rid
FROM
users u,
role r,
users_role ur
WHERE
u.id = ur.userId
AND r.id = ur.roleId
and
u.username = #{s}
</select>
用户详情执行流程
需求:根据id查询用户详情,要查询【用户】对应的【角色】信息,以及角色所包含的【资源权限】的信息
用户表,角色表,权限表,两个中间表

编写UserMapper接口
/**
* 用户详情
* @return
*/
UserInfo findById(Integer id);
编写UserService
public interface UserService extends UserDetailsService {
/**
* 用户详情
* @return
*/
UserInfo findById(Integer id);
}
}
@Service("userService")
public class UserServiceImpl implements UserService {
@Autowired
private UserMapper userMapper;
/**
* 用户详情
* @return
*/
@Override
public UserInfo findById(Integer id) {
return this.userMapper.findById(id);
}
}
编写UserController
@Controller
@RequestMapping("user")
public class UserController {
@Autowired
private UserService userService;
/**
* 用户详情
* @param id
* @return
*/
@GetMapping("findById")
public String findById(@RequestParam("id") Integer id, Model model){
UserInfo userInfo = this.userService.findById(id);
model.addAttribute("user",userInfo);
return "user-show";
}
}
编写UserMappr.xml
<!--用户详情-->
<resultMap id="userInfoById" type="UserInfo" autoMapping="true">
<id column="id" property="id"/>
<!--用户对应的角色-->
<collection property="roles" ofType="Role" javaType="List" autoMapping="true">
<id column="rid" property="id"/>
<!--角色对应的资源-->
<collection property="permissions" ofType="Permission" javaType="List" autoMapping="true">
<id column="pid" property="id"/>
</collection>
</collection>
</resultMap>
<select id="findById" resultMap="userInfoById">
SELECT
* ,
r.`id` rid,
p.`id` pid
FROM
users u
LEFT JOIN users_role ur ON ur.userId = u.id
LEFT JOIN role r ON ur.roleId = r.id
LEFT JOIN role_permission rp ON rp.roleId = r.id
LEFT JOIN permission p ON rp.permissionId = p.id
WHERE
u.id = #{id}
</select>

用户查询可添加角色
给用户添加角色,要查看当前这个用户有什么角色,哪些角色可以添加
编写UserController
/**
* 添加角色前。查询哪些角色可以添加
* @param id
* @return
*/
@GetMapping("findUserByIdAndAllRole")
public String findUserByIdAndAllRole(@RequestParam("id") Integer id , Model model){
//得知道给谁进行添加角色
UserInfo userInfo = this.userService.findById(id);
model.addAttribute("user",userInfo);
//根据用户id查询该用户哪些角色可以添加
List<Role> roleList = this.userService.findOtherRole(id);
model.addAttribute("roleList",roleList);
return "user-role-add";
}
编写UserService
/**
* 添加角色前,查询当前用户
* @param id
* @return
*/
UserInfo findUserByID(Integer id);
/**
* 添加角色前,查询当前用户可以添加的角色
* @param id
* @return
*/
List<Role> findOtherRoles(Integer id);
/**
* 添加角色前,查询当前用户
* @param id
* @return
*/
@Override
public UserInfo findUserByID(Integer id) {
return this.userMapper.findUserByID(id);
}
/**
* 添加角色前,查询当前用户可以添加的角色
* @param id
* @return
*/
@Override
public List<Role> findOtherRoles(Integer id) {
return this.userMapper.findOtherRoles(id);
}
编写UserMapper
/**
* 添加角色前,查询当前用户
* @param id
* @return
*/
UserInfo findUserByID(Integer id);
/**
* 添加角色前,查询当前用户可以添加的角色
* @param id
* @return
*/
List<Role> findOtherRoles(Integer id);
编写UserMapper.xml
<!--添加角色前,查询当前用户-->
<select id="findUserByID" resultType="UserInfo">
select * from users where id = #{id}
</select>
<!--添加角色前,查询当前用户可以添加的角色-->
<select id="findOtherRoles" resultType="Role">
select * from role where id not in(select roleId from users_role where userId = #{id})
</select>
查询当前用户哪些用户还可以添加什么角色
用户添加角色
给用户添加角色,用户与角色是多对多关系,操作中间表(users_role)即可
编写UserController
/**
* 给用户添加角色
* @param userId
* @param ids
* @return
*/
@PostMapping("addRoleToUser")
public String addRoleToUser(@RequestParam("userId") Integer userId , @RequestParam("ids") Integer[] ids){
this.userService.addRoleToUser(userId,ids);
return "redirect:findAll";
}
编写UserService
/**
* 给用户添加角色
* @param userId
* @param ids
* @return
*/
void addRoleToUser(Integer userId, Integer[] ids);
/**
* 给用户添加角色
* @param userId
* @param ids
* @return
*/
@Override
public void addRoleToUser(Integer userId, Integer[] ids) {
for(Integer roleid : ids){
this.userMapper.addRoleToUser(userId,roleid);
}
}
编写UserMapper
/**
* 给用户添加角色
* @param userId
* @return
*/
void addRoleToUser(@Param("userId") Integer userId,@Param("roleid") Integer roleid);
编写UserMapper.xml
<!--给用户添加角色-->
<insert id="addRoleToUser">
INSERT INTO `ssm_crud`.`users_role`(`userId`, `roleId`) VALUES (#{userId}, #{roleid});
</insert>
编写UserController
/**
* 删除用户
* @param id
* @return
*/
@GetMapping("deleteUser")
public String deleteUser(@RequestParam("id") Integer id){
//先把用户对应角色关系删除
this.userService.deleteUserRole(id);
//删除用户
this.userService.deleteUser(id);
return "redirect:findAll";
}
编写UserService
/**
* 删除用户对应的关系
* @param id
*/
@Override
public void deleteUserRole(Integer id) {
this.userMapper.deleteUserRole(id);
}
/**
* 删除用户
* @param id
*/
@Override
public void deleteUser(Integer id) {
this.userMapper.deleteUser(id);
}
编写UserMapper
/**
* 删除用户对应的关系
* @param id
*/
void deleteUserRole(Integer id);
/**
* 删除用户
* @param id
*/
void deleteUser(Integer id);
编写UserMapper.xml
<!--删除用户对应的关系-->
<delete id="deleteUserRole">
delete from users_role where userId = #{id}
</delete>
<!-- 删除用户-->
<delete id="deleteUser">
delete from users where id = #{id}
</delete>
blic void deleteUserRole(Integer id) {
this.userMapper.deleteUserRole(id);
}
/**
* 删除用户
* @param id
*/
@Override
public void deleteUser(Integer id) {
this.userMapper.deleteUser(id);
}
### 编写UserMapper
```java
/**
* 删除用户对应的关系
* @param id
*/
void deleteUserRole(Integer id);
/**
* 删除用户
* @param id
*/
void deleteUser(Integer id);
编写UserMapper.xml
<!--删除用户对应的关系-->
<delete id="deleteUserRole">
delete from users_role where userId = #{id}
</delete>
<!-- 删除用户-->
<delete id="deleteUser">
delete from users where id = #{id}
</delete>
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK