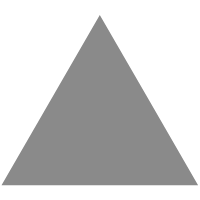
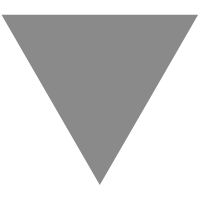
International mobile phone number input component for React Native
source link: https://reactnativeexample.com/international-mobile-phone-number-input-component-for-react-native/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
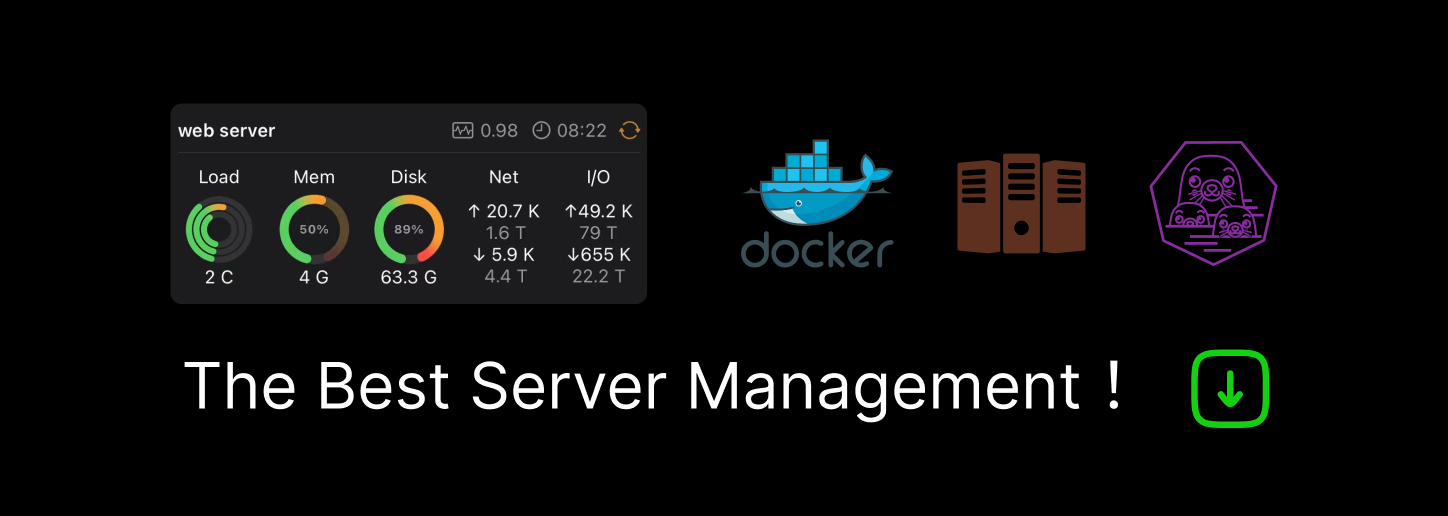
React Native International Phone Number Input

React Native International Phone Number Input
Try it out
Installation
$ npm i --save react-native-international-phone-number
$ yarn add react-native-international-phone-number
Update your
metro.config.js
file:module.exports = { ... resolver: { sourceExts: ['jsx', 'js', 'ts', 'tsx', 'cjs', 'json'], }, };
Features
- 📱 Works with iOS, Android (Cross-platform) and Expo;
- 🎨 Lib with UI customizable;
- 🌎 Phone Input Mask according to the selected country;
- 👨💻 Functional and class component support.
Basic Usage
-
Class Component:
import React from 'react';
import { View, Text } from 'react-native';
import { PhoneInput } from 'react-native-international-phone-number';
export class App extends React.Component {
constructor(props) {
super(props)
this.state = {
selectedCountry: undefined,
inputValue: ''
}
}
function handleSelectedCountry(country) {
this.setState({
selectedCountry: country
})
}
function handleInputValue(phoneNumber) {
this.setState({
inputValue: phoneNumber
})
}
render(){
return (
<View style={{ width: '100%', flex: 1, padding: 24 }}>
<PhoneInput
value={this.state.inputValue}
onChangePhoneNumber={this.handleInputValue}
selectedCountry={this.state.selectedCountry}
onChangeSelectedCountry={this.handleSelectedCountry}
/>
<View style={{ marginTop: 10 }}>
<Text>
Country:{' '}
{`${this.state.selectedCountry?.name} (${this.state.selectedCountry?.cca2})`}
</Text>
<Text>
Phone Number: {`${this.state.selectedCountry?.callingCode} ${this.state.inputValue}`}
</Text>
</View>
</View>
);
}
}
-
Function Component:
import React, { useState } from 'react';
import { View, Text } from 'react-native';
import { PhoneInput } from 'react-native-international-phone-number';
export default function App() {
const [selectedCountry, setSelectedCountry] = useState(undefined);
const [inputValue, setInputValue] = useState('');
function handleInputValue(phoneNumber) {
setInputValue(phoneNumber);
}
function handleSelectedCountry(country) {
setSelectedCountry(country);
}
return (
<View style={{ width: '100%', flex: 1, padding: 24 }}>
<PhoneInput
value={inputValue}
onChangePhoneNumber={handleInputValue}
selectedCountry={selectedCountry}
onChangeSelectedCountry={handleSelectedCountry}
/>
<View style={{ marginTop: 10 }}>
<Text>
Country:{' '}
{`${selectedCountry?.name} (${selectedCountry?.cca2})`}
</Text>
<Text>
Phone Number:{' '}
{`${selectedCountry?.callingCode} ${inputValue}`}
</Text>
</View>
</View>
);
}
-
Custom Default Flag:
import React, { useState } from 'react';
import { View, Text } from 'react-native';
import {
PhoneInput,
getCountryByCca2,
} from 'react-native-international-phone-number';
export default function App() {
const [selectedCountry, setSelectedCountry] = useState(
getCountryByCca2('CA') // <--- In this exemple, returns the CANADA Country and PhoneInput CANADA Flag
);
const [inputValue, setInputValue] = useState('');
function handleInputValue(phoneNumber) {
setInputValue(phoneNumber);
}
function handleSelectedCountry(country) {
setSelectedCountry(country);
}
return (
<View style={{ width: '100%', flex: 1, padding: 24 }}>
<PhoneInput
value={inputValue}
onChangePhoneNumber={handleInputValue}
selectedCountry={selectedCountry}
onChangeSelectedCountry={handleSelectedCountry}
/>
<View style={{ marginTop: 10 }}>
<Text>
Country:{' '}
{`${selectedCountry?.name} (${selectedCountry?.cca2})`}
</Text>
<Text>
Phone Number:{' '}
{`${selectedCountry?.callingCode} ${inputValue}`}
</Text>
</View>
</View>
);
}
Basic Usage - Typescript
import React, { useState } from 'react';
import { View, Text } from 'react-native';
import {
PhoneInput,
ICountry,
} from 'react-native-international-phone-number';
export default function App() {
const [selectedCountry, setSelectedCountry] = useState<
undefined | ICountry
>(undefined);
const [inputValue, setInputValue] = useState<string>('');
function handleInputValue(phoneNumber: string) {
setInputValue(phoneNumber);
}
function handleSelectedCountry(country: ICountry) {
setSelectedCountry(country);
}
return (
<View style={{ width: '100%', flex: 1, padding: 24 }}>
<PhoneInput
value={inputValue}
onChangePhoneNumber={handleInputValue}
selectedCountry={selectedCountry}
onChangeSelectedCountry={handleSelectedCountry}
/>
<View style={{ marginTop: 10 }}>
<Text>
Country:{' '}
{`${selectedCountry?.name} (${selectedCountry?.cca2})`}
</Text>
<Text>
Phone Number:{' '}
{`${selectedCountry?.callingCode} ${inputValue}`}
</Text>
</View>
</View>
);
}
Intermediate Usage - Typescript + Default Phone Number Value
import React, { useState } from 'react';
import { View, Text } from 'react-native';
import {
PhoneInput,
ICountry,
} from 'react-native-international-phone-number';
export default function App() {
const [selectedCountry, setSelectedCountry] = useState<
undefined | ICountry
>(undefined);
const [inputValue, setInputValue] = useState<string>('');
function handleInputValue(phoneNumber: string) {
setInputValue(phoneNumber);
}
function handleSelectedCountry(country: ICountry) {
setSelectedCountry(country);
}
return (
<View style={{ width: '100%', flex: 1, padding: 24 }}>
<PhoneInput
defaultValue="+12505550199"
value={inputValue}
onChangePhoneNumber={handleInputValue}
selectedCountry={selectedCountry}
onChangeSelectedCountry={handleSelectedCountry}
/>
<View style={{ marginTop: 10 }}>
<Text>
Country:{' '}
{`${selectedCountry?.name} (${selectedCountry?.cca2})`}
</Text>
<Text>
Phone Number:{' '}
{`${selectedCountry?.callingCode} ${inputValue}`}
</Text>
</View>
</View>
);
}
Observations:
- You need to use a default value with the following format:
+(country callling code)(area code)(number phone)
- The lib has the mechanism to set the flag and mask of the supplied
defaultValue
. However, if the supplieddefaultValue
does not match any international standard, theinput mask of the defaultValue
will be set to "BR" (please make sure that the default value is in the format mentioned above).
Advanced Usage - React-Hook-Form + Typescript + Default Phone Number Value
import React, { useState, useEffect } from 'react';
import { View, Text, TouchableOpacity, Alert } from 'react-native';
import {
PhoneInput,
ICountry,
} from 'react-native-international-phone-number';
import { Controller, FieldValues, useForm } from 'react-hook-form';
interface FormProps extends FieldValues {
phoneNumber: string;
}
export default function App() {
const [selectedCountry, setSelectedCountry] = useState<
undefined | ICountry
>(undefined);
const { control, handleSubmit, setValue, watch } =
useForm<FormProps>();
function handleSelectedCountry(country: ICountry) {
setSelectedCountry(country);
}
function onSubmit(form: FormProps) {
Alert.alert(
'Advanced Result',
`${selectedCountry?.callingCode} ${form.phoneNumber}`
);
}
useEffect(() => {
const watchPhoneNumber = watch('phoneNumber');
setValue('phoneNumber', watchPhoneNumber);
}, []);
return (
<View style={{ width: '100%', flex: 1, padding: 24 }}>
<Controller
name="phoneNumber"
control={control}
render={({ field: { onChange, value } }) => (
<PhoneInput
defaultValue="+12505550199"
value={value}
onChangePhoneNumber={onChange}
selectedCountry={selectedCountry}
onChangeSelectedCountry={handleSelectedCountry}
/>
)}
/>
<TouchableOpacity
style={{
width: '100%',
paddingVertical: 12,
backgroundColor: '#2196F3',
borderRadius: 4,
}}
onPress={handleSubmit(onSubmit)}
>
<Text
style={{
color: '#F3F3F3',
textAlign: 'center',
fontSize: 16,
fontWeight: 'bold',
}}
>
Submit
</Text>
</TouchableOpacity>
</View>
);
}
Observations:
- You need to use a default value with the following format:
+(country callling code)(area code)(number phone)
- The lib has the mechanism to set the flag and mask of the supplied
defaultValue
. However, if the supplieddefaultValue
does not match any international standard, theinput mask of the defaultValue
will be set to "BR" (please make sure that the default value is in the format mentioned above).
Customizing lib
-
Dark Mode:
<PhoneInput
...
withDarkTheme
/>
-
Custom Lib Styles:

<PhoneInput
...
inputStyle={{
color: '#F3F3F3',
}}
containerStyle={{
backgroundColor: '#575757',
borderWidth: 1,
borderStyle: 'solid',
borderColor: '#F3F3F3',
marginVertical: 16,
}}
flagContainerStyle={{
borderTopLeftRadius: 7,
borderBottomLeftRadius: 7,
backgroundColor: '#808080',
justifyContent: 'center',
}}
/>
-
Disabled Mode:
<PhoneInput
...
disabled
/>
-
Custom Disabled Mode Style:
...
const [isDisabled, setIsDisabled] = useState<boolean>(true)
...
<PhoneInput
...
containerStyle={ isDisabled ? { backgroundColor: 'yellow' } : {} }
disabled={isDisabled}
/>
...
Props
defaultValue?:
string;value:
string;onChangePhoneNumber:
(phoneNumber: string) => void;selectedCountry:
undefined | ICountry;onChangeSelectedCountry:
(country: ICountry) => void;disabled?:
boolean;withDarkTheme?:
boolean;containerStyle?:
StyleProp<ViewStyle>;flagContainerStyle?:
StyleProp<ViewStyle>;inputStyle?:
StyleProp<TextStyle>;
Functions
getAllCountries:
() => ICountry[];getCountryByPhoneNumber:
(phoneNumber: string) => ICountry | undefined;getCountryByCca2:
(phoneNumber: string) => ICountry | undefined;getCountriesByCallingCode:
(phoneNumber: string) => ICountry[] | undefined;getCountriesByName:
(phoneNumber: string) => ICountry[] | undefined;
License
GitHub
https://github.com/AstrOOnauta/react-native-international-phone-number
Recommend
-
17
Supercharging Kilian ValkhofBuilding tools that make developers awesome. Search term Supercharging <input type=number>
-
7
How to Implement the Automatic Bill Number Input Function Using HUAWEI ML Kit’s Text Recognition ...
-
14
use-count-up React/React Native component and hook to animate counting up or down to a number. Key features :trophy: Lighter implementation and smaller bundle size
-
5
react-native-datefield A simple React Native date input component. Installation yarn add react-native-d...
-
6
react-native-intl-phone-field React Native text input for validating international phone numbers, built on top of libphonenumber by Google using
-
8
Ask HN: How can scam callers fake a mobile phone number? Ask HN: How can scam callers fake a mobile phone number? 99 points by
-
5
Different format number depending on mobile phone or business advertisements Currently in my php application I have the fol...
-
7
Input Prompt component with single input field for react native Jun 13, 2022 1 min read
-
7
📱 React Native Numeric Pad 📱 A React Native component for amount or verification code input. It easily handles both decimals and integers, and runs smoothly for both IOS and Android. The design is simple and clear with numbers, dot, and o...
-
9
react-native-otp-entry react-native-otp-entry is a simple and highly customizable React Native component for entering OTP (One-Time Password). It provides an intuitive and user-friendly interface for inputting one-time passwo...
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK