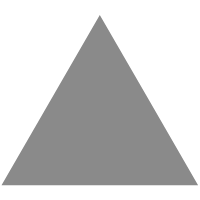
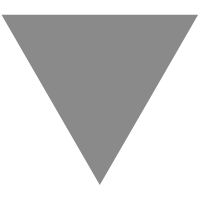
Selenium Automation Testing: Getting Started | Keyhole Software
source link: https://keyholesoftware.com/2023/07/17/getting-started-with-selenium-automation/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Selenium Automation Testing: Getting Started

Automated testing is a great way to ensure that any application can continue to grow and change while still giving fast and practical feedback to developers. This feedback can tell developers whether or not the changes introduced meet the requirements of the product and don’t introduce bugs.
As discussed in a previous blog series, automated testing can be a valuable resource when trying to deliver both agile and maintainable applications. But where should you get started and what technologies should you use to build automated tests?
In this post, I will discuss how to get started with and how to build out a simple automated test in one of the most popular options: Selenium.
What Is Selenium Automation Testing?
Selenium is an open-source test automation framework that allows developers and testers to automate web application testing across different browsers and platforms. It provides a suite of tools and libraries that facilitate the creation, execution, and maintenance of automated tests.
Selenium supports various programming languages, including Java, Python, C#, and JavaScript, making it accessible to a wide range of developers for a wide range of needs and projects.
Why Use Selenium Automation Testing?
Selenium automation testing provides several significant advantages over manual testing:
- Faster Test Execution: Automated tests can be executed much faster than manual tests, and Selenium enables the parallel execution of tests across multiple browsers and platforms. This effectively reduces test execution time and provides rapid feedback on the application’s quality.
- Improved Test Coverage: With Selenium, testers can create comprehensive test suites that cover a wide range of scenarios, edge cases, and functionalities. This helps ensure that critical aspects of the application are thoroughly tested, leading to enhanced software quality.
- Regression Testing: Selenium is particularly useful for performing regression testing, which involves retesting previously tested functionalities to ensure that new changes or updates have not introduced any issues. By automating regression tests, Selenium minimizes the effort required for repetitive testing, allowing testers to focus on other critical areas.
- Cross-Browser Compatibility: Selenium’s ability to automate tests across different browsers and versions ensures consistent behavior and helps identify browser-specific issues. This compatibility allows for efficient cross-browser testing, ensuring the application functions correctly across various environments.
- Integration with CI/CD Pipelines: Selenium seamlessly integrates with popular Continuous Integration (CI) and Continuous Deployment (CD) tools, enabling test automation to be an integral part of the software development lifecycle. Automated tests can be triggered automatically whenever new code is committed, ensuring that changes do not introduce regressions or breaking changes.
What Version to Pick?
Like most open-source, widely used software, Selenium has many flavors and ways to use it. This includes a dedicated IDE, a tool for running tests on multiple machines and environments all at the same time, as well as tools for integrating Selenium into other established testing software such as TestNG, Jenkins, and JUnit.
While all of these options are impressive and useful, I feel they are topics best saved for another day. Instead, I will focus on how to write the simplest of Selenium tests using the most common version of Selenium: Selenium Webdriver.
Set-Up
Selenium can be run in most IDEs, but for this blog, I will be setting up my intro test in Visual Studio Code. First, you’ll need Visual Studio Code installed.
Next, you’ll need to make sure that you have node installed in the folder you want to make your tests. If you don’t already have node installed you can install if from the command line using:
npm install
Then go to selenium.dev/download/ to find the language-specific bindings you’ll need. For this project, I will be using JavaScript.
You will then need to install the Selenium Webdriver, which you can do by using:
npm install selenium webdriver
Now we’re ready to start building out our test. For this test, we will be building out an automated test that Google searches for Keyhole Software, clicks the link to go to https://keyholesoftware.com/, and returns the title of the homepage.
To start, let’s put in the required constants for the automation to use Webdriver and open up a new window of Google Chrome.
const {By,Key,Builder} = require("selenium-webdriver"); require("chromedriver");
Next, we are going to create and call a method that will contain the string we want to search on Google as well as the instruction to open up a new window in Google Chrome.
async function example(){ var searchString = "Keyhole Software"; let driver = await new Builder().forBrowser("chrome").build(); await driver.get("http://google.com"); } example()
The following line will then be added to the method, which will insert our search string into Google’s search bar and hit the enter key.
await driver.findElement(By.name("q")).sendKeys(searchString,Key.RETURN);
After that, we need to be able to find the correct link that will take us to the Keyhole Software homepage and click it.
await driver.findElement(By.partialLinkText ("Keyhole Software")).click();
And finally, we need to get the title of the page that we have landed on and print it to the console log.
var title = await driver.getTitle(); console.log('Title is:',title);
After running this file, you should be greeted with a statement verifying that the automation did in fact make it to the Keyhole Software homepage!
Conclusion
Now this is obviously a very simple, basic example of what Selenium is capable of. One can easily run complex tests that verify everything from data to dates and click through hundreds of links across multiple machines before a human would even be able to tell what had just happened.
Selenium is an ever-growing piece of technology that has a large community of support across many languages and platforms. It’s quick to pick up and start building with.
Automated testing is an invaluable resource in the struggle to build applications quickly and accurately, and Selenium automated testing is one of the best, easiest-to-understand tools around in making that happen.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK