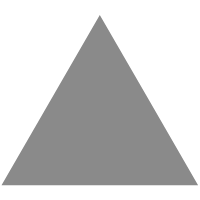
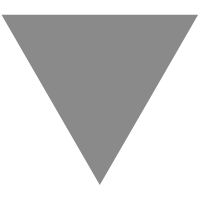
Get sum of values of a Dictionary in Python
source link: https://thispointer.com/get-sum-of-values-of-a-dictionary-in-python/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Get sum of values of a Dictionary in Python
This tutorial will discuss how to get sum of values of a dictionary in Python.
To get the sum of all values in a dictionary, we can call the values() method of the dictionary. It returns an iterable sequence of all the values from the dictionary and then we can pass those values into the sum() function. It will return the sum of all the values from the dictionary.
For instance, we have a dictionary that contains student data like names and ages. Like this,
student_data = { "Varun Sharma": 21, "Renuka Singh": 19, "Sachin Roy": 20}
Now, if we want to get the total age, we can retrieve all values from the dictionary using the values() method, then pass them into the sum function, which will return the total age. Like this,
total_age = sum(student_data.values())
It will add all the values of Dictionary into single numeric value.
Let’s see the complete example,
# Student data dictionary with names # as keys and ages as values student_data = { "Varun Sharma": 21, "Renuka Singh": 19, "Sachin Roy": 20} # Calculate the sum of ages total_age = sum(student_data.values()) # Print the sum of ages print("Sum of ages:", total_age)
Output
Sum of ages: 60
Summary
Today, we learned how to get sum of values of a dictionary in Python.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK