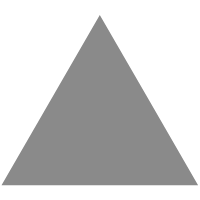
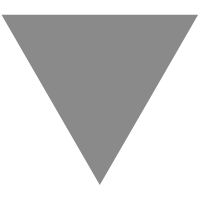
Pie Chart Example using Google Chart in Laravel 7
source link: https://www.laravelcode.com/post/pie-chart-example-using-google-chart-in-laravel-7
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
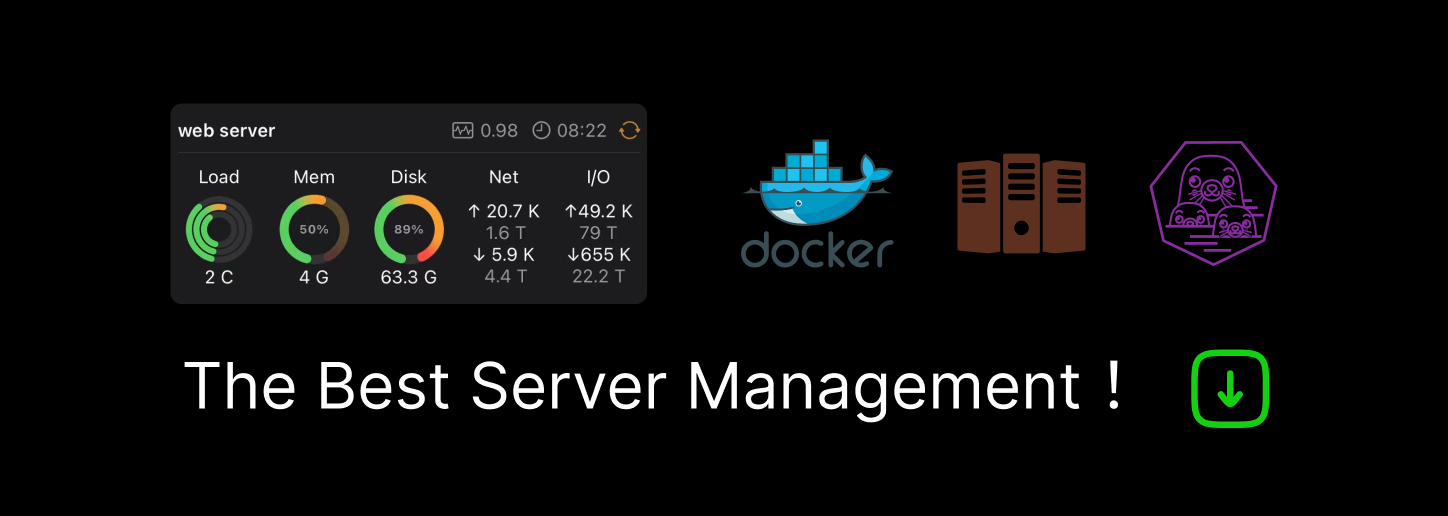
Pie Chart Example using Google Chart in Laravel 7
Hello Artisan
In this tutorial i will show you laravel chart example from scratch. I will discuss about laravel charts step by step so that you can understand. To engender dynamic pie chart in laravel i will utilize google chart api.
Google Charts provides an impeccable way to visualize data on your website. You can do dynamic pie chart in laravel utilizing chart js with laravel. But here i will utilize google chart.
In this tutorial i will show you 2d and 3d pie chart where you can show that how to transmute google chart options. If you don't ken how to utilize google chart to make pie chart, then you are a right place.
In this tutorial i will engender a product table and then we will engender 2d and 3d pie chart from product data. So let's engender dynamic charts in laravel utilizing google charts api with laravel.
Step 1: Install Laravel 7
In this step we have to download our fresh laravel app so that we can start from scratch. So run below command to download it\
composer create-project --prefer-dist laravel/laravel blog
Step 2: Create Model and Migration
Now time to create our product table and migration.
php artisan make:model Product -fm
Now open product model and set it like below
app/Product.php
namespace App;
use Illuminate\Database\Eloquent\Model;
class Product extends Model
{
protected $guarded = [];
}
After doing it we need to create our migration to migrate our database.
database/migrations/creates_products_table.php
Schema::create('products', function (Blueprint $table) {
$table->id();
$table->string("name")->nullable();
$table->string("sku")->nullable();
$table->string("description")->nullable();
$table->string("price")->nullable();
$table->integer("quantity");
$table->integer("sales");
$table->timestamps();
});
now run migrate command.
php artisan migrate
Now we have to create our factory to generate some dummy products.
database/factories/ProductFactory.php
/** @var \Illuminate\Database\Eloquent\Factory $factory */
use App\Product;
use Faker\Generator as Faker;
$factory->define(Product::class, function (Faker $faker) {
return [
"name" => $faker->word,
"sku" => $faker->unique()->randomNumber,
"description" => \Str::random(20),
"price" => $faker->numberBetween(1000, 10000),
"quantity" => $faker->numberBetween(1,100),
"sales" => $faker->numberBetween(1,100)
];
});
Now open your terminal and paste it in your termial to insert some fake product in products table.
php artisan tinker
//then
factory(\App\Product::class,100)->create()
Step 3: Create Route
Now we need to create our route to fetch our data from database. Paste below code to web.php file.
routes/web.php
Route::get("pie", "ProductController@get_all_products_for_pie_chart");
Step 4: Create Controller
Now we need to create get_all_products_for_pie_chart method in product controller. So create it.
php artisan make:controller ProductController
Now open it and paste this below code.
app/Http/Controllers/ProductController.php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
class ProductController extends Controller
{
public function get_all_products_for_pie_chart()
{
$products = \App\Product::all();
return view('pie',compact('products'));
}
}
Step 5: Create Blade File
Now we have to create pie.blade.php file to visualize our dynamic pie chart in laravel. So create it and paste this below code.
resources/views/pie.blade.php
<!doctype html>
<html lang="en">
<head>
<title>Google Pie Chart | LaravelCode</title>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1, shrink-to-fit=no">
<link rel="stylesheet" href="https://stackpath.bootstrapcdn.com/bootstrap/4.3.1/css/bootstrap.min.css" integrity="sha384-ggOyR0iXCbMQv3Xipma34MD+dH/1fQ784/j6cY/iJTQUOhcWr7x9JvoRxT2MZw1T" crossorigin="anonymous">
</head>
<body>
<div class="container p-5">
<h5>Google Pie Chart | LaravelCode</h5>
<div id="piechart" style="width: 900px; height: 500px;"></div>
</div>
<script type="text/javascript" src="https://www.gstatic.com/charts/loader.js"></script>
<script type="text/javascript">
google.charts.load('current', {'packages':['corechart']});
google.charts.setOnLoadCallback(drawChart);
function drawChart() {
var data = google.visualization.arrayToDataTable([
['Product Name', 'Sales', 'Quantity'],
@php
foreach($products as $product) {
echo "['".$product->name."', ".$product->sales.", ".$product->quantity."],";
}
@endphp
]);
var options = {
title: 'Product Details',
is3D: false,
};
var chart = new google.visualization.PieChart(document.getElementById('piechart'));
chart.draw(data, options);
}
</script>
</body>
</html>
i hope you like this article.
Author : Harsukh Makwana

Hi, My name is Harsukh Makwana. i have been work with many programming language like php, python, javascript, node, react, anguler, etc.. since last 5 year. if you have any issue or want me hire then contact me on [email protected]
Recommend
-
14
-
6
DRK 4 : 3D Pie Chart Example Thursday, July 31, 2003 Peter Hall has put together a very cool example of the 3D Pie Chart component from the
-
9
A. Introduction The last chapter, we will create a simple Pie Chart using FL Chart Package. B. Step To Create Pie Chart If you alrea...
-
11
Laravel 5 Chart example using Charts Package 393228 views 4 years ago Laravel
-
10
Google pie chart with ajax request example in PHP 986 views 6 months ago PHP In this tutorial, we will go through imple...
-
11
-
5
Plot a Pie Chart in Python using MatplotlibPlot a Pie Chart in Python using Matplotlib50 Views20/06/2022In this video, we are going to see...
-
5
Ranked #4 for todayPie Chart MakerCreate a Pie Chart for free with easy to use toolsPie Chart Maker online. Create a Pie Chart for...
-
6
Laravel 8 Google Chart Example Tutorial 535 views 10 months ago Laravel Google chart is simple, open-source and fre...
-
4
Bar Chart Example using Google Chart in Laravel 7 28955 views 2 years ago Laravel Hello Artisan Hope you are doing...
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK