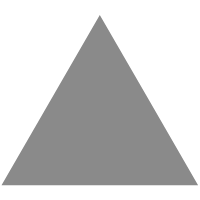
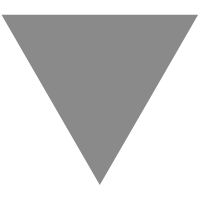
What is JavaScript? - A Beginner's Guide
source link: https://hackernoon.com/what-is-javascript-a-beginners-guide
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
What is JavaScript?
What is JavaScript? - A Beginner's Guide
7 min
by @odafetoearth
Odafe Alaiya
@odafetoearth
I am a Full stack dev who enjoys researching and...
Too Long; Didn't Read
JavaScript is a high-level, dynamically typed programming language. It allows you to write programs in more than one language. In this article, we'll explore the fundamentals of JavaScript and how it can be used to create dynamic, interactive web pages. We'll also discuss the history of JavaScript, its impact on web development, and some of the basic concepts to help you get started.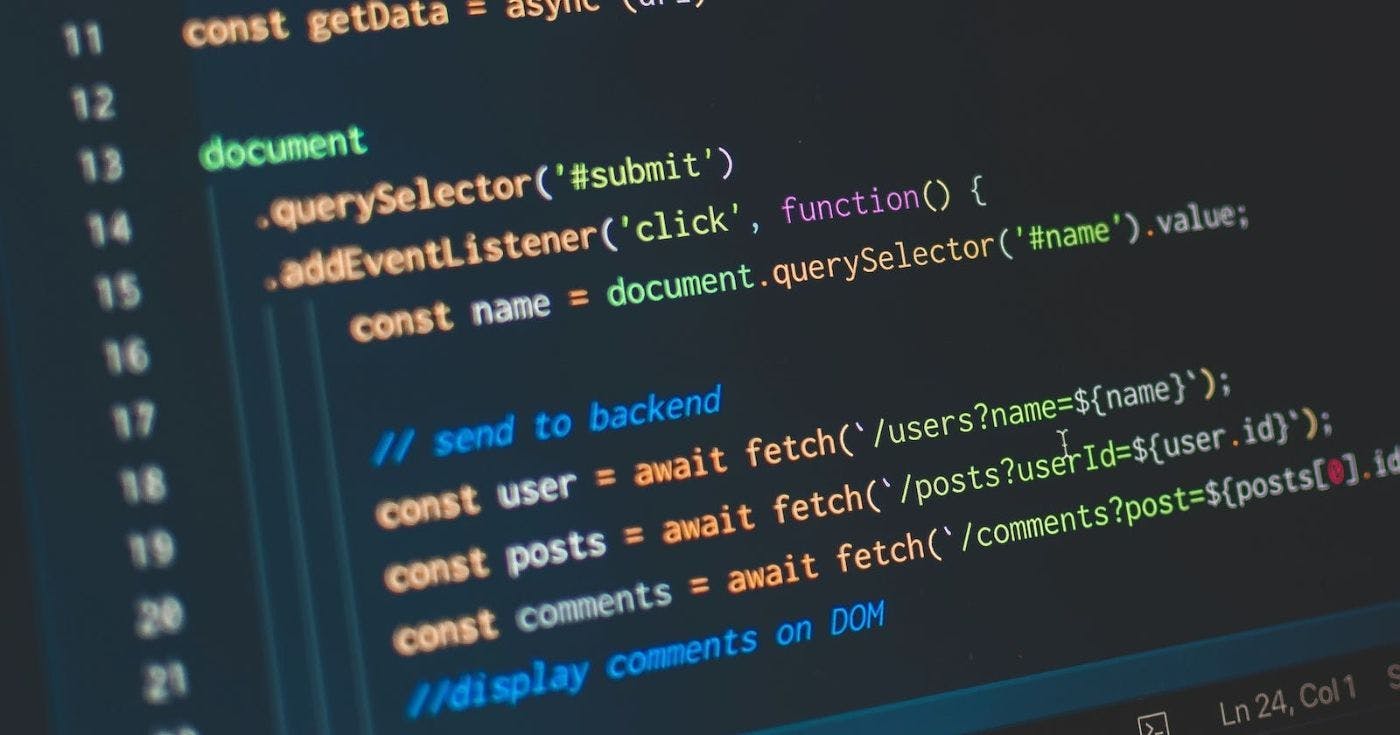
audio
element.JavaScript is an incredibly powerful and versatile programming language that has revolutionized the way websites and web applications are created. In this article, we'll explore the fundamentals of JavaScript and how it can be used to create dynamic, interactive web pages. We'll also discuss the history of JavaScript, its impact on web development, and some of the basic concepts to help you get started. So, let's dive in and start learning about this exciting language!
A Brief History of JavaScript
In 1993, Mosaic, the first web browser with a GUI (Graphical User Interface), was released and made available to non-technical people. This played a very Instrumental role in the growth of the embryonic World Wide Web and the Internet in general. After its success, the lead developer of Mosaic founded the Netscape Corporation, and a more refined version- The Netscape Navigator, was released in 1994.
Initially, web pages of this time could only be static, so there was a need to remove this limitation and build dynamic handling web pages. Netscape decided to add a scripting language to their browser to solve this problem. Brendan Eich, an American computer programmer, was initially hired by Netscape to embed the Scheme language. Eventually, It was decided that it would be a better option for Eich to devise a new scripting language, similar to Java but less like Scheme.
In September 1995, LiveScript was shipped as part of the Navigator beta. The name was later changed to JavaScript in December 1995 as part of a marketing ploy, as Java was one of the most popular languages then.
What is JavaScript?
JavaScript is a high-level, dynamically typed, prototype-based programming language. Let's break down this definition:
-
High-level language: This means it allows you to write programs in a more user-friendly context which ultimately gives abstraction from the complex details of the architecture of the computer.
-
Prototype-based: According to Mozilla's documentation, Prototype-based programming is a style of object-oriented programming in which classes are not explicitly defined but rather derived by adding properties and methods to an instance of another class or, less frequently, adding them to an empty object. In simple terms, it allows the creation of objects without first defining their class. The JavaScript Object prototype contains an extensive list of properties that you can explore here.
-
Dynamically typed: A dynamically typed language is one where the Interpreter assigns the variable a type at runtime depending on the content of the variable at that particular time.
Now that we have an understanding of what JavaScript is, let us explore what it does.
What Can I Do With JavaScript?
JavaScript can be used in two ways:
-
Client-side logic: JavaScript accounts for about 98% of client-side(Frontend) logic for websites worldwide. This is simply because it plays the role of "Frontend brain" almost perfectly, working alongside HTML (website markup) and CSS for styling to produce dynamic sites and Improve user experience. Modern websites have something called the DOM (Document Object Model).
The DOM is a representation of objects that comprise the elements of a website as nodes and objects. The DOM is made available as a programming interface, and JavaScript interacts with this interface to manipulate what we see on the website; this is how websites are made dynamic.
-
Server-side programming: Modern websites need to display their data dynamically. Because of this, they need a way to communicate with the server. They achieve this by using HTTP requests. They are predefined methods in JavaScript that you can use to retrieve, insert or delete data from a server. Writing Server-side code in JavaScript is regarded to be easier because dynamic sites tend to perform a lot of similar operations, but it is important to note that the more dynamic a site is, the more complex the requests become.
Now that we understand what JavaScript is, let us briefly explore some concepts and paradigms it entails.
JavaScript Concepts
- OOP (Object Oriented Programming): An object is a data field that stores its attributes. These attributes are stored in key-value pairs. These values could be functions, and in that case, they are called methods. Therefore object-oriented programming (OOP) is an approach in programming where data is stored in objects, and the object itself is operated on rather than having abstract functional components.
Data Types: There are seven different primitive data types in JavaScript. A primitive data type is one that has no properties or methods. data types are simply a way of holding values that would be later assigned to variables and/or functions for them to be worked on.
The primitive data types in JavaScript are:
-
Strings e.g
let str = "boy";
-
Numbers e.g
let num =9;
-
Booleans - booleans can either be
true
orfalse
e.glet isHungry = true;
-
Undefined
-
BigInt - Is used to store integers with arbitrary magnitude e.g
const x = BigInt(Number.MAX_SAFE_INTEGER);
-
Symbols e.g
$, #, / etc
you can learn more about these data types here.
-
Array methods: An array is an ordered collection of variables. Different data types can be stored in an array, whether they be primitive, objects, or even other arrays.
let myArray = ["Bob", 1, {boy: "John"}, [1, 2, 3]]
Each item in an array is given a numeric index with which they can be used to identify and manipulate. It is important to note that the numeric Index of an array always starts with
0
. In the example below, the Items in the array have an Index of0
,1
,2
respectively.let myArr = ["John", "Doe", "Stan"]; console.log(myArr[2]); //Expected Output //Stan
Array methods are simply the functions used to manipulate an array. Some of them are:
-
Push() - Insert an Item to the end of an array.
-
Pop() - Remove the last item in an array.
-
Unshift() - Add an element to the beginning of an array.
-
Shift() - Remove an element from the beginning of an array.
-
Slice() - Create a copy of an array.
-
Reverse() - Reverse the Items in an array.
-
Concat() - Merges one or more arrays and returns a new array.
-
Join() - Joins all elements in an array using a separator and returns a string. The default separator is a comma i.e
,
-
You can learn more about array methods here.
- DOM: The Mozilla documentation describes the DOM as an API that represents and interacts with any HTML document. In other words, it is a model that represents the HTML document as nodes in the browser. These nodes can then be changed or manipulated by Javascript in order to add dynamic content to the website. You should read more about the DOM here.
Diagram of the DOM
-
Asynchronous Programming: Asynchronous programming allows your program to run a potentially long task while still being able to respond to other events while that task runs. This is an important concept because some operations run on websites can potentially take a long amount of time hence why they need to be asynchronous.
Examples of these are:
-
HTTP requests using
fetch()
. -
Sending an email also uses
fetch()
. -
Asking users for file access using
showOpenFilePicker()
.
-
You can learn more about asynchronous programming here.
-
Promise: Promises in JavaScript are a way of handling asynchronous actions. It is best described as a proxy or a stand-in for a value not necessarily known when the promise was made. The eventual state of a promise can either be fulfilled or rejected. It will be fulfilled when the operation is successful or rejected when the operation encounters an error.
let fetchData = fetch('https://imaginary-random-api.json'); console.log(fetchData); //'https://imaginary-random-api.json' is not a real API fetchData.then((response) => { console.log(`Received response: ${response.status}`); }); console.log('Started Process...')
When the code above is run, this is what happens:
-
We call the
fetch()
API and assign it to a variablefetchData
. -
We then log
fetchData
to the console. -
The Promise's
then()
is used to catch the response. -
We log a message Indicating we have started.
-
the output should be:
//Promise { <state>: "pending" }
//Started Process…
//Received response: 200
The Initial state of a promise is pending hence why the Initial output indicates that*.* The started process...
seems to have been logged before the response. This is because fetch()
returns while the request is still ongoing. The diagram below shows the life cycle of a promise
- Apis: API is an acronym for Application Programming Interface, and it is an intermediary that allows two applications to interact and exchange data with each other. This is how organizations share data, and they exist in every application we use today, from a weather app to a ride-sharing app to even social media applications APIs are all around us.
Conclusion
In conclusion, coding in JavaScript can get confusing, but as far as you ground yourself with the basic concepts and paradigms, the journey to becoming a great developer loses a lot of potholes. You should check out Mozilla's Documentation and freecodecamp.org's courses to get more information.
Thanks for reading!
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK