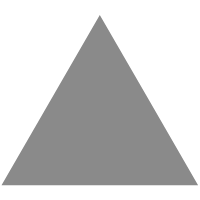
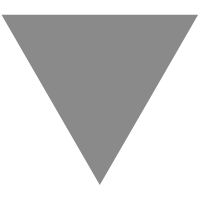
So You Think You Know For Loops?
source link: https://dev.to/travis_codez/so-you-think-you-know-for-loops-1k31
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
So You Think You Know For Loops?
So you've been learning JavaScript for a while; perhaps you've written several programs and solved dozens of problems on Code Wars? Or maybe you're just working your way through some tutorials and courses, or even just starting to get your feet a little wet? I assure you, if you've been writing all of your for loops like this:
for (let i = 0; i < n; i++) {
}
you're only scratching the surface of the for loops potential.
During my coding journey, I have discovered the weight room to have the greatest philosophical carryover to programming. Nobody in the weight room would believe they have a complete bench by exclusively doing flat barbell bench. In the weight room, you learn to hit the muscle from different angles and develop strength across several different planes of motion. Then yet, in programming, so many tutorials and resources only teach from a one dimensional perspective, the programming equivalent of exclusively doing flat barbell bench. Is it any wonder people get stuck in tutorial hell, when the tutorials do not approach learning from different angles and perspectives? That's why I think it's important to approach the for loop from an entirely different angle, to develop perspective on its true potential, and to develop a deeper understanding of how JavaScript works.
I took inspiration for this post from the Chapter 5 of the book Eloquent JavaScript, by Marijin Haverbeke. It helped me see for loops in an entirely different perspective, one that I have yet to see in any single JavaScript tutorial or course yet. And I'm going to break it down in greater detail than the book itself. So grab your favourite beverage and hang on tight, this one's going to take you for a loop!
The for loop in this example takes a starting value, a test function (conditional expression) that if true, calls the body function and passes the current iterated value (the value of i) to the body function, which subsequently calls the update function with the value of i, updating the loop value. In text alone, this sounds quite convoluted and confusing, so let's take a look at the code.
`const loop = (value, testFunction, updateFunction, bodyFunction) => {
for (let i = value; testFunction(i); i =updateFunction(i)){
bodyFunction(i)
}
}
loop(3, n => n > 0; n => n - 1, console.log)
`
To understand what is going on here, we need to take a look at the arguments being passed into the loop function. The value being passed in is 3, n => n > 0 = testFunction, n - 1 = updateFunction, and console.log = bodyFunction. This would commonly be written out as:
`for (let i = 3; i > 0; i--) {
console.log(i)
But if it was written out like this, it would miss out on the power of JavaScript: you can pass values directly as arguments to a function call without having to declare them first as variables! This allows for more refined and customized control of the loop via callback functions! While in most use cases, using a traditionally instantiated iterator (let i = insert value) would be the best case, there may be use cases where one would want to control the loops behaviour via callback functions. By passing in values directly as function calls, it allows to write code more adherent to DRY principles, and thereby eliminates repetition if the values had to be declared elsewhere with let or const. Furthermore, this example demonstrates the power and flexibility of Javascript that is never shown in tutorials or courses when teaching loops. To write good code adherent to functional programming standards, understanding how functions and values can be passed as arguments is key, especially when chaining together several higher order functions, which is a common practice in the React framework.
So take a moment and experiment with the code example, change the values, and dig deeper to develop a greater understanding of the for loop. I encourage you to try and solve other problems by writing for loops in this manner, it will pay dividends later in your coding journey when you write more callback functions to solve problems.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK