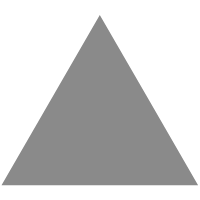
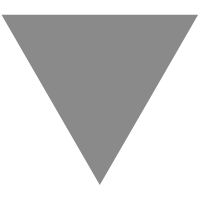
How to write tests with Fabric8 Kubernetes Client
source link: https://developers.redhat.com/articles/2023/01/24/how-write-tests-fabric8-kubernetes-client
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
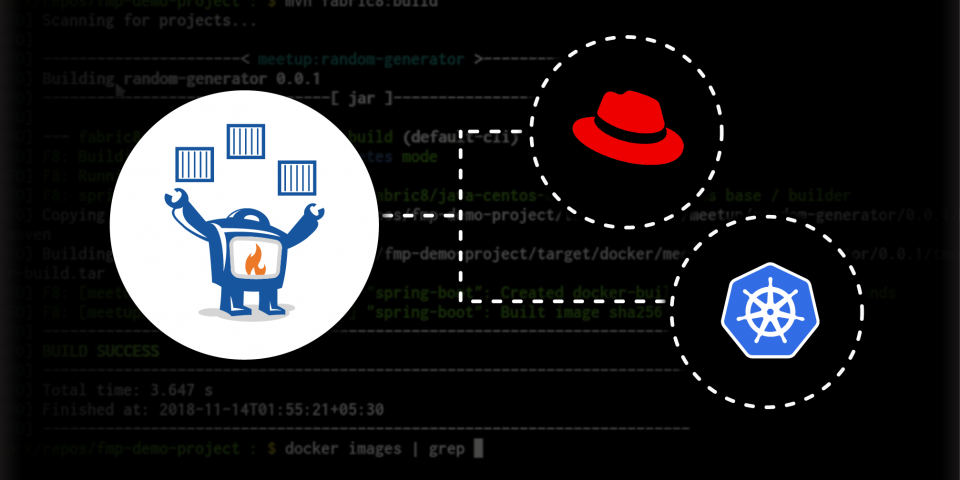
Like regular applications, it is essential to write tests while building applications that interact with the Kubernetes API server (i.e., Kubernetes Operators). However, it is not always possible to have a Kubernetes test environment available for running tests. Also, our tests may require us to satisfy certain prerequisites (some specific Kubernetes version) in order to run successfully.
In this blog post, we will look at testing libraries made available by Fabric8 Kubernetes Client and focus mainly on Fabric8 Kubernetes Mock Server and Fabric8 JUnit5 Extension.
This article is the final installment in my series:
How to write tests using Fabric8 Kubernetes Mock Server
How to write tests using Fabric8 Kubernetes Mock Server
Since it’s not always possible to have a Kubernetes Cluster available for testing while writing tests, most developers try to mock KubernetesClient calls using mocking frameworks like Mockito and JMockit. While this can work for most scenarios, overuse of mocking nested KubernetesClient calls can leave tests cluttered with mock calls and decrease readability.
Fabric8 Kubernetes Client provides a Kubernetes Mock Server that provides spins up a server during testing that looks very much like a real Kubernetes API server. Based on OkHttp’s MockWebServer, it tries to emulate the Kubernetes API server’s calls for common operations such as get, list, create, watch, etc., which can be common scenarios while testing Kubernetes applications.
In order to use Kubernetes Mock Server, you need to add the following dependency:
<dependency>
<groupId>io.fabric8</groupId>
<artifactId>kubernetes-server-mock</artifactId>
<version>${fabric8.version}</version>
<scope>test</scope>
</dependency>
Once added as a dependency, you can start using Kubernetes Mock Server in your tests. Let’s see how we can get started with using Kubernetes Mock Server in our tests.
If you’re using JUnit4, you can add a JUnit Rule for Kubernetes Mock Server in your test as follows:
import io.fabric8.kubernetes.client.server.mock.KubernetesServer;
public class Foo {
@Rule
public KubernetesServer crudServer = new KubernetesServer(true, true);
// …
}
There are two configurable arguments when initializing Kubernetes Mock Server:
- Crud: Enable Opinionated Kubernetes mock server, where users don’t need to provide expectations for their operations (defaults to false).
- Https: Use HTTPS or not (defaults to true).
If you’re using JUnit5, you can use the following @EnableKubernetesMockClient annotation:
import io.fabric8.kubernetes.client.server.mock.EnableKubernetesMockClient;
@EnableKubernetesMockClient
class Foo {
private KubernetesClient kubernetesClient;
private KubernetesMockServer server;
// ..
}
You can use Kubernetes Mock Server in two modes:
- CRUD Mode: Opinionated Kubernetes Mock Server acts very similar to a real Kubernetes API Server and processes common operations such as get, create, watch, etc.
- Expectations Mode: The user defines expectations for Kubernetes Mock Server for API endpoints expected to hit during test execution and defines response elements.
I will explain both modes with the help of an example.
Consider an elementary class PodGroupService that manages a group of pods in the currently logged namespace sharing some labels.
Refer to my Kubernetes Client demo PodGroupService.java.
package io.fabric8.demos.tests.mockserver;
import io.fabric8.kubernetes.client.KubernetesClient;
import java.util.Map;
public class PodGroupService {
private final KubernetesClient kubernetesClient;
private final Map<String, String> matchLabels;
public PodGroupService(KubernetesClient client, Map<String, String> matchLabels) {
this.kubernetesClient = client;
this.matchLabels = matchLabels;
}
public PodList list() {
return kubernetesClient.pods().withLabels(matchLabels).list();
}
public int size() {
return list().getItems().size();
}
// Rest of methods
}
Next, let’s try to write a test for this size method using Kubernetes Mock Server using both modes.
CRUD mode
In CRUD mode, you don’t need to set any expectations. You need to use KubernetesClient assuming a real Kubernetes API server is running in the background. You can see it’s quite transparent and readable:
Refer to PodGroupServiceCrudTest.java.
package io.fabric8.demos.tests.mockserver;
import io.fabric8.kubernetes.api.model.PodBuilder;
import io.fabric8.kubernetes.client.KubernetesClient;
import io.fabric8.kubernetes.client.server.mock.EnableKubernetesMockClient;
import io.fabric8.kubernetes.client.server.mock.KubernetesMockServer;
import org.junit.jupiter.api.Test;
import java.util.Collections;
import static org.junit.jupiter.api.Assertions.assertEquals;
@EnableKubernetesMockClient(crud = true)
class PodGroupServiceCrudTest {
private KubernetesClient kubernetesClient;
private KubernetesMockServer server;
@Test
void size_whenPodsWithLabelPresent_thenReturnCount() {
// Given
Map<String, String> matchLabel = Collections.singletonMap("foo", "bar");
kubernetesClient.pods().resource(createNewPod("p1", matchLabel)).create();
PodGroupService podGroupService = new PodGroupService(kubernetesClient, matchLabel);
// When
int count = podGroupService.size();
// Then
assertEquals(1, count);
}
}
Expectations mode
While in Expectations mode, we need to set up expectations for the behavior we want when a certain Kubernetes resource endpoint the KubernetesClient requests:
Refer to the PodGroupServiceTest.java.
package io.fabric8.demos.tests.mockserver;
import io.fabric8.kubernetes.api.model.PodBuilder;
import io.fabric8.kubernetes.api.model.PodListBuilder;
import io.fabric8.kubernetes.client.KubernetesClient;
import io.fabric8.kubernetes.client.server.mock.EnableKubernetesMockClient;
import io.fabric8.kubernetes.client.server.mock.KubernetesMockServer;
import org.junit.jupiter.api.Test;
import java.util.Collections;
import static java.net.HttpURLConnection.HTTP_OK;
import static org.junit.jupiter.api.Assertions.assertEquals;
@EnableKubernetesMockClient
class PodGroupServiceTest {
private KubernetesClient kubernetesClient;
private KubernetesMockServer server;
@Test
void size_whenPodsWithLabelPresent_thenReturnCount() {
// Given
server.expect().get()
.withPath("/api/v1/namespaces/test/pods?labelSelector=foo%3Dbar")
.andReturn(HTTP_OK, new PodListBuilder().addToItems(
new PodBuilder()
.withNewMetadata()
.withName("pod1")
.addToLabels("foo", "bar")
.endMetadata()
.build())
.build())
.once();
PodGroupService podGroupService = new PodGroupService(kubernetesClient, Collections.singletonMap("foo", "bar"));
// When
int count = podGroupService.size();
// Then
assertEquals(1, count);
}
}
Writing tests against real Kubernetes clusters
Writing tests against real Kubernetes clusters
Apart from Kubernetes Mock Server, Fabric8 Kubernetes Client provides a set of JUnit5 extension annotations that can simplify writing tests against a real Kubernetes Cluster.
To use these JUnit5 annotations, you need to add this dependency:
<dependency>
<groupId>io.fabric8</groupId>
<artifactId>kubernetes-junit-jupiter</artifactId>
<version>${fabric8.version}</version>
<scope>test</scope>
</dependency>
The following table lists the annotations provided by this dependency:
Name |
Description |
|
Creates a temporary test namespace and configures a KubernetesClient instance in the test class to use in the tests. |
|
Apply a YAML file to set up the environment before test execution. See example. |
|
Only execute the test when a specific Kubernetes resource is present in the Kubernetes cluster before test execution. It’s quite helpful in the case of custom resources. See example. |
|
Only execute test when Kubernetes version is at least specified version. See example. |
Now let’s come back to our PodGroupService example and try to write an end-to-end test for the size()
method. You can see it’s very similar to the test we wrote for Kubernetes Mock Server in CRUD mode, but here we’re using the JUnit5 annotations: PodGroupServiceIT.java.
package io.fabric8.demos.tests.e2e;
// …
import io.fabric8.junit.jupiter.api.KubernetesTest;
import io.fabric8.junit.jupiter.api.RequireK8sSupport;
import io.fabric8.junit.jupiter.api.RequireK8sVersionAtLeast;
@KubernetesTest
@RequireK8sSupport(Pod.class)
@RequireK8sVersionAtLeast(majorVersion = 1, minorVersion = 16)
class PodGroupServiceIT {
KubernetesClient kubernetesClient;
@Test
void size_whenPodsPresent_thenReturnActualSize() {
// Given
PodGroupService podGroupService = new PodGroupService(kubernetesClient, Collections.singletonMap("app", "size-non-zero"));
podGroupService.addToGroup(createNewPod("p1", "size-non-zero"));
podGroupService.addToGroup(createNewPod("p2", "size-non-zero"));
// When
int result = podGroupService.size();
// Then
assertEquals(2, result);
}
}
Fabric8 Kubernetes series wrap-up
Fabric8 Kubernetes series wrap-up
This concludes my series on Fabric8 Kubernetes Java for developers. This article demonstrated how to write unit and end-to-end tests using testing libraries provided by Fabric8 Kubernetes Client. You can find the code in this GitHub repository.
For more information, check out the Fabric8 Kubernetes Client GitHub page. Feel free to follow us on these channels:
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK