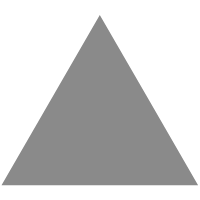
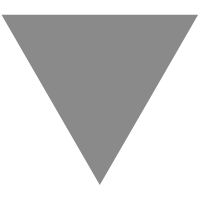
golang 递归判断回文字符串
source link: https://studygolang.com/articles/3147?fr=sidebar
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
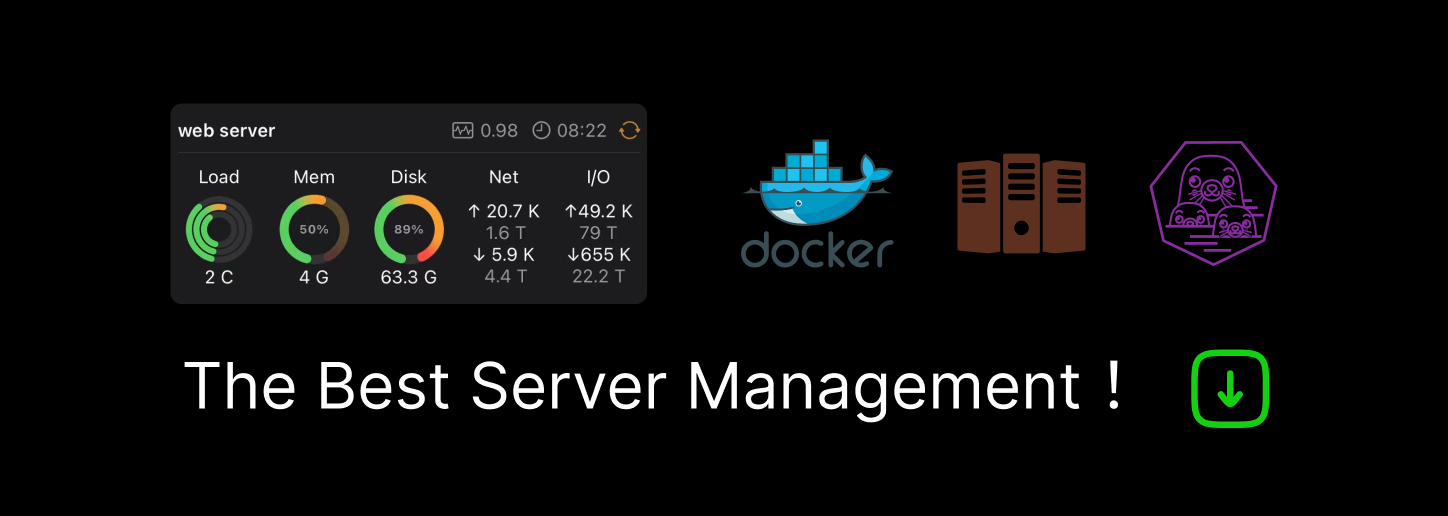
golang 递归判断回文字符串
guonaihong · 2015-05-25 23:00:01 · 3501 次点击 · 预计阅读时间 2 分钟 · 大约8小时之前 开始浏览判断回文字符串是个比较经典的问题。
思路就是拿第一个字符和最一个字符比较,如果不等退出,相同的话继续刚刚的过程,直到第一个字符和最后一个字符相遇或者他们的距离为1时。说明他们是回文字符串。
下面的代码会忽略空白字符 如"1 1 2 1"会让为是回文字符串。
golang
package main import ( "fmt" "os" "strings" "unicode/utf8" ) func doPalindrome(s string) bool { if utf8.RuneCountInString(s) <= 1 { return true } word := strings.Trim(s, "\t \r\n\v") first, sizeOfFirst := utf8.DecodeRuneInString(word) last, sizeOfLast := utf8.DecodeLastRuneInString(word) if first != last { return false } return doPalindrome(word[sizeOfFirst : len(word)-sizeOfLast]) } func IsPalindrome(word string) bool { s := "" s = strings.Trim(word, "\t \r\n\v") if len(s) == 0 || len(s) == 1 { return false } return doPalindrome(s) } func main() { args := os.Args[1:] for _, v := range args { ok := IsPalindrome(v) if ok { fmt.Printf("%s\n", v) } } }
clang 递归版:
#include <stdio.h> #include <string.h> #include <ctype.h> #include <stdint.h> int do_palind(char *first, char *last) { /*跳过头部的空白字符*/ while(*first && isspace(*first)) { first++; } /*跳过尾部的空白字符*/ while (first < last && isspace(*last)) { last--; } if (last - first <= 0) return 1; if (*first != *last) return 0; return do_palind(++first, --last); } int ispalindrome(const char *str) { if (str[0] == '\0' || str[1] == '\0') return 0; //printf("---->%ld\n", strlen(str)); return do_palind((char *)str, (char *)str + strlen(str) - 1); } int main(int argc, char **argv) { int is; while (*++argv) { is = ispalindrome(*argv); if (is) printf("%s\n", *argv); } }
clang 循环版:
#include <stdio.h> #include <string.h> #include <ctype.h> int ispalindrome(const char *str) { char *last; if (str[0] == '\0' || str[1] == '\0') return 0; last = (char *)str + strlen(str) - 1; while (str < last) { while (str < last && isspace(*str)) { str++; } while (str < last && isspace(*last)) { last--; } if (*str != *last) return 0; str++; last--; } return 1; } int main(int argc, char **argv) { int is; while (*++argv) { is = ispalindrome(*argv); if (is) { printf("%s\n", *argv); } } }
有疑问加站长微信联系(非本文作者)

Recommend
-
36
刷力扣的时候, 碰到了一个找最长回文字符串的题, 解开了, 但只要提交就提示超时, 然后就开始想办法优化, 下面说一下我处理的方法 有兴...
-
26
我这天天写核心业务的人,都没用什么算法啊!其实算法无处不在,栈队列树链表都包含算法思想,算法并不是单纯指用代码解决那些深奥难懂的数学逻辑问题,而是代码中的普适化思维。并且算法也不可怕,是基本功,就像足球中的体能训练,微软...
-
33
题目: 给定一个字符串 s ,找到 s 中最长的回文子串。你可以假设 s 的最大长度为 1000。 思路: 看到这道题目,首先想到回文字符串,是一个沿正中字...
-
10
如何高效判断回文链表 👆让天下没有难刷的算法!若 GitBook 访问太慢,可尝试
-
4
【面试现场】如何找到字符串中的最长回文子串?
-
6
golang判断字符串出现的位置及是否包含 次序 · 2018-10-30 07:34:40 · 25919 次点击 · 预计阅读时间 1 分钟 · 大约8小时之前 开始浏览
-
4
【leetcode】680.验证回文字符串Ⅱ 2020-03-07 链接:https://leetcode-cn.com/problems/valid-palindrome-ii 给定一个非空字符串 s,最多删除一个...
-
6
统计字符串中不同回文子序列的个数 作者:Grey 原文地址: ...
-
4
如何判断回文链表
-
8
2023-07-31:用r、e、d三种字符,拼出一个回文子串数量等于x的字符串。 1 <= x <= 10^5。 来自百度。 精选 原创
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK