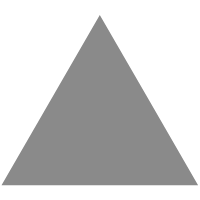
4
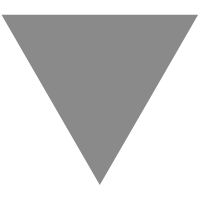
Generate Docusaurus Docs from JSDoc · GitHub
source link: https://gist.github.com/slorber/0bf8c8c8001505f0f99a062ac55bf442
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Generate Docusaurus Docs from JSDoc · GitHub
Instantly share code, notes, and snippets.
Generate Docusaurus Docs from JSDoc
I needed something a little smaller than this for a single package.
const fs = require('fs')
const path = require('path')
const glob = require('glob')
const { execSync } = require('child_process');
/**
* Runs through packages folders looking for JSDoc and generates markdown docs
*/
function generateDocs() {
console.log('Generating package docs')
// Use glob to get all js/ts files
const pathPattern = path.join(__dirname, './lib/**/*.[jt]s?(x)')
const filePaths = glob.sync(pathPattern, {
ignore: [
'**/node_modules/**',
'**/cypress/**',
'**/__tests__/**',
'**/*.test.js',
'**/*_spec.js',
],
})
console.log(filePaths)
for(const file of filePaths) {
const { base: fileName } = path.parse(file);
const relativePath = path.relative(process.cwd(), file);
const markdown = execSync(`./node_modules/.bin/jsdoc2md ${relativePath}`);
const writeDir = path.join(
__dirname,
`website/docs/api/${relativePath.replace(fileName, '')}`,
)
// check if the directory exists
if (!fs.existsSync(writeDir)) {
// create the directory
fs.mkdirSync(writeDir, { recursive: true })
}
// write the markdown file
fs.writeFileSync(`${writeDir}/${fileName}.md`, markdown)
}
// Let the user know what step we're on
console.log('\u001B[32m', '✔️ Package docs generated', '\u001B[0m')
}
generateDocs()
process.exit(0)
This worked for me. Thanks for the initial work @slorber !
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK