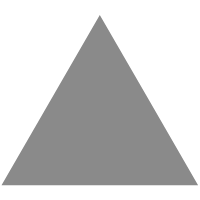
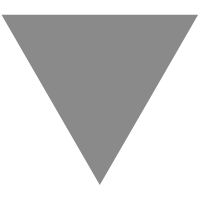
Why You Should Avoid Mutating or Reassigning Props in Vue
source link: https://blog.bitsrc.io/why-you-should-avoid-mutating-or-reassigning-props-in-vue-ed25f27be88d
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
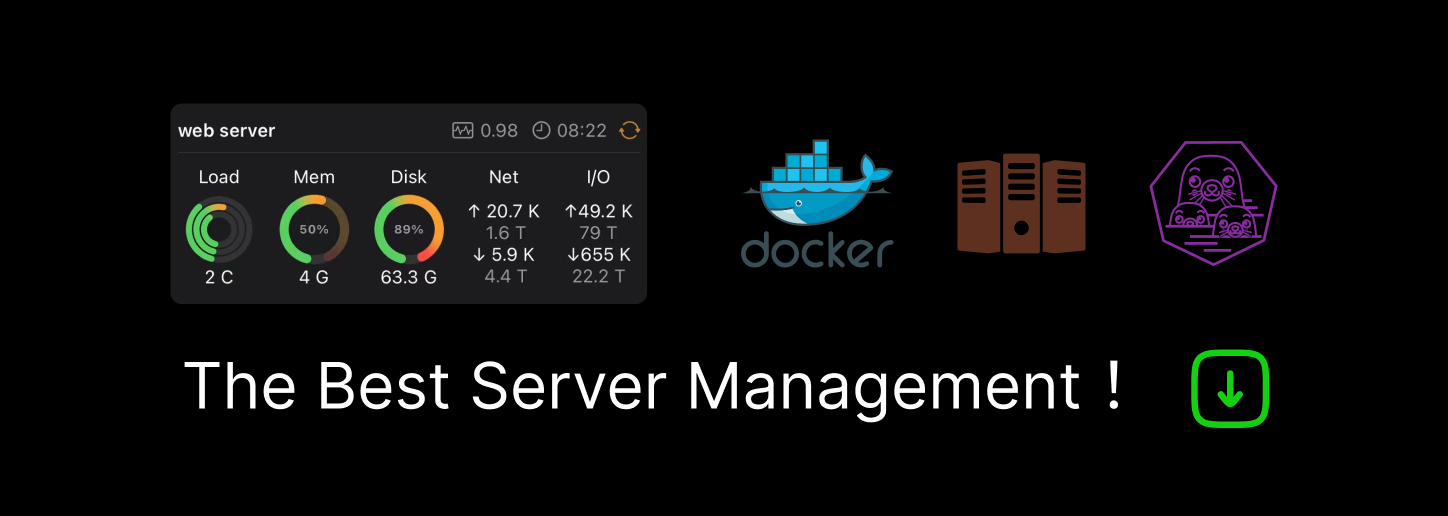
Why You Should Avoid Mutating or Reassigning Props in Vue

Image from LearnVue
Props are a powerful feature in Vue 3 that allows you to pass data from a parent component to a child component. They are a one-way flow of data, meaning that the parent component can pass data to the child, but the child cannot modify the data passed to it via props.
However, it can be tempting to mutate or reassign props within the child component, especially if you are used to working with other frameworks that allow two-way data binding. It’s important to resist this temptation, as mutating props in Vue 3 can lead to a number of issues.
Why Props Should Be Considered Immutable
There are a few reasons why you should not mutate props in Vue 3:
- Props are intended to be a one-way flow of data from the parent to the child. If the child were to mutate a prop, it would violate this one-way flow and create potential inconsistencies in the application.
- Mutating props can lead to unexpected behavior, as the parent component may not be aware that the prop has been modified. This can cause bugs that are difficult to track down and fix.
- In Vue 3, the reactivity system works by watching the data properties of a component and re-rendering the component when they change. If a prop is mutated, the reactivity system will not be able to detect the change, and the component will not re-render as expected.
A great example of the above example I’ve setup below:
As you can see in this example — The local name updates, but it doesn’t update in the parent component which is a massive problem as you are breaking reactivity, maybe unintentionally. The following will most likely also lead to unintended side-effects in your application.
How to Change Prop Values Correctly
So, if you can’t mutate props, how can you respond to changes in props within the child component? There are multiple ways on doing so, but I will show you the recommended solution.
Emit back changed value
One option for responding to prop changes in Vue 3 is to use the emit
function to emit an event back to the parent component. The parent component can then listen for this event and take appropriate action.
Here is an updated example of the Child & Parent component of using emit
to respond to a prop change in a child component:
Child.vue
Notice — I’ve removed the localName ref as it is not needed with the updated solution. See further down below in Parent.vue
<template>
<div>
{{ name }} <br />
<button @click="changeName"></button>
</div>
</template>
<script lang="ts">
import { defineComponent, ref } from 'vue';
export default defineComponent({
name: 'Child',
props: {
name: {
type: String,
required: true
}
},
setup(props, context) {
const changeName = () => {
context.emit('updateName', 'John Doe');
};
return {
changeName
};
}
});
</script>
<style scoped></style>
Parent.vue
<template>
<div>
Parent Name: {{ name }}
<Child :name="name" @updateName="setName" />
</div>
</template>
<script lang="ts">
import { defineComponent, ref } from 'vue';
import Child from '@/components/Child.vue';
export default defineComponent({
name: 'Parent',
components: { Child },
setup() {
const name = ref('Nicky');
const setName = (newName: string) => {
name.value = newName;
};
return {
setName,
name
};
}
});
</script>
In summary, while assigning props to a new ref()
and changing the prop value locally may seem convenient at first, but it can lead to performance and maintainability issues in your Vue 3 components — Also as you see in the example I set up in CodeSandbox, the reactivity breaks. When assigning a new value to the localName, the parent component does not get notified of the change. It is generally better to access props directly using the props
object in the component's setup function to avoid breaking reactivity and to make your component easier to maintain.
Recommend
-
65
-
158
readme.md
-
17
Guitar Decomposed: 5. Mutating the Third Posted by Bartosz Milewski under Music
-
10
Here’s a Plan to Stop the Coronavirus From MutatingPrioritize people who are immunocompromised for early vaccination.Illustration: WIRED Staff; Getty ImagesWhen the pa...
-
10
最近又写了一个 K8s 的 Mutating Webhook ,阅读了一下 官方文档 ,有些特殊需要记住的地摘记如下。虽然主要是针对 Mutating...
-
5
Why You Should Avoid Security NihilismDecember 10th 2021 new story8I love reading about cybersecu...
-
9
Why you should avoid the base model M2 MacBook Air News ...
-
5
C++ Program To Print Number without reassigningSkip to content
-
6
Why you should avoid import * in Python By Bob Belderbos on 15 August 2023 Anyone who’s worked with...
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK