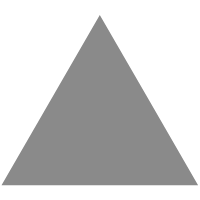
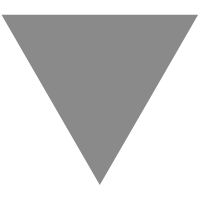
Upload And Dowload Object In SAP BTP Object Store On AWS With NodeJS
source link: https://blogs.sap.com/2022/12/29/upload-and-dowload-object-in-sap-btp-object-store-on-aws-with-nodejs/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Upload And Dowload Object In SAP BTP Object Store On AWS With NodeJS
In the tutorial Create Multi-Cloud Application Consuming Object Store Service , the method consuming Object Store Service on AWS with Java has been introduced in detail . In help portal
the method in Java also has been introduced.
In the blog Connecting to SAP BTP Object Store running on Microsoft Azure Platform using NodeJS
Ujala Kumar Panda has introduce use nodejs to consume BTP Object Store on Azure .
Today I want to introduce the methed with nodjs in a MTA application. I will use S3 Client – AWS SDK for JavaScript v3 to consume the service .
Prerequisite:
1, You have installed CF Client .
2, You have installed Nodejs .
3, You have installed Cloud MTA Build Tool .
4, You have installed VSCode (optional).
Steps :
Step 1: Configure Object Store to use Amazon Simple Storage Service .
You can following the document in help. The following are the screen shot :
As the result you will found the credential information and note it down .

Step 2: Generate SAPUI5 project with generator-saphanaacademy-mta

Open the application with visual code .

Step 3: Add package aws-sdk and formidable in package.json under srv .

Step 4: Change server.js under srv as the following
The following constant are from step 1 credential .
const express = require('express');
const app = express();
const fs = require('fs');
const bodyParser = require('body-parser');
const formidable = require('formidable');
const { S3Client, PutObjectCommand, ListObjectsCommand, GetObjectCommand } = require("@aws-sdk/client-s3");
const regionV = "eu-central-1";
const endPointV = "s3-eu-central-1.amazonaws.com";
const accessKeyIdV = "AKIA5PG";
const secretAccessKeyV = "2cj70kGQfrxZ";
const bucketV = "hcp-61ea54163b1be";
const xsenv = require('@sap/xsenv');
xsenv.loadEnv();
const services = xsenv.getServices({
uaa: { tag: 'xsuaa' }
});
const xssec = require('@sap/xssec');
const passport = require('passport');
passport.use('JWT', new xssec.JWTStrategy(services.uaa));
app.use(passport.initialize());
app.use(passport.authenticate('JWT', {
session: false
}));
function makeid(length) {
var result = '';
var characters = 'ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789';
var charactersLength = characters.length;
for (var i = 0; i < length; i++) {
result += characters.charAt(Math.floor(Math.random() * charactersLength));
}
return result;
}
app.use(bodyParser.json());
// app.use(bodyParser.urlencoded({ extended: true }));
app.get('/srv/s3', function (req, res) {
if (req.authInfo.checkScope('$XSAPPNAME.User')) {
const client = new S3Client({ region: regionV, endPoint: endPointV, credentials: { accessKeyId: accessKeyIdV, secretAccessKey: accessKeyIdV } });
var keyid = makeid(5).concat(".txt");
var content = makeid(5);
const params =
{
Bucket: bucketV, // The name of the bucket. For example, 'sample_bucket_101'.
Key: keyid, // The name of the object. For example, 'sample_upload.txt'.
Body: content // The content of the object. For example, 'Hello world!".
};
const putCommand = new PutObjectCommand(params);
client.send(putCommand).then(data => {
res.status(200).send(data);
}).catch(err => {
res.status(500).send(err);
});
} else {
res.status(403).send('Forbidden');
}
});
app.post('/srv/s3/upload', function (req, res) {
const form = new formidable.IncomingForm();
const client = new S3Client({ region: regionV, endPoint: endPointV, credentials: { accessKeyId: accessKeyIdV, secretAccessKey: secretAccessKeyV } });
const params =
{
Bucket: bucketV, // The name of the bucket. For example, 'sample_bucket_101'.
Key: "",
Body: ""
};
form.parse(req, function (err, fields, files) {
if (err) {
return res.status(400).json({ error: err.message });
}
const [firstFile] = Object.values(files);
params.Key = firstFile.originalFilename;
const file = fs.readFileSync(firstFile.filepath);
params.Body = file;
const putCommand = new PutObjectCommand(params);
client.send(putCommand).then(data => {
res.status(200).send(data);
}).catch(err => {
console.log(err);
res.status(500).send(err);
});
});
});
app.post('/srv/s3/download', function (req, res) {
const client = new S3Client({ region: regionV, endPoint: endPointV, credentials: { accessKeyId: accessKeyIdV, secretAccessKey: secretAccessKeyV } });
var keyV = req.body.key;
const params =
{
Bucket: bucketV,
Key: keyV,
};
const getCommand = new GetObjectCommand(params);
client.send(getCommand).then(data => {
console.log(data);
const chunks = []
const stream = data.Body;
stream.on('data', chunk => chunks.push(chunk));
stream.once('end', () => res.status(200).send(chunks));
}).catch(err => {
console.log(err.message);
res.status(500).send(err.message);
});
});
app.get('/srv/s3/list', function (req, res) {
if (req.authInfo.checkScope('$XSAPPNAME.User')) {
const client = new S3Client({ region: regionV, endPoint: endPointV, credentials: { accessKeyId: accessKeyIdV, secretAccessKey: secretAccessKeyV } });
const params =
{
Bucket: bucketV // The name of the bucket. For example, 'sample_bucket_101'.
};
const putCommand = new ListObjectsCommand(params);
client.send(putCommand).then(data => {
res.status(200).send(data);
}).catch(err => {
res.status(500).send(err);
});
} else {
res.status(403).send('Forbidden');
}
});
app.get('/srv', function (req, res) {
if (req.authInfo.checkScope('$XSAPPNAME.User')) {
res.status(200).send('objstore');
} else {
res.status(403).send('Forbidden');
}
});
app.get('/srv/user', function (req, res) {
if (req.authInfo.checkScope('$XSAPPNAME.User')) {
res.status(200).json(req.user);
} else {
res.status(403).send('Forbidden');
}
});
const port = process.env.PORT || 5001;
app.listen(port, function () {
console.info('Listening on http://localhost:' + port);
});
Step 5: Adjust index.html under app/resources .

Step 6: Build and deploy the project to BTP cloud foundry with follwoing command:
mbt build
cf deploy mta_archives\objstore_0.0.1.mtar
Step 7: Test with browser:



Step 8: Test with postman with :
You can get the oauth2 information from the screen after deployment.


With oauth2 information you can test the download and upload function .

The End!
Thanks for your time!
Best regards!
Jacky Liu
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK