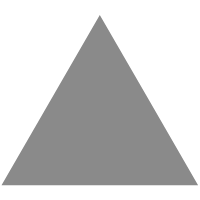
5
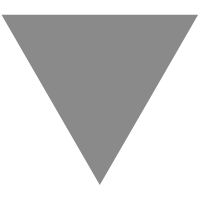
Java如何测量方法执行时间
source link: https://www.pkslow.com/archives/java-elapsed-time
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
为了知道调用方法用了多长时间,我们需要测量一下方法的执行时间。废话少说,直接给出四种方法。
JDK currentTimeMillis
先定义一个call
方法,我们来测量这个方法的执行时间:
private static void call() {
try {
Thread.sleep(500);
} catch (InterruptedException e) {
throw new RuntimeException(e);
}
}
使用JDK方法:
private static void currentTimeMillis() {
long start = System.currentTimeMillis();
call();
long end = System.currentTimeMillis();
long elapsed = end - start;
System.out.println(elapsed);
}
JDK nanoTime
使用JDK方法:
private static void nanoTime() {
long start = System.nanoTime();
call();
long end = System.nanoTime();
long elapsed = end - start;
elapsed = elapsed / 1000000;
System.out.println(elapsed);
}
Java8 Time Instant
使用Java 8的方法:
private static void java8TimeInstant() {
Instant start = Instant.now();
call();
Instant end = Instant.now();
long elapsed = Duration.between(start, end).toMillis();
System.out.println(elapsed);
}
commons lang StopWatch
使用commons-lang3
库的方法,先引入库:
<dependency>
<groupId>org.apache.commons</groupId>
<artifactId>commons-lang3</artifactId>
<version>3.12.0</version>
</dependency>
private static void stopWatch() {
StopWatch w = new StopWatch();
w.start();
call();
w.stop();
System.out.println(w.getTime());
}
基本一样,但currentTimeMillis容易有误差,精确的测量不建议使用:
currentTimeMillis: 501
nanoTime: 500
java8TimeInstant: 500
stopWatch: 500
代码请看GitHub: https://github.com/LarryDpk/pkslow-samples
Code for all: GitHub
欢迎关注微信公众号<南瓜慢说>,将持续为你更新...
Recommendations:
Cloud Native
Terraform
Container: Docker/Kubernetes
Spring Boot / Spring Cloud
Https
如何制定切实可行的计划并好好执行
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK