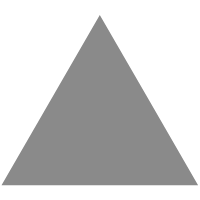
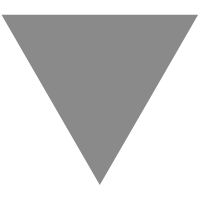
GitHub - mrkuz/kradle: Swiss army knife for Kotlin/JVM (and also Java) developme...
source link: https://github.com/mrkuz/kradle
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Kradle
Swiss army knife for Kotlin/JVM (and also Java) development.
kradle
is a Gradle plugin, which sets up your Kotlin/JVM (or Java) project in no time.
With a few lines of configuration, you will be able to:
Most of the functionality is provided by other well-known plugins. kradle
takes care of the setup and provides a
unified configuration DSL.
Table of contents
What's new?
See CHANGELOG.
(Very) Quick Start
Kotlin:
mkdir demo && cd demo
curl -O https://raw.githubusercontent.com/mrkuz/kradle/main/examples/kotlin/app/settings.gradle.kts
curl -O https://raw.githubusercontent.com/mrkuz/kradle/main/examples/kotlin/app/build.gradle.kts
gradle bootstrap
Java:
mkdir demo && cd demo
curl -O https://raw.githubusercontent.com/mrkuz/kradle/main/examples/java/app/settings.gradle.kts
curl -O https://raw.githubusercontent.com/mrkuz/kradle/main/examples/java/app/build.gradle.kts
gradle bootstrap
Run application:
./gradlew run
Package application and run JAR:
./gradlew uberJar && java -jar build/libs/demo-1.0.0-uber.jar
Build Docker image and run container:
./gradlew buildImage && docker run --rm demo
Quick Start
Add the kradle
plugin to your build script: net.bitsandbobs.kradle
.
build.gradle.kts
plugins {
id("org.jetbrains.kotlin.jvm") version "1.7.20"
id("net.bitsandbobs.kradle") version "main-SNAPSHOT"
}
group = "com.example"
version = "1.0.0"
kradle {
kotlinJvmApplication {
jvm {
application {
mainClass("com.example.demo.AppKt")
}
}
}
}
Make sure you apply the Kotlin plugin before kradle
. For applications, you have to provide the mainClass
.
If you are starting from scratch, you can run gradle boostrap
to initialize Git, add Gradle wrapper and create
essential directories and files.
The example above uses the Kotlin/JVM application preset.
Check the configuration reference to see all available options.
For Java projects apply the Java plugin instead of the Kotlin plugin.
Tasks
Which tasks are available, depends on the features enabled.
Task | Description | Alias for | Plugins used |
---|---|---|---|
bootstrap | Bootstraps app/lib project | - | - |
showDependencyUpdates | Displays dependency updates | - | Gradle Versions Plugin |
lint | Runs ktlint (Kotlin) and checkstyle (Java) | - | ktlint Plugin, Checkstyle Plugin |
analyzeCode | Runs detekt (Kotlin), PMD (Java) and SpotBugs (Java) code analysis | - | detekt Plugin, PMD Plugin, SpotBugs Plugin |
analyzeDependencies | Analyzes dependencies for vulnerabilities | - | OWASP Dependency Check Plugin |
dev | Runs the application and stops it when sources change (use with -t , applications only) |
- | - |
runBenchmarks | Runs JMH benchmarks | benchmark | kotlinx.benchmark Plugin |
integrationTest | Runs integration tests | - | - |
functionalTest | Runs functional tests | - | - |
runTests | Runs all tests | - | - |
analyzeTestCoverage | Runs test coverage analysis | - | Kover, JaCocCo Plugin |
generateDocumentation | Generates Dokka HTML documentation | - | Dokka Plugin |
package | Creates JAR | jar | Java Plugin |
uberJar | Creates Uber-JAR (applications only) | - | Gradle Shadow Plugin |
buildImage | Builds Docker image (applications only) | - | Jib Plugin |
pushImage | Pushes container image to remote registry (applications only) | - | Jib Plugin |
install | Installs JAR to local Maven repository (libraries only) | publishToMavenLocal | Maven Publish Plugin |
generateGitignore | Generates .gitignore | - | - |
generateBuildProperties | Generates build.properties | - | - |
generateDetektConfig | Generates detekt-config.yml | - | - |
generateCheckstyleConfig | Generates checkstyle.xml | - | - |
generateLombokConfig | Generates lombok.config | - | - |
generateHelmChart | Generates Helm chart | - | - |
processHelmChart | Processes Helm chart | - | - |
compile | Compiles main classes | classes | - |
verify | Runs all checks and tests | check | - |
kradleDump | Dumps kradle diagnostic information | - | - |
Features
kradle
groups its functionality into features. They must be enabled explicitly. For example to get support for
benchmarks:
kradle {
jvm {
benchmark.enable()
}
}
You can use one of the following statements, whatever fits your taste.
benchmark.enable()
benchmark(true)
benchmark()
If the feature has options, enable
takes a configuration code block as argument.
kradle {
jvm {
benchmark.enable {
jmh {
version("1.35")
}
}
}
}
This configures and enables the feature. You can omit enable
and use benchmark { … }
.
To configure the feature without enabling it, use configureOnly
.
It is also possible to disable features. This can be useful if you are using presets and want to get rid of inherited features.
benchmark.disable()
benchmark(false)
Options shown in this section of the documentation represent the defaults.
If the name of the option starts with use
, it adds dependencies to your project (e.g. useKotest
).
Features can have sub-features. For example, junitJupiter
is a sub-feature of test
.
kradle {
jvm {
test {
junitJupiter.enable {
version("5.9.1")
}
}
}
}
In contrast to normal features, some of them are enabled per default.
General
kradle {
general {
…
}
}
Groups general features.
Bootstrapping
kradle {
general {
bootstrap.enable()
}
}
Adds the task bootstrap
, which
- Initializes Git
- Adds Gradle wrapper
- Creates essentials directories and files
- Stages new files
Git integration
kradle {
general {
git.enable()
}
}
Adds the task generateGitignore
, which generates .gitignore with sane defaults.
gitCommit
, gitBranch
and gitBranchPrefix
are added to the project properties.
The gitBranchPrefix
is the branch name up to the first occurrence of /
, -
or _
.
Build profiles
kradle {
general {
buildProfiles.enable()
}
}
Adds profile
to the project properties.
Options
kradle {
general {
buildProfiles {
active("default")
}
}
}
active
: Sets the active build profile
Example
If you want to pass the profile via command line argument (./gradlew -Pprofile=<PROFILE>
), you can use following
snippet:
kradle {
general {
buildProfiles {
active(project.properties["profile"].toString())
}
}
}
Project properties
kradle {
general {
projectProperties.enable()
}
}
Looks for a file called project.properties in the project directory. If found, the entries are added to the project properties.
If build profiles are enabled, the entries of project-<PROFILE>.properties are also added. They have precedence over project.properties.
Build properties
kradle {
general {
buildProperties.enable()
}
}
Adds the task generateBuildProperties
, which generates a file build.properties containing the project name, group,
version and the build timestamp.
If build profiles are enabled, the active profile is added.
If Git integration is enabled, the Git commit id is added.
The task is executed after processResources
.
project.name=…
project.group=…
project.version=…
build.profile=…
build.timestamp=…
git.commit-id=…
Custom scripts
kradle {
general {
scripts.enable()
}
}
Creates new script tasks which execute a chain of shell commands.
Options
kradle {
general {
scripts {
"<NAME>" {
description("…")
dependsOn("…")
prompt(key = "…", text = "…", default = "…")
commands("…")
}
}
}
}
<NAME>
: Name of the created taskdescription
: Description of the created taskdependsOn
: List of task dependenciesprompt
: Asks for user input. The entered values can be accessed with$#{inputs.<KEY>}
. Can be called zero, once or multiple timescommands
: Commands to execute. If any fails, the execution is stopped and the build fails
Example
kradle {
general {
scripts {
"release" {
description("Create release branch and tag")
prompt(key = "version", text = "Version?", default = project.version.toString())
commands(
"git checkout -b release/$#{inputs.version}",
"git tag v$#{inputs.version}"
)
}
}
}
}
Adds the task release
which can be called like any other task: ./gradlew release
.
Helm charts
kradle {
general {
helm.enable()
}
}
Adds the task generateHelmChart
, which generates a basic Helm chart in src/main/helm.
Adds the task processHelmChart
, which copies src/main/helm to build/helm and expands all property references in Chart.yaml and values.yaml.
Adds following script tasks:
helmInstall
: Installs the chart build/helmhelmUpgrade
: Upgrades the releasehelmUninstall
: Uninstalls the release
Options
kradle {
general {
helm {
releaseName(project.name)
// valuesFile("…")
}
}
}
releaseName
: Release namevaluesFile
: Use values file withhelmInstall
andhelmUpgrade
(relative to project directory)
JVM features
kradle {
jvm {
…
}
}
Groups JVM related features.
Options
kradle {
jvm {
targetJvm("17")
}
}
targetJvm
: Sets target release ("--release"
)
Kotlin development
kradle {
jvm {
kotlin.enable()
}
}
Adds Kotlin Standard Library, Kotlin reflection library, and kotlin.test library dependencies.
Enables Opt-ins.
JSR-305 nullability mismatches are reported as error ("-Xjsr305=strict"
).
Plugins used: kotlinx.serialization Plugin, All-open Compiler Plugin, Java Plugin, detekt Plugin, ktlint Plugin
Sub-features
-
detekt static code analysis
- Enabled per default
- Requires feature code analysis
- Adds the tasks
generateDetektConfig
, which generates a configuration file with sane defaults
-
- Enabled per default
- Requires feature linting
- Uses all standard and experimental rules per default
Options
kradle {
jvm {
kotlin {
// useCoroutines("1.6.4")
lint {
ktlint.enable {
version("0.43.2")
rules {
// disable("…")
}
}
}
codeAnalysis {
detekt.enable {
version("1.21.0")
configFile("detekt-config.yml")
}
}
test {
// useKotest("5.2.3")
// useMockk("1.13.2")
}
}
}
}
useCoroutines
: Adds Kotlin coroutines dependencytest.useKoTest
: Adds kotest test dependencies (only if test improvements are enabled)test.useMockk
: Adds mockk test dependency (only if test improvements are enabled)ktlint.version
: ktlint version usedktlint.rules.disable
: Disables ktlint rule. Can be called multiple timesdetekt.version
: detekt version useddetekt.configFile
: detekt configuration file used (relative to project directory)
Java development
kradle {
jvm {
java.enable()
}
}
Plugins used: Java Plugin, PMD Plugin, SpotBugs Plugin, Checkstyle Plugin
Sub-features
- PMD
- Enabled per default
- Active rule sets:
errorprone
,multithreading
,performance
andsecurity
- SpotBugs
- Requires feature code analysis
- Enabled per default
- checkstyle
- Enabled per default
- Requires feature linting
- Looks for the configuration file checkstyle.xml in the project directory. If not found,
kradle
generates one
Options
kradle {
jvm {
java {
previewFeatures(false)
// withLombok("1.18.24")
lint {
checkstyle.enable {
version("10.3.4")
configFile("checkstyle.xml")
}
}
codeAnalysis {
pmd.enable {
version("6.50.0")
ruleSets {
bestPractices(false)
codeStyle(false)
design(false)
documentation(false)
errorProne(true)
multithreading(true)
performance(true)
security(true)
}
}
spotBugs.enable {
version("4.7.2")
// useFbContrib(7.4.7)
// useFindSecBugs(1.12.0)
}
}
}
}
}
previewFeatures
: Enables preview featureswithLombok
: Enables Project Lombok. Adds the taskgenerateLombokConfig
, which generates lombok.config with sane defaultscheckstyle.version
: checkstyle version usedcheckstyle.configFile
: checkstyle configuration file used (relative to project directory)pmd.version
: PMD version usedpmd.ruleSets.*
: Enables/disables PMD rule setsspotBugs.version
: SpotBugs version usedspotBugs.useFbContrib
: Enables fb-contrib pluginspotBugs.useFbContrib
: Enables Find Security Bugs plugin
Application development
kradle {
jvm {
application.enable()
}
}
Conflicts with library development.
If build profiles are enabled, the environment variable KRADLE_PROFILE
is set when
using run
.
Plugins used: Application Plugin
Options
kradle {
jvm {
application {
// mainClass("…")
}
}
}
mainClass
: Sets the main class (required)
Library development
kradle {
jvm {
library.enable()
}
}
Adds the task install
, which installs the library to your local Maven repository.
Conflicts with application development.
Plugins used: Java Library Plugin , Maven Publish Plugin
Dependency management
kradle {
jvm {
dependencies.enable()
}
}
Adds the task showDependencyUpdates
, which shows all available dependency updates. It only considers stable versions;
no alpha, beta, release candidate or milestone builds.
Plugins used: Gradle Versions Plugin
Options
kradle {
jvm {
dependencies {
useCaffeine("3.1.1")
useGuava("31.1-jre")
useLog4j("2.19.0")
}
}
}
useCaffeine
: Adds Caffeine caching libraryuseGuava
: Adds Guava Google core librariesuseLog4j
: Adds log4j logging
Vulnerability scans
kradle {
jvm {
vulnerabilityScan.enable()
}
}
Adds the task analyzeDependencies
, which scans all dependencies on the runtime and compile classpath for
vulnerabilities.
Plugins used: OWASP Dependency Check Plugin
Linting
kradle {
jvm {
lint.enable()
}
}
Adds the task lint
, which runs enabled linters.
lint
is executed when running check
.
Options
kradle {
jvm {
lint {
ignoreFailures(false)
}
}
}
ignoreFailures
: Build is successful, even if there are linting errors
Code analysis
kradle {
jvm {
codeAnalysis.enable()
}
}
Adds the task analyzeCode
, which runs enabled code analysis tools:
analyzeCode
is executed when running check
.
Options
kradle {
jvm {
codeAnalysis {
ignoreFailures(false)
}
}
}
ignoreFailures
: Build is successful, even if there are code analysis errors
Development mode
kradle {
jvm {
developmentMode.enable()
}
}
Adds the task dev
, which watches the directories src/main/kotlin, src/main/java and src/main/resource. If
changes are detected, the
application is stopped. Should be used with continuous build flag -t
to archive automatic rebuilds and restarts.
When launching the application with dev
, the environment variable KRADLE_DEV_MODE=true
is set.
If build profiles are enabled, the environment variable KRADLE_PROFILE
is set.
To speed up application start, the JVM flag -XX:TieredStopAtLevel=1
is used.
Requires application development.
Test improvements
kradle {
jvm {
test.enable()
}
}
Test file names can end with Test
, Tests
, Spec
or IT
.
When running tests, the environment variables KRADLE_PROJECT_DIR
and KRADLE_PROJECT_ROOT_DIR
are set.
If build profiles are enabled, the environment variable KRADLE_PROFILE
is set.
Adds the task runTests
, which runs all tests (unit, integration, functional, custom).
Plugins used: Java Plugin , Gradle Test Logger Plugin
Sub-features
- JUnit Jupiter testing framework
- Enabled per default
Options
kradle {
jvm {
test {
junitJupiter.enable {
version("5.9.1")
}
prettyPrint(false)
showStandardStreams(false)
withIntegrationTests(false)
withFunctionalTests(false)
/*
withCustomTests("<NAME>")
useArchUnit("1.0.0")
useTestcontainers("1.17.5")
*/
}
}
}
junitJupiter.version
: JUnit Jupiter version usedprettyPrint
: Prettifies test output with Gradle Test Logger PluginshowStandardStreams
: Shows stdout and stderr in test outputwithIntegrationTests
: Adds taskintegrationTest
, which runs tests under src/integrationTest. The task is executed when runningcheck
withFunctionalTests
: Adds taskfunctionalTest
, which runs tests under src/functionalTest. The task is executed when runningcheck
withCustomTests
: Adds task<NAME>Test
, which runs tests under src/<NAME>. The task is executed when runningcheck
. Can be called multiple timesuseArchUnit
: Adds ArchUnit test dependenciesuseTestcontainers
: Adds Testcontainers test dependencies
Code coverage
kradle {
jvm {
codeCoverage.enable()
}
}
Adds the task analyzeTestCoverage
, which runs enabled test coverage tools.
analyzeTestCoverage
is executed when running check
.
Plugins used: Kover, JaCocCo Plugin
Sub-features
- Kover
- Enabled per default
- Generates HTML report in build/reports/kover/
- JaCocCo
- Generates HTML report in build/reports/jacoco/
Options
kradle {
jvm {
codeCoverage {
kover {
excludes("…")
}
/*
jacoco.enable {
version("0.8.8")
excludes("…")
}
*/
}
}
}
kover.excludes
: List of test tasks to exclude from analysisjacoco.version
: JaCoCo version usedjacoco.excludes
: List of test tasks to exclude from analysis
Benchmarks
kradle {
jvm {
benchmark.enable()
}
}
Adds the task runBenchmarks
, which runs JMH benchmarks found under src/benchmark/kotlin.
Plugins used: kotlinx.benchmark Plugin , All-open Compiler Plugin
Options
kradle {
jvm {
benchmark {
jmh {
version("1.35")
}
}
}
}
jmh.version
: JMH version used
Packaging
kradle {
jvm {
packaging.enable()
}
}
Adds the task uberJar
, which creates an Uber-Jar. This is a JAR containing all dependencies.
Adds the task package
, which is an alias for jar
.
Adds Main-Class
the manifest, so the JAR is runnable (application only).
Plugins used: Gradle Shadow Plugin
Options
kradle {
jvm {
packaging {
uberJar {
minimize(false)
}
}
}
}
minimize
: Minimizes Uber-Jar, only required classes are added
Docker
kradle {
jvm {
docker.enable()
}
}
Adds the task buildImage
, which creates a Docker image using Jib.
Adds the task pushImage
, which pushes the container image to remote registry.
Adds the project property imageName
.
Files in src/main/extra/ will be copied to the image directory /app/extra/.
Plugins used: Jib Plugin
Requires application development.
Options
kradle {
jvm {
docker {
baseImage("bellsoft/liberica-openjdk-alpine:17")
imageName(project.name)
allowInsecureRegistries(false)
// ports(…)
// jvmOptions("…")
// arguments("…")
// withJvmKill(1.16.0")
withStartupScript(false)
}
}
}
baseImage
: Base image usedimageName
: Name of the created image (without tag)allowInsecureRegistries
: Allows use of insecure registriesports
: List of exposed portsjvmOptions
: Options passed to the JVMarguments
: Arguments passed to the applicationwithJvmKill
: Adds jvmkill to the image, which terminates the JVM if it is unable to allocate memorywithStartupScript
: Uses a script as entrypoint for the container. Either you provide your own script at src/main/extra/app.sh orkradle
will create one
Documentation
kradle {
jvm {
documentation.enable()
}
}
Adds the task generateDocumentation
, which uses Dokka to generate a HTML
documentation based on KDoc comments. The documentation can be found under build/docs.
Package and module documentation can be placed in package.md or module.md in the project or any source directory.
Plugins used: Dokka Plugin
Frameworks
kradle {
jvm {
frameworks {
…
}
}
}
Configures miscellaneous frameworks.
The integration of frameworks is very basic. For more control and features consider using their official Gradle plugins.
Spring Boot
kradle {
jvm {
frameworks {
springBoot.enable()
}
}
}
Adds Spring Boot and Spring Boot Test dependencies.
If build profiles are enabled, the environment variable SPRING_PROFILE_ACTIVE
is set when
using run
and dev
.
Options
kradle {
jvm {
frameworks {
springBoot {
version("2.7.4")
// withDevTools("2.7.4")
// useWeb("2.7.4")
// useWebFlux("2.7.4")
// useActuator("2.7.4")
}
}
}
}
version
: Spring Boot version usedwithDevTools
: Uses SpringBoot DevTools when runningdev
useWeb
: Adds Spring Web dependenciesuseWebFlux
: Adds Spring WebFlux dependenciesuseActuator
: Adds Spring Boot Actuator
Presets
Presets preconfigure kradle
for specific use cases. The options set by the preset can be overridden.
Example:
kradle {
kotlinJvmLibrary {
jvm {
buildProperties.disable()
}
}
}
Kotlin/JVM application
kradle {
kotlinJvmApplication {
jvm {
application {
mainClass("…")
}
}
}
}
Same as:
kradle {
general {
bootstrap.enable()
git.enable()
projectProperties.enable()
buildProperties.enable()
}
jvm {
kotlin {
useCoroutines()
lint {
ktlint {
rules {
disable("no-wildcard-imports")
}
}
}
test {
useKotest()
useMockk()
}
}
application {
mainClass("…")
}
dependencies.enable()
vulnerabilityScan.enable()
lint.enable()
codeAnalysis.enable()
developmentMode.enable()
test {
prettyPrint(true)
withIntegrationTests()
withFunctionalTests()
}
codeCoverage.enable()
benchmark.enable()
packaging.enable()
docker {
withJvmKill()
}
documentation.enable()
}
}
Kotlin/JVM library
kradle {
kotlinJvmLibrary.activate()
}
Same as:
kradle {
general {
bootstrap.enable()
git.enable()
projectProperties.enable()
buildProperties.enable()
}
jvm {
kotlin {
useCoroutines()
lint {
ktlint {
rules {
disable("no-wildcard-imports")
}
}
}
test {
useKotest()
useMockk()
}
}
library.enable()
dependencies.enable()
vulnerabilityScan.enable()
lint.enable()
codeAnalysis.enable()
test {
prettyPrint(true)
withIntegrationTests()
withFunctionalTests()
}
codeCoverage.enable()
benchmark.enable()
packaging.enable()
documentation.enable()
}
}
Java application
kradle {
javaApplication {
jvm {
application {
mainClass("…")
}
}
}
}
Same as:
kradle {
general {
bootstrap.enable()
git.enable()
projectProperties.enable()
buildProperties.enable()
}
jvm {
java {
withLombok()
codeAnalysis {
spotBugs {
useFbContrib()
useFindSecBugs()
}
}
}
application {
mainClass("…")
}
dependencies.enable()
vulnerabilityScan.enable()
lint.enable()
codeAnalysis.enable()
developmentMode.enable()
test {
prettyPrint(true)
withIntegrationTests()
withFunctionalTests()
}
codeCoverage.enable()
benchmark.enable()
packaging.enable()
docker {
withJvmKill()
}
documentation.enable()
}
}
Java library
kradle {
javaLibrary.activate()
}
Same as:
kradle {
general {
bootstrap.enable()
git.enable()
projectProperties.enable()
buildProperties.enable()
}
jvm {
java {
withLombok()
codeAnalysis {
spotBugs {
useFbContrib()
useFindSecBugs()
}
}
}
library.enable()
dependencies.enable()
vulnerabilityScan.enable()
lint.enable()
codeAnalysis.enable()
test {
prettyPrint(true)
withIntegrationTests()
withFunctionalTests()
}
codeCoverage.enable()
benchmark.enable()
packaging.enable()
documentation.enable()
}
}
Configuration DSL reference
This example shows all features enabled with their default configuration.
kradle {
general {
bootstrap.enable()
git.enable()
buildProfiles {
active("default")
}
projectProperties.enable()
buildProperties.enable()
scripts {
/*
"<NAME>" {
description("…")
dependsOn("…")
prompt(key = "…", text = "…", default = "…")
commands("…")
}
*/
}
helm {
releaseName(project.name)
// valuesFile("…")
}
}
jvm {
targetJvm("17")
kotlin {
// useCoroutines("1.6.4")
lint {
ktlint.enable {
version("0.43.2")
rules {
// disable("…")
}
}
}
codeAnalysis {
detekt.enable {
version("1.21.0")
configFile("detekt-config.yml")
}
}
test {
// useKotest("5.2.3")
// useMockk("1.13.2")
}
}
java {
previewFeatures(false)
// withLombok("1.18.24")
lint {
checkstyle.enable {
version("10.3.4")
configFile("checkstyle.xml")
}
}
codeAnalysis {
pmd.enable {
version("6.50.0")
ruleSets {
bestPractices(false)
codeStyle(false)
design(false)
documentation(false)
errorProne(true)
multithreading(true)
performance(true)
security(true)
}
}
spotBugs.enable {
version("4.7.2")
useFbContrib("7.4.7")
useFindSecBugs("1.12.0")
}
}
}
application {
// mainClass("…")
}
library.enable() // Conflicts with application
dependencies {
// useCaffeine("3.1.1")
// useGuava("31.1-jre")
// useLog4j("2.19.0")
}
vulnerabilityScan.enable()
lint {
ignoreFailures(false)
}
codeAnalysis {
ignoreFailures(false)
}
developmentMode.enable()
test {
junitJupiter.enable {
version("5.9.1")
}
prettyPrint(false)
showStandardStreams(false)
withIntegrationTests(false)
withFunctionalTests(false)
// withCustomTests("…")
// useArchUnit("1.0.0")
// useTestcontainers("1.17.5")
}
codeCoverage {
kover.enable {
// excludes("…")
}
jacoco.configureOnly {
version("0.8.8")
// excludes("…")
}
}
benchmark {
jmh {
version("1.35")
}
}
packaging {
uberJar {
minimize(false)
}
}
docker {
baseImage("bellsoft/liberica-openjdk-alpine:17")
imageName(project.name)
allowInsecureRegistries(false)
// jvmOptions("…")
// arguments("…")
// ports(…)
// withJvmKill("1.16.0")
withStartupScript(false)
}
documentation.enable()
frameworks {
springBoot {
version("2.7.4")
// withDevTools("2.7.4")
// useWeb("2.7.4")
// useWebFlux("2.7.4")
// useActuator("2.7.4")
}
}
}
}
How to report bugs
Please open a new issue and if possible provide the output
of gradle kradleDump
.
Versioning (since 2.0.0)
kradle
uses a MAJOR.MINOR.PATCH
pattern.
Patch release
- Contains only bug fixes.
- Configuration DSL will not change.
- Dependencies are only updated when required to fix an issue.
Minor release
- Introduces new features.
- Contains dependency upgrades.
- Configuration DSL can change, but in a backwards compatible manner.
- Default values for options can change.
- Functionality can change.
- Make sure to check the CHANGELOG before updating.
Major release
- Configuration DSL can change and violate backwards compatibility (Breaking change in the CHANGELOG).
- Otherwise same as minor release.
License
This project is licensed under the terms of the MIT license.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK