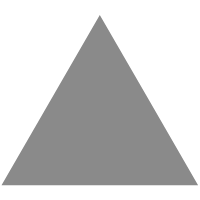
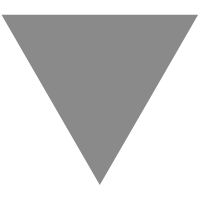
Watch user location using Geolocation API
source link: https://www.laravelcode.com/post/watch-user-location-using-geolocation-api
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Watch user location using Geolocation API
If you are developing real time user location application, then you may want to use The Geolocation API instead of third party services. The Geolocation API supports all modern web browsers. You don't need to write lengthy code.
The Geolocation API in Javscript is used to get user location. For privacy reason, user is asked permission to share user's location. If the user grant permission, you will get user location details object.
In this article, we will share how you can watch for user location.
navigator.geolocation.watchPosition() method
The navigator.geolocation.watchPosition() method registers a handler function that will be called automatically each time when user device location changes, and it will returns the updated location.
Syntax
navigator.geolocation.watchPosition(successFunction, errorFunction, options);
Parameters:
successFunction
when user grants permission
errorFunction Optional which calls if error occurs
options Optional configuration options for getting location
The method accepts three parameters,
// success function
function success (position) {
console.log(position);
}
// error function
function error (error) {
console.warn(error);
}
var options = {
enableHighAccuracy: true
};
const watchId = navigator.geolocation.watchPosition(success, error, options);
navigator.geolocation.clearWatch() method
navigator.geolocation.clearWatch() method is used to stop watching user location handling started in navigator.geolocation.watchPosition() method.
Syntax
navigator.geolocation.clearWatch(watchId);
Parameters:
watchId
Id of the geolocation.watchPosition() method.
Here is the full example rendering user location in Google Maps:
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>Google Maps</title>
<style>
#map {
height: 400px;
width: 600px;
}
</style>
</head>
<body>
<div id="map"></div>
<button onclick="stopWatch()">Stop</button>
<script async defer src="https://maps.googleapis.com/maps/api/js?key=GOOGLE_API_KEY"></script>
<script type="text/javascript">
const watchId = navigator.geolocation.watchPosition(
position => initMap(position)
);
function stopWatch() {
navigator.geolocation.clearWatch(watchId);
}
function initMap(position) {
const { latitude, longitude } = position.coords;
const center = {lat: latitude, lng: longitude};
const options = {zoom: 15, scaleControl: true, center: center};
map = new google.maps.Map(document.getElementById('map'), options);
const point = {lat: latitude, lng: longitude};
// create marker
var marker = new google.maps.Marker({position: point, map: map});
}
</script>
</body>
</html>
This will keep eye on user location. When user location updates, the watchPosition will send new location to Google Maps and Google Maps will update location in map.
Author : Harsukh Makwana

Hi, My name is Harsukh Makwana. i have been work with many programming language like php, python, javascript, node, react, anguler, etc.. since last 5 year. if you have any issue or want me hire then contact me on [email protected]
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK