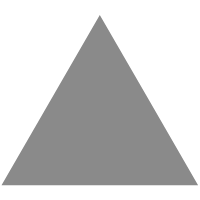
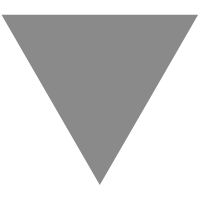
How to Generate QR Code in Laravel 8
source link: https://www.laravelcode.com/post/how-to-generate-qr-code-in-laravel-8
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
How to Generate QR Code in Laravel 8
QR(Quick Response) code is a type of barcode used to store data. A QR scanner reads the barcode and convert it to original form of data. This makes it simple to transfer data using QR code.
In Laravel application, it is simple to make QR code. In this article, we will use simplesoftwareio/simple-qrcode package for Laravel. It also provides variety of customization options. We will go through tutorial step by step from the scratch.
- Step 1: Create Laravel application
- Step 2: Install and configure package
- Step 3: Create controller and routes
- Step 4: Create routes
- Step 5: Create view file
So let's start from new Laravel application.
Step 1: Create Laravel application
The first step we do is to create fresh Laravel application using following command. So start Terminal and run the command where you want to create application.
composer create-project laravel/laravel qrcode
Once application is created, go to project directory in Terminal.
cd qrcode
Step 2: Install and configure package
In the second step, we will install package. Paste below command in Terminal and hit enter.
composer require simplesoftwareio/simple-qrcode
Once the package is installed, register the package classes to config/app.php file. Add below lined in providers and aliases array.
'providers' => [
SimpleSoftwareIO\QrCode\QrCodeServiceProvider::class,
],
'aliases' => [
'QrCode' => SimpleSoftwareIO\QrCode\Facades\QrCode::class,
],
Step 3: Create controller and routes
In the third step, we will create controller class using artisan command.
php artisan make:controller QRCodeController
This will make controller class app/Http/Controllers/QRCodeController.php
. Open the file and add the below two methods.
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
use SimpleSoftwareIO\QrCode\Facades\QrCode;
class QRCodeController extends Controller
{
/**
* QR code view.
*
* @return void
*/
public function index()
{
return view('qrCode');
}
/**
* Generate Qr code.
*
* @return void
*/
public function generate(Request $request)
{
$time = time();
// create a folder
if(!\File::exists(public_path('images'))) {
\File::makeDirectory(public_path('images'), $mode = 0777, true, true);
}
QrCode::generate($request->qr_message, 'images/'.$time.'.svg');
$img_url = 'images/'.$time.'.svg';
\Session::put('qrImage', $img_url);
return redirect()->route('QRCode.index');
}
}
Step 4: Create routes
Now create the routes in routes/web.php
file to handle controller methods.
<?php
use Illuminate\Support\Facades\Route;
use App\Http\Controllers\QRCodeController;
Route::get('qr-code', [QRCodeController::class, 'index'])->name('QRCode.index');
Route::post('generate', [QRCodeController::class, 'generate'])->name('QRCode.generate');
Step 5: Create view file
We need to create blade file to view QR code. Create qrCode.blade.php
file and put the following HTML code.
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>QR code generator</title>
<link href="https://cdn.jsdelivr.net/npm/[email protected]/dist/css/bootstrap.min.css" rel="stylesheet">
</head>
<body>
<div class="container mt-5">
<div class="row col-md-6">
<h1>QR Code generate</h1>
<form method="post" action="{{ route('QRCode.generate') }}">
@csrf
<div class="mb-3">
<label for="qr-message" class="form-label">Text</label>
<input type="text" class="form-control" id="qr-message" name="qr_message" aria-describedby="message-help">
<div id="message-help" class="form-text">The message that you want to save in code.</div>
</div>
<input type="submit" class="btn btn-success">
</form>
<div class="text-center">
@if(\Session::has('qrImage'))
<div class="mb-3">
<img src="{{ asset(\Session::get('qrImage')) }}" class="img img-responsive">
</div>
<a href="{{ asset(\Session::get('qrImage')) }}" class="btn btn-primary" download>Download</a>
{{ \Session::forget('qrImage') }}
@endif
</div>
</div>
</div>
</body>
</html>
That's it. Now run the Laravel server with command:
php artisan serve
In your browser go to http://localhost:8000/qr-code
and submit the form. Now scan the QR code with any mobile application and it will return the text that you have submited in textbox.
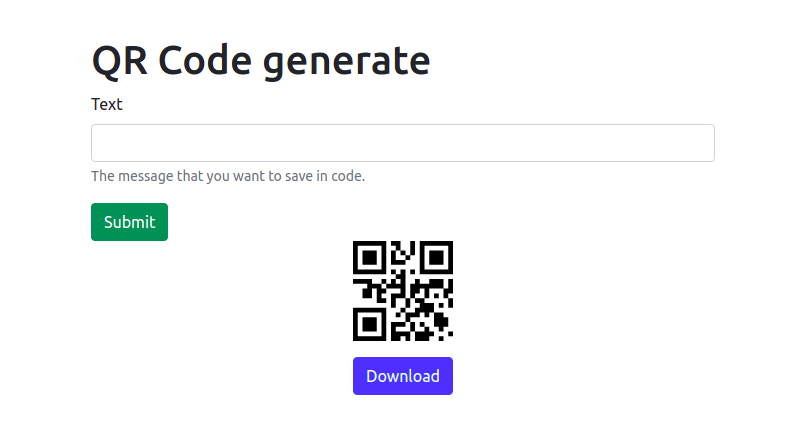
To customize QR code , visit official website.
Author : Harsukh Makwana

Hi, My name is Harsukh Makwana. i have been work with many programming language like php, python, javascript, node, react, anguler, etc.. since last 5 year. if you have any issue or want me hire then contact me on [email protected]
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK