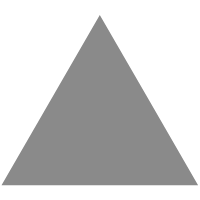
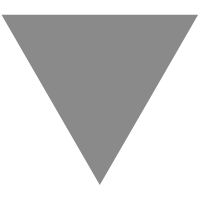
Customizable Checkbox and Radio Buttons using iCheck jQuery plugin
source link: https://www.laravelcode.com/post/customizable-checkbox-and-radio-buttons-using-icheck-jquery-plugin
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Customizable Checkbox and Radio Buttons using iCheck jQuery plugin
While creating simple html form, there are lots of plugins available by which you can easily and speedy design and create form for example, bootstrap, etc. One of the plugin is iCheck plugin.
iCheck is lightweight, simple jQuery(and Zepto) plugin which creates highly customizable checkboxes and radio buttons. iCheck creates identical inputs across different browsers and devices for desktop and mobile phones.
There are many key features of iCheck like:
Identical inputs across different browsers and devices — both desktop and mobile
Touch devices support — iOS, Android, BlackBerry, Windows Phone, Amazon Kindle
Keyboard accessible inputs — Tab, Spacebar, Arrow up/down and other shortcuts
Screenreader accessible inputs — ARIA attributes for VoiceOver and others
Customization freedom — use any HTML and CSS to style inputs (try 6 Retina-ready skins)
jQuery and Zepto JavaScript libraries support from single file
Lightweight size — 1 kb gzipped
32 options to customize checkboxes and radio buttons
11 callbacks to handle changes
9 methods to make changes programmatically
Saves changes to original inputs, works carefully with any selectors
How to use
First download iCheck plugin from GitHub and include icheck.js and theme css file in the <head> tag. Don't forget to include jQuery v1.7+ before icheck.js.
<head>
<link href="../skins/all.css?v=1.0.2" rel="stylesheet">
<script type="text/javascript" src="./js/jquery.js"></script>
<script type="text/javascript" src="../icheck.js?v=1.0.2"></script>
</head>
You can also use CDN files if you don't want to manually download and include.
<head>
<link href="https://cdnjs.cloudflare.com/ajax/libs/iCheck/1.0.2/skins/all.css" rel="stylesheet">
<script type="text/javascript" src="https://ajax.googleapis.com/ajax/libs/jquery/3.4.1/jquery.min.js"></script>
<script type="text/javascript" src="https://cdnjs.cloudflare.com/ajax/libs/iCheck/1.0.2/icheck.min.js"></script>
</head>
In the <form> element, add checkbox or radio button where you want to use it.
<input type="checkbox">
<input type="checkbox" checked>
<input type="radio" name="iCheck">
<input type="radio" name="iCheck" checked>
And lastly add javascript code before end of </body> tag.
<script type="text/javascript">
$(document).ready(function(){
$('input').iCheck({
checkboxClass: 'icheckbox_minimal',
radioClass: 'iradio_minimal',
increaseArea: '20%' // optional
});
});
</script>
iCheck has many schemes and colors. You can include specific theme css instead of all theme.
Minimal skin

Square skin

Flat skin

Line skin

Polaris skin

Futurico skin

Options
Bellow are default options, you can change these options.
{
// 'checkbox' or 'radio' to style only checkboxes or radio buttons, both by default
handle: '',
// base class added to customized checkboxes
checkboxClass: 'icheckbox',
// base class added to customized radio buttons
radioClass: 'iradio',
// class added on checked state (input.checked = true)
checkedClass: 'checked',
// if not empty, used instead of 'checkedClass' option (input type specific)
checkedCheckboxClass: '',
checkedRadioClass: '',
// if not empty, added as class name on unchecked state (input.checked = false)
uncheckedClass: '',
// if not empty, used instead of 'uncheckedClass' option (input type specific)
uncheckedCheckboxClass: '',
uncheckedRadioClass: '',
// class added on disabled state (input.disabled = true)
disabledClass: 'disabled',
// if not empty, used instead of 'disabledClass' option (input type specific)
disabledCheckboxClass: '',
disabledRadioClass: '',
// if not empty, added as class name on enabled state (input.disabled = false)
enabledClass: '',
// if not empty, used instead of 'enabledClass' option (input type specific)
enabledCheckboxClass: '',
enabledRadioClass: '',
// class added on indeterminate state (input.indeterminate = true)
indeterminateClass: 'indeterminate',
// if not empty, used instead of 'indeterminateClass' option (input type specific)
indeterminateCheckboxClass: '',
indeterminateRadioClass: '',
// if not empty, added as class name on determinate state (input.indeterminate = false)
determinateClass: '',
// if not empty, used instead of 'determinateClass' option (input type specific)
determinateCheckboxClass: '',
determinateRadioClass: '',
// class added on hover state (pointer is moved onto input)
hoverClass: 'hover',
// class added on focus state (input has gained focus)
focusClass: 'focus',
// class added on active state (mouse button is pressed on input)
activeClass: 'active',
// adds hoverClass to customized input on label hover and labelHoverClass to label on input hover
labelHover: true,
// class added to label if labelHover set to true
labelHoverClass: 'hover',
// increase clickable area by given % (negative number to decrease)
increaseArea: '',
// true to set 'pointer' CSS cursor over enabled inputs and 'default' over disabled
cursor: false,
// set true to inherit original input's class name
inheritClass: false,
// if set to true, input's id is prefixed with 'iCheck-' and attached
inheritID: false,
// set true to activate ARIA support
aria: false,
// add HTML code or text inside customized input
insert: ''
}
Callbacks
iCheck provides bellow callbacks, which can be used to handle on changes done.
Callback name | When used |
---|---|
ifClicked | user clicked on a customized input or an assigned label |
ifChanged | input's checked, disabled or indeterminate state is changed |
ifChecked | input's state is changed to checked |
ifUnchecked | checked state is removed |
ifToggled | input's checked state is changed |
ifDisabled | input's state is changed to disabled |
ifEnabled | disabled state is removed |
ifIndeterminate | input's state is changed to indeterminate |
ifDeterminate | indeterminate state is removed |
ifCreated | input is just customized |
ifDestroyed | customization is just removed |
Use on() method to bind them to inputs:
$('input').on('ifChecked', function(event) {
alert(event.type + ' callback');
});
iCheck supports bellow methods to make changes programmatically.
$('input').iCheck('uncheck'); — remove checked state
$('input').iCheck('toggle'); — toggle checked state
$('input').iCheck('disable'); — change input's state to disabled
$('input').iCheck('enable'); — remove disabled state
$('input').iCheck('indeterminate'); — change input's state to indeterminate
$('input').iCheck('determinate'); — remove indeterminate state
$('input').iCheck('update'); — apply input changes, which were done outside the plugin
$('input').iCheck('destroy'); — remove all traces of iCheck
You can also specify callback function, that will be executed on each method call:
$('input').iCheck('check', function() {
alert('Well done, Sir');
});
This way you can easily create beautiful checkboxes and radio buttons. I hope it will help you.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK