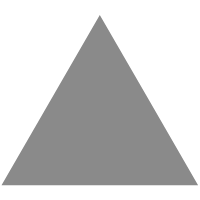
2
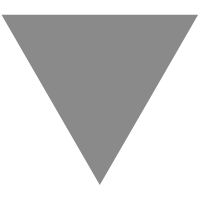
Easy inline web worker with Typescript
source link: https://gist.github.com/julianpoemp/1292445696eae2ea319d92ae15ecffa4
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
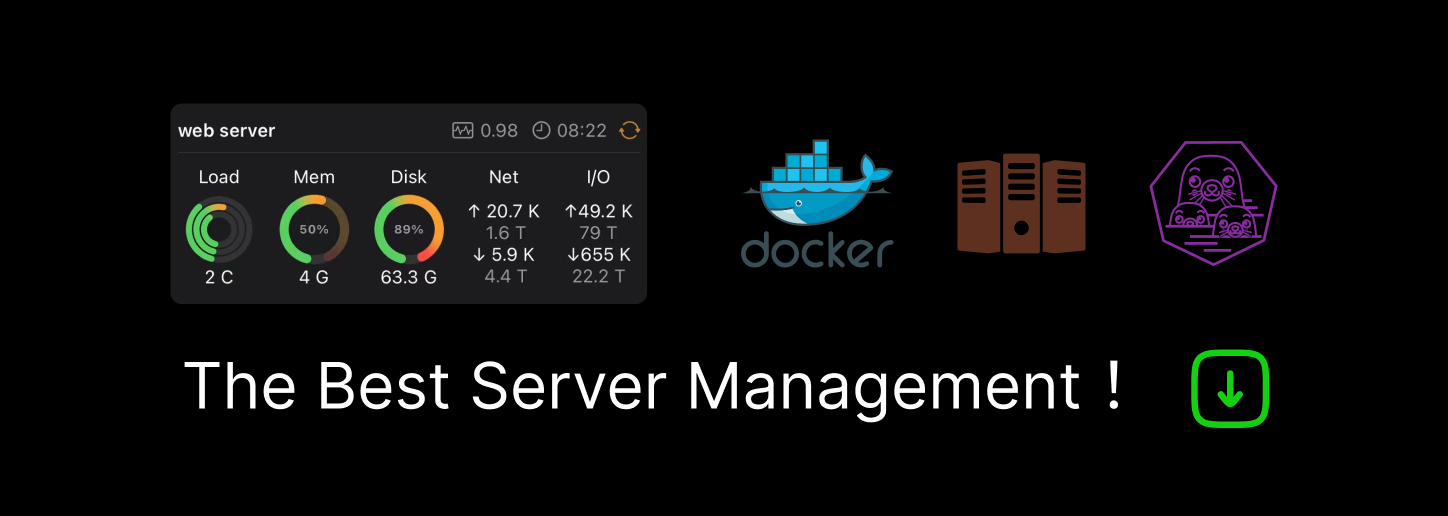
Easy web worker with Typescript
This code makes use of two classes: TsWorker and TsWorkerJob. TsWorker initializes an inline worker and runs one job. The TsWorker class needs to be initialized with a function and the function's arguments as an array.
Example:
const tsWorker = new TsWorker();
// create a new job. You can create a sub class of TsWorker so that you don't have to add the function any time.
const tsJob: TsWorkerJob = new TsWorkerJob((args: any[]) => {
return new Promise<any>((resolve, reject) => {
// because args is an array you need to use indexes.
const time = args[0];
console.log(`caculate anything...`);
setTimeout(() => {
resolve(42);
}, time);
});
}, [3000]);
// run the job asynchronous
tsWorker.run(tsJob).then((ev: TsWorkerEvent) => {
// finished
const secondsLeft = (ev.statistics.ended - ev.statistics.started) / 1000;
console.log(`the result is ${ev.result}`);
console.log(`time left for calculation: ${secondsLeft}`);
}).catch((error) => {
// error
console.log(`error`);
console.error(error);
});
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK