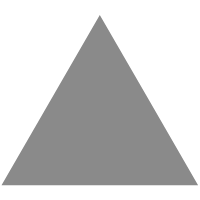
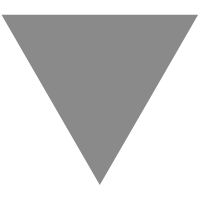
Delete an Element From an Array in PHP
source link: https://www.laravelcode.com/post/delete-an-element-from-an-array-in-php
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Delete an Element From an Array in PHP
While working with PHP array, you may want to remove few elements from an array. PHP has huge variety of array functions by which you can do this. There are different ways to delete an array element from an array in different situations, where some are more useful for some specific tasks than others.
Deleting a single array element
If you want to delete an element from array by key, unset()
is the best and simple way.
<?php
$arr = [0 => 'a', 1 => 'b', 2 => 'c'];
unset($arr[1]); // specify index of element you want to remove
print_r($arr); // Array ( [0] => a [2] => c )
Note that when you use unset()
method, array key remains same. If you also want to reindex the array after element remove, array_splice()
is the right option. The array_splice()
function removes selected elements from an array and reindex them.
<?php
$arr1 = [0 => 'a', 1 => 'b', 2 => 'c', 3 => 'd'];
array_splice($arr1, 1, 1);
print_r($arr1);
You can also insert new element in place of old element. array_splice()
takes forth argument as array for replacement.
// syntax
array_splice($array, offset, length, replacement)
Where $array
is selected array, offset
is start of the removed portion of array, length
is how many elements will be removed. replacement
array will be replaced with removed element.
<?php
$arr1 = [0 => 'a', 1 => 'b', 2 => 'c', 3 => 'd'];
$arr2 = [4 => 'e', 5 => 'f'];
array_splice($arr1, 1, 2, $arr2);
print_r($arr1);
If you simply want to remove last element of array, array_pop()
can be use for that. array_pop()
returns the value of the last element of array, shortening the array by one element.
<?php
$arr = [0 => 'a', 1 => 'b', 2 => 'c', 3 => 'd'];
$pop = array_pop($arr);
array_pop($arr);
print_r($arr); // Array ( [0] => a [1] => b )
print_r($pop); // d
If you don't know key of element you want to remove, then you can use array_search()
to get the key and remove it with unset if found.
<?php
$arr = [0 => 'a', 1 => 'b', 2 => 'c', 3 => 'd'];
if (($key = array_search('b', $arr)) !== false) {
unset($arr[$key]);
}
Delete multiple array elements
If you want to delete multiple elements from the array and you don't want to use unset()
or array_splice()
function multiple times, array_diff()
will help to remove multiple elements.
<?php
$arr = ['blue', 'green', 'yellow', 'red', 'orange', 'yellow'];
$new_arr = array_diff($arr, ['yellow', red]);
print_r($new_arr); // Array ( [0] => blue [1] => green [4] => orange )
I hope you will like this article.
Author : Harsukh Makwana

Hi, My name is Harsukh Makwana. i have been work with many programming language like php, python, javascript, node, react, anguler, etc.. since last 5 year. if you have any issue or want me hire then contact me on [email protected]
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK