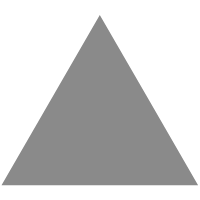
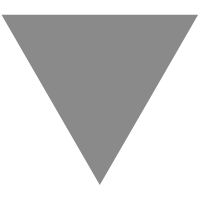
Laravel 8 Multiple Authentication Tutorial example
source link: https://www.laravelcode.com/post/laravel-8-multiple-authentication-tutorial-example
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Laravel 8 Multiple Authentication Tutorial example
If you are building an application which requires multiple level of access for multiple users, then you have to build multiple authentication system for multiple users. Laravel provides out of the box authentication for users table.
There are mainly two ways to create multiple authentication system for different useres. Either you use single table for all users on role-permission bases. This will make things complicated if you have many user type. The other option is to use different tables for different types of users.
In this article, I will share you how you can create multiple authentication system using users and admins table. We will go through step by step from fresh Laravel setup and Laravel Breeze. So let's start from creating new Admin model.
Step 1: Create Admin model
In your Terminal or CMD, go through your project's root directory and run the below command to create Admin model.
php artisan make:model Admin
Now open the Admin model at app/Models directory and change exends class to Illuminate\Foundation\Auth\User as Authenticatable instead of Model.
<?php
namespace App\Models;
use Illuminate\Database\Eloquent\Factories\HasFactory;
use Illuminate\Foundation\Auth\User as Authenticatable;
use Illuminate\Database\Eloquent\Model;
class Admin extends Authenticatable
{
use HasFactory;
}
Step 2: configure config/auth.php
Now we need to add new authentication providers in config/auth.php file. Open file and first add new admin guard in 'guards' array.
'guards' => [
'web' => [
'driver' => 'session',
'provider' => 'users',
],
'admin' => [
'driver' => 'session',
'provider' => 'admins',
],
],
In the same file, add new providers in providers array.
'providers' => [
'users' => [
'driver' => 'eloquent',
'model' => App\Models\User::class,
],
'admins' => [
'driver' => 'eloquent',
'model' => App\Models\Admin::class,
],
],
Step 3: Add migration for admins table
In this step, we will create new migration class for admins table. In the Terminal, run the following command to create new migration file.
php artisan make:migration create_admins_table
This will create new migration at database/migrations
directory. Open file and add new fields in up() method. We will create only login functionalities for admins table, If you want to add register and email verifucation functionalities, you need to add more fields in it.
<?php
use Illuminate\Database\Migrations\Migration;
use Illuminate\Database\Schema\Blueprint;
use Illuminate\Support\Facades\Schema;
class CreateAdminsTable extends Migration
{
/**
* Run the migrations.
*
* @return void
*/
public function up()
{
Schema::create('admins', function (Blueprint $table) {
$table->id();
$table->string('name');
$table->string('email')->unique();
$table->string('password');
$table->timestamps();
});
}
/**
* Reverse the migrations.
*
* @return void
*/
public function down()
{
Schema::dropIfExists('admins');
}
}
After you define fields in it, run the migrate command to generate admins table in database.
php artisan migrate
Step 4: Login routes
From the Laravel 8, login and registers routes for users are defined in routes/auth.php file. We will also add admins login routes in it. You are free to define them in routes/web.php also.
Add following routes in it.
<?php
use Illuminate\Support\Facades\Route;
use App\Http\Controllers\Auth\AdminAuthController;
Route::get('/admin/login', [AdminAuthController::class, 'showLoginForm'])->name('admin.login');
Route::post('/admin/login', [AdminAuthController::class, 'login'])->name('admin.login');
Route::get('/admin/logout', [AdminAuthController::class, 'logout'])->name('admin.logout');
Step 5: Create a controller class
We need to create an controller for login and logout feature. We will create Controller in the Auth directory, so create a controller by running below command in Terminal.
php artisan make:controller Auth/AdminAuthController
Open the class file and add the methods as defined in routes file.
<?php
namespace App\Http\Controllers\Auth;
use Auth;
use App\Http\Controllers\Controller;
use App\Http\Requests\Auth\LoginRequest;
use Illuminate\Http\Request;
class AdminAuthController extends Controller
{
/**
* Display admin login view
*
* @return \Illuminate\View\View
*/
public function showLoginForm()
{
if (Auth::guard('admin')->check()) {
return redirect()->route('admin.dashboard');
} else {
return view('auth.adminLogin');
}
}
/**
* Handle an incoming admin authentication request.
*
* @param \App\Http\Requests\Auth\LoginRequest $request
* @return \Illuminate\Http\RedirectResponse
*/
public function login(Request $request)
{
$this->validate($request, [
'email' => 'required|email',
'password' => 'required',
]);
if(auth()->guard('admin')->attempt([
'email' => $request->email,
'password' => $request->password,
])) {
$user = auth()->user();
return redirect()->intended(url('/admin/dashboard'));
} else {
return redirect()->back()->withError('Credentials doesn\'t match.');
}
}
/**
* Destroy an authenticated session.
*
* @param \Illuminate\Http\Request $request
* @return \Illuminate\Http\RedirectResponse
*/
public function logout(Request $request)
{
Auth::guard('admin')->logout();
$request->session()->invalidate();
$request->session()->regenerateToken();
return redirect('/');
}
}
Explanation:
You already knows which method does what. showLoginForm() method view the admin login form. login() method handle the authentication request and redirect to dashboard and logout() method logout admin from the current session.
Step 6: Create admin login blade.
In this step, we will create a view for admin login view. Create a blade view at resources/views/auth/adminLogin.blade.php and add the following login HTML code.
<!DOCTYPE html>
<html>
<head>
<title>Neumorphism/Soft UI</title>
<link href="https://cdn.jsdelivr.net/npm/[email protected]/dist/css/bootstrap.min.css" rel="stylesheet">
<style type="text/css">
.form-control,
.form-control:focus,
.btn {
border-radius: 50px;
background: #e0e0e0;
box-shadow: 20px 20px 60px #bebebe,
-20px -20px 60px #ffffff;
}
</style>
</head>
<body>
<div class="container">
<div class="row">
<div class="col-6 offset-3">
<h1 class="text-center m-5">Admin login</h1>
@if(\Session::has('error'))
<div class="alert alert-danger">{{ \Session::get('error') }}</div>
@endif
<form method="post" action="{{ route('admin.login') }}">
@csrf
<div class="row mt-3">
<div class="form-floating mb-3">
<input type="email" class="form-control" id="email" name="email" placeholder="Input email address">
<label for="email">Email address</label>
</div>
<div class="form-floating mb-3">
<input type="password" class="form-control" id="password" name="password" placeholder="Input password">
<label for="password">Password</label>
<button type="submit" class="btn">Submit</button>
</div>
</div>
</form>
</div>
</div>
</div>
</body>
</html>
Step 7: Create AdminController for admin dashboard
We also need to send admin user to somewhere after login success. We will create a dashboard view. First we need to create a controller. Run the following command into Terminal to create a controller.
php artisan make:controller AdminController
Now open controller and add dashboard() method in it.
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
class AdminController extends Controller
{
/**
* redirect admin after login
*
* @return \Illuminate\View\View
*/
public function dashboard()
{
return view('adminDashboard');
}
}
Step 8: Create a route for dashboard
We also need to create a protected route for admin dashboard. Open a routes/web.php and add new route.
<?php
use Illuminate\Support\Facades\Route;
use App\Http\Controllers\AdminController;
Route::group(['middleware' => ['auth:admin']], function () {
Route::get('admin/dashboard', [AdminController::class, 'dashboard'])->name('admin.dashboard');
});
Notice that we have wrapped admin dashboard route to auth:admin middleware. This prevents unauthenticated users to access the route. When you create a new guard in config/auth.php file, a new middleware is also generate with same name, auth prefixed. You can also define middleware in controller's __construct() method or chain middleware() method in route.
Step 9: Create a dashboard blade view
In this step, we need to create a view which will load when admin login. A logout route is also included in the view. Create resources/views/adminDashboard.blade.php file and add the following HTML code.
<!DOCTYPE html>
<html>
<head>
<title>Neumorphism/Soft UI</title>
<link href="https://cdn.jsdelivr.net/npm/[email protected]/dist/css/bootstrap.min.css" rel="stylesheet">
<style type="text/css">
.form-control,
.form-control:focus,
.btn {
border-radius: 50px;
background: #e0e0e0;
box-shadow: 20px 20px 60px #bebebe,
-20px -20px 60px #ffffff;
}
</style>
</head>
<body>
<div class="container">
<div class="row">
<div class="col-6 offset-3">
<h1 class="text-center m-5">Admin dashboard</h1>
@if(\Session::has('error'))
<div class="alert alert-danger">{{ \Session::get('error') }}</div>
@endif
<form method="get" action="{{ route('admin.logout') }}">
@csrf
<div class="row mt-3">
<button type="submit" class="btn">Logout</button>
</div>
</form>
</div>
</div>
</div>
</body>
</html>
That's it. Our all coding part is done. Now we will do test on admin and user login. Run a Laravel server using below command:
php artisan serve
In your browser, go to the url http://localhost:8000/login for user login or http://localhost:8000/admin/login for admin login. Confirm that after login to one guard, you can't access to other guard's route.
Note: To create a admin user, you can always create a normal user using register route at http://localhost:8000/register and create a new user. After creating the user, copy this row to admins table. This is only for testing. Don't use same email and password for admins and users table else someone with one guard may try and get access with other guard.
Conclusion
This way, you can create as many authentication users as you want. You don't need to manage roles and permission because all the users use different guard.
I hope you liked the tutorial and will help in your development.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK