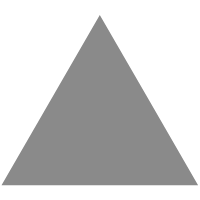
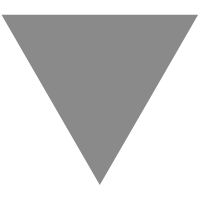
Stacks in Javascript
source link: https://medium.com/@dcortes.net/stacks-in-javascript-13bfdf65fa71
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Stacks in Javascript

Introduction article to the data structure. Stack concept and examples applied to the Javascript language.
Introduction
Data structures are a means of handling large amounts of data efficiently, Knowing its structure and composition gives us more effective tools to design our product in relation to certain problems. On this occasion we will perform a stacks introduction, reviewing its peculiarity and use.
Stack concept
Stacks are a dynamic data structure that allows elements to be stored and retrieved based on a structure LIFO (last in, first out). The given name comes from the analogy of a set of physical items stacked on top of each other, making it easy to grab an item from the top of the stack.

Stack implementation in Javascript
To implement a stack in Javascript we will rely on the repository https://github.com/trekhleb/javascript-algorithms that contains an excellent implementation, but we will simplify some methods and structures to be able to understand its operation. We will support everyone in object-oriented programming.
Stack class
class that represents a stack, this class will have the property items
that represents a set of elements to store (in this case, we will use an array of elements, but it can be any other). At startup, the items
property will have no elements.
class Stack {
constructor() {
this.items = [];
}
}
The next steps will be to add methods to the Stack
class that will help us perform the manipulation of elements in our stack.
Method to insert elements
To insert items into our stack, we’ll create the common method called a push. This method stacks elements.
push()
push(element) {
this.items.push(element);
}

Element removal method
To remove items from our stack, we will create the common method called pop. This method unstacks elements.
pop()
pop() {
return this.items.pop();
}

Element display method
Another of the most common operations of the stacks is the visualization of the element that is about to leave. That is the element that is on top. This method is called peek.
peek()
peek() {
return this.items[this.items.length - 1];
}
Full code
Ending
In this article, we take a simple look at stacks, however, these methods should be enough to cover basic use cases. Of course, there are many ways to extend and refine our example. I recommend to continue exploring implementations and adapt them to your need.
Thanks for coming this far, if you find this useful don’t forget to clap 👏 and share 😀. Subscribe to receive more content 🔔.
If you need additional help, please contact me 🤠.
Thank you very much for reading, I appreciate your time.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK