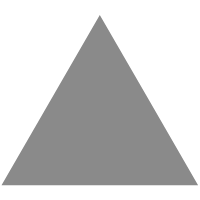
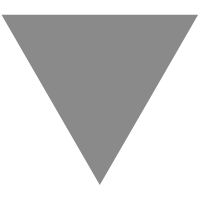
ASP.NET Core 使用 Web Socket
source link: https://blog.51cto.com/u_15706023/5635347
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
WebSocket:
WebSocket 是 HTML5 开始提供的一种在单个 TCP 连接上进行全双工通讯的协议。
WebSocket 使得客户端和服务器之间的数据交换变得更加简单,允许服务端主动向客户端推送数据。在 WebSocket API 中,浏览器和服务器只需要完成一次握手,两者之间就直接可以创建持久性的连接,并进行双向数据传输。在 WebSocket API 中,浏览器和服务器只需要做一个握手的动作,然后,浏览器和服务器之间就形成了一条快速通道。两者之间就直接可以数据互相传送。
基本使用:
在.NET 中,提供了websocket 的支持,在System.Net.WebSockets命名空间下,提供websocket支持。也有WebSocket4Net这样的进一步封装的nuget包。这里我们使用.net 默认提供的WebSockets命名空间。
新建ASP.NET Core web api项目,并添加如下代码。
startup代码
public void Configure(IApplicationBuilder app, IWebHostEnvironment env)
{
if (env.IsDevelopment())
{
app.UseDeveloperExceptionPage();
}
app.UseHttpsRedirection();
app.UseRouting();
app.UseAuthorization();
// 启用websocket中间件
app.UseWebSockets();
app.UseEndpoints(endpoints =>
{
endpoints.MapControllers();
});
}
服务器端controller代码
namespace WebSocketTest1.Controllers
{
[ApiController]
[Route("[controller]")]
public class WebSocketController : ControllerBase
{
[HttpGet("/ws")]
public async Task Get()
{
// 判断是否是 websocket 协议的请求
if (HttpContext.WebSockets.IsWebSocketRequest)
{
// 如果是 websocket 协议的请求,则获取到当前的 websocket 请求
using var websocket = await HttpContext.WebSockets.AcceptWebSocketAsync();
// websocket 建立完成,就执行定义的方法,该方法进行收发信息的逻辑代码
await Echo(websocket);
}
else
{
// 如果不是websocket协议的请求,返回400
HttpContext.Response.StatusCode = 400;
}
}
[HttpGet("/test")]
public IActionResult Test()
{
return Ok("test");
}
/// <summary>
/// 收发信息
/// </summary>
/// <param name="webSocket"></param>
/// <returns></returns>
private static async Task Echo(WebSocket webSocket)
{
// 接受信息的数组
var buffer = new byte[1024 * 4];
// 接受客户端消息
var result = await webSocket.ReceiveAsync(new ArraySegment<byte>(buffer), CancellationToken.None);
// 如果客户端没有关闭,一切正常没有异常,就保持和客户端的连接,正常收发
while (!result.CloseStatus.HasValue)
{
// 解析客户端发送的消息,并且定义返回到客户端的消息,生产byte数组
var serverMsg = Encoding.UTF8.GetBytes($"Server: Hello. You said: {Encoding.UTF8.GetString(buffer)}");
// 将消息发送到客户端
await webSocket.SendAsync(new ArraySegment<byte>(serverMsg, 0, serverMsg.Length), result.MessageType, result.EndOfMessage, CancellationToken.None);
// 再次接收客户端的消息
result = await webSocket.ReceiveAsync(new ArraySegment<byte>(buffer), CancellationToken.None);
}
// 客户端出现异常了,则服务器可以关闭连接了
await webSocket.CloseAsync(result.CloseStatus.Value, result.CloseStatusDescription, CancellationToken.None);
}
}
}
客户端可以直接在浏览器console控制台
let webSocket = newWebSocket(‘wss://localhost:端口号/ws’);
验证连接
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK