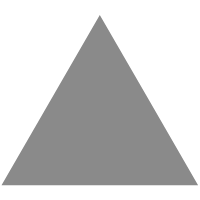
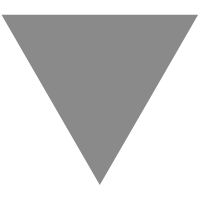
CoffeeScript: a TDD example
source link: https://dzone.com/articles/coffeescript-tdd-example
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
CoffeeScript: a TDD example
CoffeeScript is a language building an abstraction over JavaScript (as the similar name suggests.) It is an abstraction over the syntax of JavaScript, not over its concepts: the language is still based on functions as objects which may bind to other objects, and prototypical inheritance.
CoffeeScript favors the best practices of JavaScript by transforming abstractions you would have written anyway, or borrowed from a framework, into language concepts for maximum conciseness. It has a compilation step - as every language must compile to a lower-level one, like C or Java.
Since cowboy coding is not my preferred way to work, I prepared a Test-Driven Development example by using jsTestDriver. In this article, you get two things: a CoffeeScript introduction, and the tools for unit testing it (and consequently, how to TDD with CoffeeScript).
Building on Top of JavaScript
There is something to be said for those who go out of their way to try to improve the infrastructure of existing programs. Many have taken a stab at it by creating tools that are a compliment to JavaScript, and it appears to have worked quite well in this case. The reason? Because JavaScript is in need of some improvements.
One of the programs that people have come up with is CoffeeScript. It is literally a bit of computer code that overlays the existing JavaScript codes to make it read even more fluidly. The benefit that one gets from this is that they can start to use JavaScript in a more efficient way with fewer bumps in the road. As it stands right now, JavaScript has numerous challenging issues that we must recognize. However, that can all be amended by using JavaScript as it was intended and just working towards perfecting it.
CoffeeScript is certainly not the end all be all of the programs for improving JavaScript, but it is a great step in the right direction. People should at least try out the CoffeeScript code to see if it may be useful to them in terms of improving the quality of codes that they receive from JavaScript. If so, then it will have been worth the trouble.
The infrastructure
The basic structure consists of two folders: src/ and lib/; remember the compilation step. We'll put .coffee files into src/ and compile them to .js equivalents in a symmetrical tree in lib/.
We add also a jsTestDriver.conf file to tell the unit testing framework all the files to load, which are only the "binary" .js scripts:
server: http://localhost:4224 load: - lib/*.js
Compiling
This is the first version of the test I've managed to write, fizzbuzztest.coffee. It is a tautology that should always pass:
mytest = () -> assertEquals(1, 1) tests = { "test1is1": mytest } TestCase("tests for fizzbuzz kata", tests)
You see here that functions are still first-class objects, but only anonymous functions are supported. CoffeeScript is a Python/Ruby-like language without semicolons, and there are some affinities and common backgrounds with the latter language.
I still use the old syntax for calling functions for now, although parentheses can be omitted in many cases. CoffeeScript is conservative, and even accepts semicolons if you want to write them.
I compiled this script with coffee -o lib/ -c src/. fizzbuzztest.js is the result:
(function(){ var mytest, tests; mytest = function() { return assertEquals(1, 1); }; tests = { "test1is1": mytest }; TestCase("tests for fizzbuzz kata", tests); })();
The global namespace is not touched by default, and var keywords are automatically introduced to preserve it. When I later needed the global namespace, I wrote:
this.fizzbuzz = /* ... function definition ... */
This in this case is the window object or the other global object where you execute the code.
Running
To run the test, we must initialize the test driver (only once):
jsTestDriver java -jar JsTestDriver-1.3.2.jar --port 4224
jsTestDriver will now listen at localhost:4224. Load this URL in your browser and capture it by clicking on the link. Tests will be executed inside the browser when requested: for more details see the related article.
Every time you want to run the tests, execute them from the command line:
java -jar JsTestDriver-1.3.2.jar --tests all
This is the complete history of my kata. Here is the final version of the code (spoiler alert!), with support for addition of other factors than 3 or 5. The code is probably uglier than average, but it compiles:
tests = { "test ordinary numbers are unchanged": -> assertEquals(1, fizzbuzz(1)) assertEquals(2, fizzbuzz(2)) assertEquals(4, fizzbuzz(4)) assertEquals(142, fizzbuzz(142)) "test multiples of 3 become fizz": -> assertEquals("Fizz", fizzbuzz(3)) assertEquals("Fizz", fizzbuzz(6)) assertEquals("Fizz", fizzbuzz(9)) "test multiples of 5 become buzz": -> assertEquals("Buzz", fizzbuzz(5)) assertEquals("Buzz", fizzbuzz(10)) "test multiples of 3 and 5 become fizzbuzz": -> assertEquals("FizzBuzz", fizzbuzz(15)) assertEquals("FizzBuzz", fizzbuzz(45)) } TestCase("tests for fizzbuzz kata", tests)
newRule = (word, divisor) -> (number) -> return word if number % divisor == 0 "" newFizzBuzz = (rules) -> (number) -> result = "" concatenation = (rule) -> result = result + rule(number) concatenation rule for rule in rules return result if result number fizzRule = newRule("Fizz", 3) buzzRule = newRule("Buzz", 5) this.fizzbuzz = newFizzBuzz([fizzRule, buzzRule])
Comments On The Experience
CoffeeScript offers a shorter syntax, which presents a bit of a learning curve but not a steep one. I went through the whole example in 1 hour (I already knew how to use jsTestDriver, however.)
Syntax shapes how you write code by making some things easier: I found myself using higher-order functions which create other ones more often, since creating a function now is just a matter of putting -> before some lines of code.
Variable naming is also simpler as you just have to think of the name, not about var or polluting the scope. More time to dedicate to design, and less to language issues. Some one-liners like the instruction if the expression is handy but not essential, and are there due to Ruby's inspiration.
There's, even more, to discover in CoffeeScript, such as the options for the binding of functions which helps not to lose the reference to this. However, the question is if all this convenience has more value than the time spent to learn a new language and add infrastructure to make it work - the compiler, the build hooks, and the parallel tree to ignore in your version control system.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK