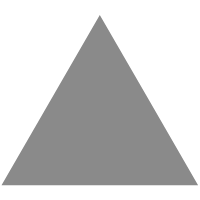
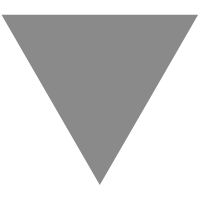
Vue Js File Download Example with Axios
source link: https://www.laravelcode.com/post/vue-js-file-download-example-with-axios
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Vue Js File Download Example with Axios
In this article, I will share with you how to download files in VueJs using Axios with a simple example. as you know files uploading and downloading this a common feature in any web application. so, here i will share file downloading in vuejs with a simple example. just follow the steps.
As we know Axios js is very popular for HTTP requests. you can fire get, post, put, etc request using Axios js in Vue js, node js, react js, etc. but if you need the same requirement to download file response from API and used to give download using Axios js then how you can do that? i will help you to do file downloading using Axios.
Axios HTTP code :
axios({
url: 'http://localhost:8000/api/get-file',
method: 'GET',
responseType: 'blob',
}).then((response) => {
var fileURL = window.URL.createObjectURL(new Blob([response.data]));
var fileLink = document.createElement('a');
fileLink.href = fileURL;
fileLink.setAttribute('download', 'file.pdf');
document.body.appendChild(fileLink);
fileLink.click();
});
HTML Code :
<!DOCTYPE html>
<html>
<head>
<title>Download File using Axios Vue JS? - HackTheStuff</title>
<script src="https://cdn.jsdelivr.net/npm/vue"></script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/axios/0.19.0/axios.min.js" integrity="sha256-S1J4GVHHDMiirir9qsXWc8ZWw74PHHafpsHp5PXtjTs=" crossorigin="anonymous"></script>
</head>
<body>
<div id="app">
<button @click="onClick()">DownLoad</button>
</div>
<script type="text/javascript">
var app = new Vue({
el: '#app',
methods: {
onClick() {
axios({
url: 'http://localhost:8000/my.pdf',
method: 'GET',
responseType: 'blob',
}).then((response) => {
var fileURL = window.URL.createObjectURL(new Blob([response.data]));
var fileLink = document.createElement('a');
fileLink.href = fileURL;
fileLink.setAttribute('download', 'file.pdf');
document.body.appendChild(fileLink);
fileLink.click();
});
}
}
})
</script>
</body>
</html>
i hope you like this article.
Author : Harsukh Makwana

Hi, My name is Harsukh Makwana. i have been work with many programming language like php, python, javascript, node, react, anguler, etc.. since last 5 year. if you have any issue or want me hire then contact me on [email protected]
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK