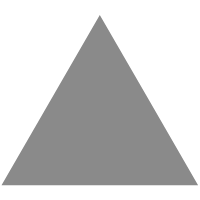
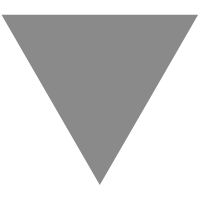
Node.js MySQL Create Database Connection
source link: https://www.laravelcode.com/post/node-js-mysql-create-database-connection
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Node.js MySQL Create Database Connection
MySQL is widely used database management system used in web application. It is popular and easy to connect with any web application.
In this article, we will learn how to connect MySQL data base with Node.js app.
Note: It is assumed that you have already installed MySQL server and Node.js. If you need to install into Linux system, you can download and install from MySQL and Node.js official website.
After successfully installed Node.js and MySQL, we need to install mysql driver for Node.js to interact with database. We will use npm package manager to install node.js packages.
You might want to create package.json file to save all dependencies installed in the package. You can run following command and input the few questions asked about application.
npm init -y
Now install mysql package using below command.
npm install mysql
This will create node_modules directory with all node package installed in it. package.json file saves all dependencies installed in the application.
Now create server.js file, in which we will connect mysql database with Node.js. In the server.js file first import mysql module using require() method.
const mysql = require('mysql');
Now, create mysql.createConnection()
method and pass MySQL credentials object into it.
const conn = mysql.createConnection({
host: 'localhost',
port: 3306,
database: 'mydbname',
user: 'root',
password: 'secret'
});
Now, we will connect database using connect()
method.
conn.connect((err) => {
if (err) {
// throw err;
console.log(err.message);
} else {
// connection established
console.log('Connected with mysql database.');
}
});
You may close the connection programmatically using end()
method.
conn.end(function(err) {
if (err) {
// throw err;
console.log(err.message);
} else {
console.log('Connection with mysql closed.');
}
});
Or close the connection forcefully using destroy()
method.
conn.destroy();
So far in this article, we have connected MySQL database with Node.js. In the later articles, we will query to MySQL database from Node.js.
Author : Harsukh Makwana

Hi, My name is Harsukh Makwana. i have been work with many programming language like php, python, javascript, node, react, anguler, etc.. since last 5 year. if you have any issue or want me hire then contact me on [email protected]
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK