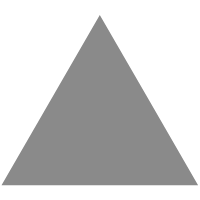
2
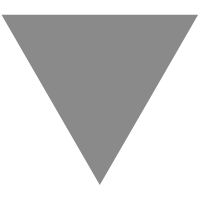
Go 项目实战-获取多级分类下的全部商品
source link: https://studygolang.com/articles/35802
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
备注:前面项目中用到的代码已经分享到
GitHub
中去了,并且以后所有项目中会出现的代码都会提交上去,欢迎查阅。
- 传入任意分类编号,获取该分类下所有子分类的分类编号
- 通过获取的分类编号列表,查询对应的商品数据
接下来咱们就来实现以上需求:
第一步:获取指定分类下的所有子分类编号
func (Category) GetCategoryIds(DB *gorm.DB, cateId int64) (pids map[int]int64) {
if cateId == 0 {
return
}
var Result []*Category
// 查询全部的分类编号和pid
DB.Select([]string{"id", "pid"}).Find(&Result)
index := 0
pids = make(map[int]int64)
// 递归遍历指定分类下的全部子分类编号
// 通过匿名函数的方式进行递归算法
var inPids func(Result []*Category, cateId int64)
inPids = func(Result []*Category, cateId int64) {
if Result == nil {
return
}
for _, item := range Result {
// 判断pid 和传入的 分类编号 相等
if item.Pid == cateId {
pids[index] = item.ID
index++
// 递归传入下一个的分类编号
inPids(Result, item.ID)
}
}
}
// 初始化
inPids(Result, cateId)
return
}
第二步:对获取到的分类编号数组(hashMap)进行处理
第一步获取到的结果集是
hashMap
数组,无法在gorm
中直接使用,需要进行处理:处理成'1','2','3','4'
这样的字符串才可以直接使用进行查询!
var GoodsResult []*model.Goods
var resErr error
if len(pids) > 0 {
// 定义 bytes Buffer 变量
pidsByte := new(bytes.Buffer)
for _, value := range pids {
// 通过fmt.fmt.Fprintf 进行格式化赋值处理
_, err := fmt.Fprintf(pidsByte, "'%s',", strconv.FormatInt(value, 10))
if err != nil {
return
}
}
pidsString := pidsByte.String()
// 过滤,最后两个字符(',)
pidsString = pidsString[0 : len(pidsString)-2]
// 过滤,第一个字符(')
pidsString = pidsString[1:]
// 查询结果
resErr = DB.Where("goods_cate in (?)", pidsString).Order("created_at desc").Find(&GoodsResult).Error
} else {
resErr = DB.Order("created_at desc").Find(&GoodsResult).Error
}
// 获取数据总数量
count := DB.Find(&model.Goods{}).RowsAffected
if resErr != nil {
utils.Fail(ctx, resErr.Error(), nil)
return
}
utils.Success(ctx, "获取成功", gin.H{
"count": count,
"data": GoodsResult,
})
到了这一步,咱们就实现了上面需求的功能。 更多功能请持续关注!!!!!
星球地址:https://t.zsxq.com/03MJM7YfI
关注公众号「程序员小乔」
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK