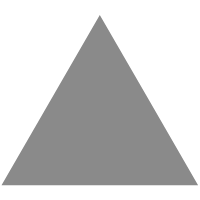
7
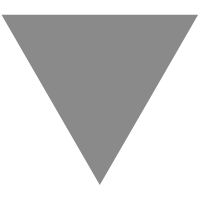
Mybatis实现oracle批量插入及分页查询
source link: https://blog.51cto.com/825272560/5431186
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Mybatis实现oracle批量插入及分页查询
推荐 原创1、单条数据insert
<!--简单SQL-->
insert into userinfo (USERID, USERNAME, AGE) values(1001,'小明',20);
<!--Mybatis写法1,有序列,主键是自增ID,主键是序列-->
<insert id="insert" parameterType="com.zznode.modules.bean.UserInfo">
<selectKey resultType="java.lang.Integer" order="BEFORE" keyProperty="userid">
SELECT userinfo_userid_seq.nextval as userid from dual
</selectKey>
insert into EPG_ALARM_INFO (USERID, USERNAME, AGE)
values (#{userid}, #{username}, #{age})
</insert>
<!--Mybatis写法2,无序列,主键是uuid,字符串-->
<insert id="insert" parameterType="com.zznode.modules.bean.UserInfo">
insert into EPG_ALARM_INFO (USERID, USERNAME, AGE, TIME)
values (#{userid}, #{username}, #{age}, sysdate)
</insert>
2、批量数据批量insert
- insert all into 的方式返回值由最后的select 决定
<!--简单SQL, 方法1-->
INSERT ALL
INTO userinfo (USERID, USERNAME, AGE) values(1001,'小明',20)
INTO userinfo (USERID, USERNAME, AGE) values(1002,'小红',18)
INTO userinfo (USERID, USERNAME, AGE) values(1003,'张三',23)
select 3 from dual;
<!--简单SQL, 方法2-->
begin
insert into userinfo (USERID, USERNAME, AGE) values(1001,'小明',20);
insert into userinfo (USERID, USERNAME, AGE) values(1001,'小红',18);
insert into userinfo (USERID, USERNAME, AGE) values(1001,'张三',23);
end;
<!--简单SQL, 方法3-->
insert into userinfo (USERID, USERNAME, AGE)
select 1001, '小明', 20 from dual union all
select 1002, '小红', 18 from dual union all
select 1003, '张三', 23 from dual
<!--Mybatis写法1,无序列-->
<insert id="insertBatch" parameterType="java.util.List">
INSERT ALL
<foreach collection="list" index="index" item="item">
INTO userinfo (USERID, USERNAME, AGE)
VALUES (#{item.userid}, #{item.username}, #{item.age})
</foreach>
select list.size from dual
</insert>
<!--Mybatis写法2,无序列-->
<insert id="insertBatch">
insert into EPG_ALARM_INFO (USERID, USERNAME, AGE)
<foreach collection="list" item="item" index="index" separator="union all">
<!-- <foreach collection="list" item="item" index="index" separator="union all" open="(" close=")"> -->
<!-- (select #{item.userid}, #{item.username}, #{item.age} from dual) -->
<!-- 上面带括号,下面不带括号,都可以,少量数据不带括号效率高 -->
select #{item.userid}, #{item.username}, #{item.age} from dual
</foreach>
</insert>
<!--Mybatis写法3,有序列-->
<insert id="insertBatch">
insert into EPG_ALARM_INFO (USERID, USERNAME, AGE)
SELECT userinfo_userid_seq.nextval, m.* FROM (
<foreach collection="list" item="item" index="index" separator="union all">
select #{item.username}, #{item.age} from dual
</foreach>
) m
</insert>
3、创建序列
-
minvalue n (/nominvalue):最小值为n
-
maxvalue n (/nomaxvalue):最大值为n
-
start with n:从n开始计数
-
increment by n:每次增加n
-
cache n (/nocache):缓存n个sequence值 / 不缓存,如果缓存,则会有跳号的危险
-
noorder (/order):不保证序列号按顺序生成请求
-
cycle n (/nocycle):如果到达最大值n后,再次从start with n开始
-
currval:序列的当前值,新序列必须使用一次nextval 才能获取到值,否则会报错
-
nextval:表示序列的下一个值。新序列首次使用时获取的是该序列的初始值,从第二次使用时开始按照设置的步进递增
-
删除序列语法: drop sequence seq_表名
<!-- create sequence 序列名 increment by 1 --每次增加几个,我这里是每次增加1 start with 1 --从1开始计数 nomaxvalue --不设置最大值 nocycle --一直累加,不循环 nocache; --不建缓冲区 在插入语句中调用:序列名.nextval 生成自增主键。 --> <!--创建序列--> create sequence SEQ_USERINFO minvalue 1 maxvalue 9999999999 start with 1 increment by 1 nocache; <!--删除序列--> drop sequence SEQ_USERINFO
4、oracle分页查询
前端与后端交互,分页查询
- serviceImpl业务代码
public List<TBadUserW> queryPageBadUserInfo(TbadUserQuery queryModel) {
log.info("分页查询请求参数,{}", JSON.toJSONString(queryModel));
int pageNum = queryModel.getPageNum(); // 开始页
int pageSize = queryModel.getPageSize(); // 每页数量
queryModel.setStart((pageNum - 1) * pageSize); // 开始行数 (+1后)
queryModel.setEnd(pageNum * pageSize); // 结束行数
List<TBadUserW> beans = badUserWDao.queryPageBadUserInfo(queryModel);
log.info("最终查询数量:", beans.size());
return beans;
}
- mapper.xml文件
<select id="queryPageInfo" parameterType="com.zznode.test.bean.TbadUserQuery"
resultMap="BaseResultMap" >
SELECT tt.* FROM
(
<!--前端分页需要 total总记录-->
SELECT t.*, ROWNUM rown, COUNT (*) OVER () total FROM
(
select <include refid="Base_Column_List"/> from T_BAD_USER_W
<where>
<if test="city != null and city !=''">
and city = #{city}
</if>
<if test="county != null and county != ''">
and county = #{county}
</if>
<if test="startTime != null and startTime !=''">
and loadtime >= to_date(#{startTime} , 'yyyy-mm-dd hh24:mi:ss')
</if>
<if test="endTime != null and endTime !=''">
and loadtime <![CDATA[<=]]> to_date(#{endTime} , 'yyyy-mm-dd hh24:mi:ss')
</if>
</where>
)t
)tt
where tt.rown > #{start} and tt.rown <![CDATA[<=]]> #{end}
</select>
后端海量数据导出,批量查询
-
service业务逻辑编写
public List<TBadUserW> queryPageBadUserInfo(TbadUserQuery queryModel) { log.info("分页查询请求参数,{}", JSON.toJSONString(queryModel)); List<TBadUserW> result = new ArrayList<>(); int pageNum = queryModel.getPageNum(); // 开始页 int pageSize = queryModel.getPageSize(); // 每页数量(可以每页设置为200/500/1000),每次查询的条数 boolean searchAll = true; while (searchAll){ queryModel.setStart((pageNum - 1) * pageSize); // 开始行数 (+1后) queryModel.setEnd(pageNum * pageSize); // 结束行数 List<TBadUserW> beans = badUserWDao.queryPageBadUserInfo(queryModel); if (null == beans || beans.size() < pageSize) { searchAll = false; } if (CollectionUtils.isNotEmpty(beans)) { result.addAll(beans); } pageNum++; } log.info("最终查询数量:", result.size()); return result; }
-
mapper.xml文件编写
<!--这种写法是比较高效的分批查询方法,分批不需要查询total总量,不支持total-->
<select id="queryPageInfo" parameterType="com.zznode.test.bean.TbadUserQuery"
resultMap="BaseResultMap" >
SELECT tt.* FROM
(
SELECT t.*, ROWNUM rown FROM
(
select <include refid="Base_Column_List"/> from T_BAD_USER_W
<where>
<if test="city != null and city !=''">
and city = #{city}
</if>
<if test="county != null and county != ''">
and county = #{county}
</if>
<if test="startTime != null and startTime !=''">
and loadtime >= to_date(#{startTime} , 'yyyy-mm-dd hh24:mi:ss')
</if>
<if test="endTime != null and endTime !=''">
and loadtime <![CDATA[<=]]> to_date(#{endTime} , 'yyyy-mm-dd hh24:mi:ss')
</if>
</where>
)t where ROWNUM <![CDATA[<=]]> #{end}
)tt
where tt.rown > #{start}
</select>
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK