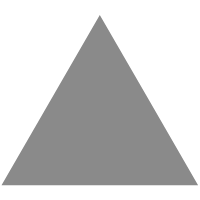
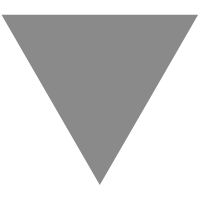
如何从0开发一个Vue组件库并发布到npm - 贵贵子
source link: https://www.cnblogs.com/zdsdididi/p/16392869.html
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
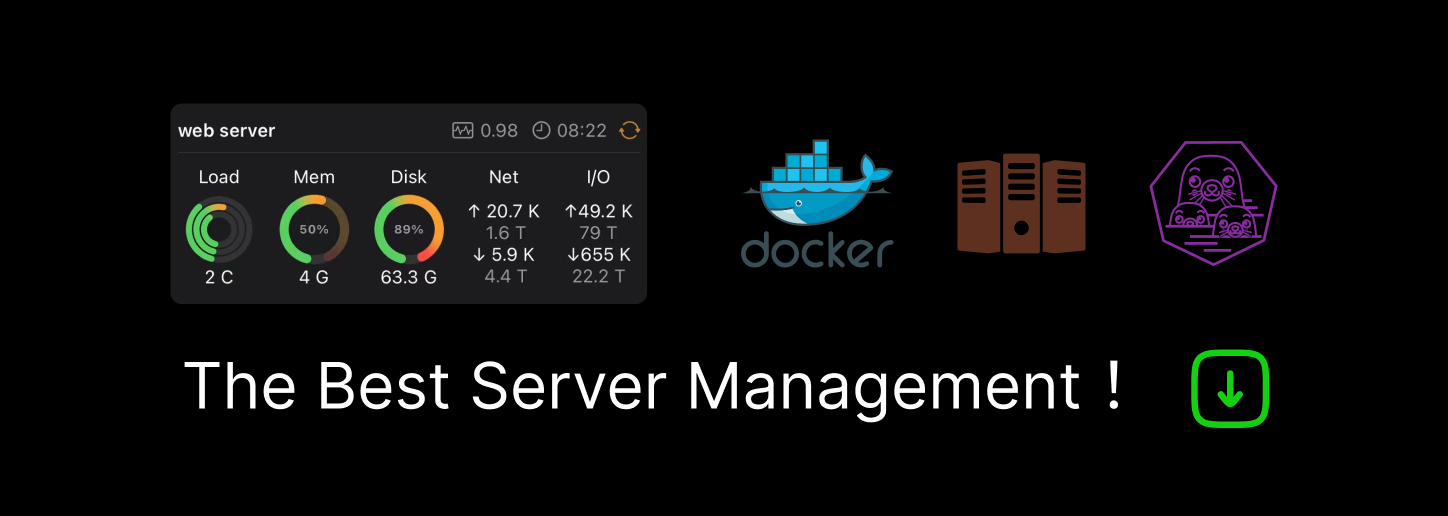
1、新建文件夹在终端打开执行 npm init -y
生成package.json如下,注意如果要发布到npm,name不能有下划线,大写字母等
{
"name": "vuecomponentdi",
"version": "1.0.0",
"description": "",
"main": "index.js",
"scripts": {
"test": "echo \"Error: no test specified\" && exit 1"
},
"keywords": [],
"author": "",
"license": "ISC"
}
2、建立目录结构
目录结构如下
-- vueComponentDi
-- packages
-- button
-- index.js
-- index.vue
-- toast
-- index.js
-- index.vue
-- index.js
-- package.json
3、本地调试
- vueComponentDi/index.js
export default function(){
console.log('本地调试')
}
- 新建一个vue项目
vue create testvue
在testvue下的package.json下的测试依赖devDependencies添加vueComponentDi/index.js绝对地址
"devDependencies": {
...
"vuecomponentdi": "F:/vueComponent@Di/vueComponentDi",//根据自己实际项目地址填写
...
}
- 执行npm link
在testvue执行npm link将vuecomponentdi软链接到node_modules中
- vuecomponentdi安装Eslint
由于testvue引入组件会进行Eslint检测,不安装会报错(testvue关闭Eslint可省略这一步)
安装方法:
npm install [email protected] --save-dev
./node_modules/.bin/eslint --init
- 在testvue使用vuecomponentdi
import test from "vuecomponentdi"
<template>
<div class="home">
<img alt="Vue logo" src="../assets/logo.png">
<HelloWorld msg="Welcome to Your Vue.js App"/>
</div>
</template>
<script>
// @ is an alias to /src
import HelloWorld from '@/components/HelloWorld.vue'
import test from "vuecomponentdi"
export default {
name: 'Home',
components: {
HelloWorld
},
created(){
test()
}
}
</script>
控制台打印>本地调试
4、开发一个button组件
- button模块:进入vueComponentDi/packages/button/index.vue
type只支持传入primary属性.
v-on = "$listemers"表示包含了父作用域中的(不含.native修饰符的) v-on事件监听器,它可以通过v-on="$listerners"传入内部组件
<template>
<div>
<button class="di-button" v-on="$listeners" :class="[type?`di-button--${type}`:'']"><slot></slot></button>
</div>
</template>
<script>
export default {
name:"di-button",
props:{
type:String
}
}
</script>
<style>
.di-button{
display: inline-block;
line-height: 1;
white-space: nowrap;
cursor: pointer;
background: #fff;
border: 1px solid #dcdfe6;
color: #606266;
-webkit-appearance: none;
text-align: center;
box-sizing: border-box;
outline: none;
margin: 0;
transition: .1s;
font-weight: 500;
-moz-user-select: none;
-webkit-user-select: none;
-ms-user-select: none;
padding: 12px 20px;
font-size: 14px;
border-radius: 4px;
}
.di-button:focus, .di-button:hover {
color: #409eff;
border-color: #c6e2ff;
background-color: #ecf5ff;
}
.di-button:active {
color: #3a8ee6;
border-color: #3a8ee6;
outline: none;
}
.di-button--primary {
color: #fff;
background-color: #409eff;
border-color: #409eff;
}
.di-button--primary:focus, .di-button--primary:hover {
background: #66b1ff;
border-color: #66b1ff;
color: #fff;
}
.di-button--primary.is-active, .di-button--primary:active {
background: #3a8ee6;
border-color: #3a8ee6;
color: #fff;
}
</style>
- button模块导出:进入vueComponentDi/packages/button/index.js
如果导出一个带有install函数的对象,则在Vue2中可以直接使用Vue.use(xx)调用此函数,既执行 Vue.component(name,option)创建了一个组件
import button from "./index.vue"
button.install=(Vue)=>{
Vue.component(button.name,button)
}
export default button
- 聚合导出button:进入vueComponentDi/index.js
因为开发的组件不止一个,所以需要在入口文件统一导出
import diButton from "./packages/button"
export {
diButton
}
- 在testvue使用
<template>
<div class="home">
<di-button type="primary">按钮</di-button>
</div>
</template>
<script>
// @ is an alias to /src
import Vue from 'vue'
import {diButton} from "vuecomponentdi"
Vue.use(diButton)
export default {
name: 'Home'
}
</script>
5、开发一个toast弹窗
- toast模块:vueComponentDi/packages/toast/index.vue
type只支持warning和success
<template>
<div class="di-toast" :class="`di-toast--${type}`" v-if="show">
{{message}}
</div>
</template>
<script>
export default {
data(){
return {
message:'',
show:false,
type:''
}
}
}
</script>
<style>
.di-toast{
width: 60%;
width: 200px;
background: rgb(15, 15, 15);
padding:3px;
text-align: center;
color: #fff;
border-radius: 10px;
position: fixed;
left: calc(50% - 100px);
top: 200px;
}
.di-toast--warning{
background: #FDF6EC;
color: #E6A28B;
}
.di-toast--success{
background: #F0F9EB;
color: #93C26D;
}
</style>
- toast模块导出:vueComponentDi/packages/toast/index.js
因为toast弹窗需要在vue中支持this.$toast调用,所以用了Vue.extend方法,这个 API 在日常开发中很少使用,一般在开发组件的时候它才能派上用场,官方定义:使用基础 Vue 构造器,创建一个“子类”。参数是一个包含组件选项的对象
import toast from './index.vue'
toast.install = (Vue) => {
const toastConstructor = Vue.extend(toast);//使用基础 Vue 构造器,创建一个“子类”。参数是一个包含组件选项的对象。
let $vm = new toastConstructor();//将这个子类实例化
let $el = $vm.$mount().$el;//$vm执行$mount()手动挂载会返回一个带有$el的对象,$el就是一个dom对象
document.querySelector("body").appendChild($el);//将这个dom对象添加到body中
//在Vue原型上注入$toast方法
Vue.prototype.$toast = (option) => {
$vm.show = true
if (!(option instanceof Object)) {
//如果传的不是对象直接弹出
$vm.message = option
} else {
$vm.message = option.message
$vm.type = option.type
}
setTimeout(() => {
$vm.show = false
}, 2000)
}
}
export default toast
- 聚合导出:vueComponentDi/index.js
import diButton from "./packages/button"
import toast from "./packages/toast"
export {
diButton,
toast
}
- vuetest中使用toast
<template>
<div class="home">
<di-button type="primary" @click="toast">按钮</di-button>
</div>
</template>
<script>
// @ is an alias to /src
import Vue from "vue";
import { diButton, toast } from "vuecomponentdi";
Vue.use(diButton);
Vue.use(toast);
export default {
name: "Home",
methods: {
toast() {
// this.toast("这是一个普通弹窗");
// this.$toast({
// message: "这是一个成功弹窗",
// type: "success",
// });
this.$toast({
message: "这是一个警告弹窗",
type: "warning",
});
},
},
};
</script>
6、发布到npm
组件开发完成需要发布到npm以便于别人使用;因为发布的是公有包,所以需要在vueComponentDi/package.json中配置
"publishConfig": {
"access": "public"
},
完整package.json:
{
"name": "vuecomponentdi",
"version": "1.0.0",
"description": "",
"main": "index.js",
"scripts": {
"test": "echo \"Error: no test specified\" && exit 1"
},
"keywords": [],
"author": "",
"license": "ISC",
"devDependencies": {
"eslint": "^6.7.2",
"eslint-plugin-vue": "^8.2.0"
},
"publishConfig": {
"access": "public"
}
}
npm发布很简单,只需要两个命令:
npm login
npm publish
执行npm login需要你有npm账号,可以到 npm官网 注册
发布完成之后就可以在任何一个vue2项目中安装使用了:
npm install vuecomponentdi
git地址: vue组件开发
Recommend
-
122
组件化是长期开发过程中一个提炼精华的过程,目的主要是以下几点: 提高复用性 解耦 提升未来的开发效率 那么如何达到这样的效果呢,我们可以分几步来循序渐进地完成。 下文主要是思路,想直接获取代码可转战ElementUI的Github去看源码 一 组件的定
-
43
你的父母是在多大年纪有了你呢?你又是在多大年纪有了宝宝呢?想必很多父母报出的数字都在我们所能接受的范围之内。82岁的著名艺术家韩美林喜得贵子,并晒出一段视频,向大家分享了老来得子的喜悦。比如,意大利著名男高音歌唱家帕瓦罗蒂66岁得子;影星刘
-
43
“为什么我们四线的屌丝,不能在北京实现逆袭”?
-
20
Vue组件库开发:另类通信方式问题:不用Vuex怎么让兄弟组件便捷通信?甚至让业务组件和内部组件通信?答案:使用eventHub如果不使用EventHub,我们想让父组件的两个子组件,甚至两个孙子组件之...
-
16
作为一名程序员,没有过多且复杂的过往,兜兜转转的经历都是围绕着 编程 这...
-
6
编者按:本文来自微信公众号 “显微故事”(ID:xianweigushi) ,作者:显微故事编辑部,36氪经授权发布。
-
6
TransX 一个小巧玲珑的 vue 组件切换动画库发布于 10 月 28 日TNTWeb - 全称腾讯新闻前端团队,组内小伙伴在Web前端、NodeJS开发、UI设计、移动APP等大...
-
5
刘媛媛:寒门贵子 已置顶 |...
-
9
为什么要用lerna 将大型代码仓库分割成多个独立版本化的 软件包(package)对于代码共享来说非常有用。但是,如果某些更改 跨越了多个代码仓库的话将变得很 麻烦 并且难以跟踪,并且, 跨越多个代码仓库的测试将迅速变得非常复杂。
-
6
1、新建文件夹在终端打开执行 npm init -y生成package.json如下,注意如果要发布到npm,name不能有下划线,大写字母等
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK