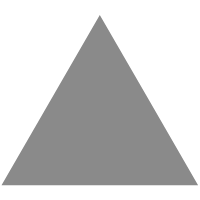
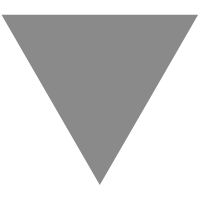
For Loop with two variables in Python
source link: https://thispointer.com/for-loop-with-two-variables-in-python/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
In this Python tutorial, you will learn to use for loop with two variables.
Table Of Contents
Introduction
In python, for loop is used to iterate elements from an iterable or sequence. The iterable can be a list, tuple, set, or dictionary. A for loop iterates over a sequence of elements in the iterable.
Syntax:
for iterator in iterable: statement 1 statement 2 ....... ....... statement n
Using for loop with two variables to iterate over dictionary.
A Dictionary is a key-value pair that stores data in the form of keys and values. So by using the items() method, we can iterate over keys and values pairs at a time using iterators inside for loop.
Advertisements
Syntax:
for key_iterator, value_iterator in dictionary_data.items(): print(key_iterator,":",value_iterator)
Here,
1. key_iterator is used to return keys by iterating keys in a dictionary.
2. value_iterator is used to return values by iterating values in a dictionary.
Let’s dive into an example, to understand it better!!
In this example, we are creating a dictionary with 5 items (key: value pairs). It will display keys and values by using for loop with two iterators(key_iterator, value_iterator).
# Create dictionary with 5 items dictionary_data = { 1: "welcome", 2: "to", 3: "thispointer", 4: "python", 5:"tutorial"} # Iterate over keys and values from a dictionary for key_iterator, value_iterator in dictionary_data.items(): print(key_iterator,":",value_iterator)
Output:
1 : welcome 2 : to 3 : thispointer 4 : python 5 : tutorial
We can see that both keys and values are returned with a single for loop.
Using for loop with two variables with zip().
In Python, the zip() function is used to iterate one or more iterables at a time.
Syntax:
for iterator1, iterator2 in zip(data1,data2): print(iterator1, iterator2)
Here,
- data1, data2 are the iterables(lists).
- The iterator1 is used to iterate elements in data1 and iterator2 is used to iterate elements in data2.
By using zip() with for loop, we can combine these two lists and get the data from the two lists at a time. Let’s dive into an example, to understand it better!!
In this example, we are creating two lists with 5 elements each and display all elements from both lists by looping through them.
# Create data1 with 5 items data1 = [1,2,3,4,5] #Create data2 with 5 items data2 = ["welcome","to","thispointer","python","tutorial"] # Iterate over all elements from both the lists for iterator1, iterator2 in zip(data1,data2): print(iterator1, iterator2)
Output:
1 welcome 2 to 3 thispointer 4 python 5 tutorial
In the above code, we combined two iterators using zip().
Example 2:
In this example, we are creating three lists and using for loop with three variables with zip().
# create data1 with 5 items data1 = [1, 2, 3, 4, 5] # create data2 with 5 items data2 = ["welcome", "to", "thispointer", "python", "tutorial"] # create data3 with 5 items data3 = ["welcome", "to", "thispointer", "c++", "tutorial"] # Iterate over all elements from all the lists for iterator1, iterator2,iterator3 in zip(data1,data2,data3): print(iterator1, iterator2,iterator3)
Output:
1 welcome welcome 2 to to 3 thispointer thispointer 4 python c++ 5 tutorial tutorial
From the above output, we can see that all the variables are combined.
Using for loop with two variables with enumerate().
In Python, the enumerate() function is used to return the index along with the element used for the loop in an iterable. Here, we can iterate two variables in for loop with iterators.
Syntax:
for iterator1, iterator2 in enumerate(data2): print(data1[iterator1],iterator2)
Here,
- data1, data2 are the iterables(lists)
- iterator1 is used to iterate elements in data1 and iterator2 is used to iterate elements in data2.
Let’s dive into an example, to understand it better!!
In this example, we are creating two lists with 5 elements each and display all elements from both lists by looping through them.
# create data1 with 5 items data1 = [11, 22, 33, 44, 55] # create data2 with 5 items data2 = ["welcome", "to", "thispointer", "python", "tutorial"] # Iterate over all elements from all the lists for iterator1, iterator2 in enumerate(data2): print(data1[iterator1], iterator2)
Output:
11 welcome 22 to 33 thispointer 44 python 55 tutorial
In the above code, we are displaying the elements present in data1 and data2. As we know the first variable (iterator) displays the index, so we used [] to get elements present at each index.
Summary
By using zip() and enumerate(), we can iterate with two variables in a for loop. We can also iterate with two elements in a dictionary directly using for loop.
Pandas Tutorials -Learn Data Analysis with Python
Are you looking to make a career in Data Science with Python?
Data Science is the future, and the future is here now. Data Scientists are now the most sought-after professionals today. To become a good Data Scientist or to make a career switch in Data Science one must possess the right skill set. We have curated a list of Best Professional Certificate in Data Science with Python. These courses will teach you the programming tools for Data Science like Pandas, NumPy, Matplotlib, Seaborn and how to use these libraries to implement Machine learning models.
Checkout the Detailed Review of Best Professional Certificate in Data Science with Python.
Remember, Data Science requires a lot of patience, persistence, and practice. So, start learning today.
Join a LinkedIn Community of Python Developers
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK