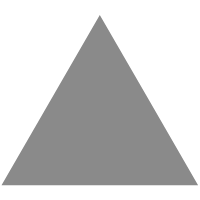
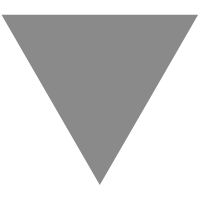
Web Accessibility in React
source link: https://www.jsnow.io/p/javascript/react/web-accessibility-in-react
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
19/5/2022
Web Accessibility in React

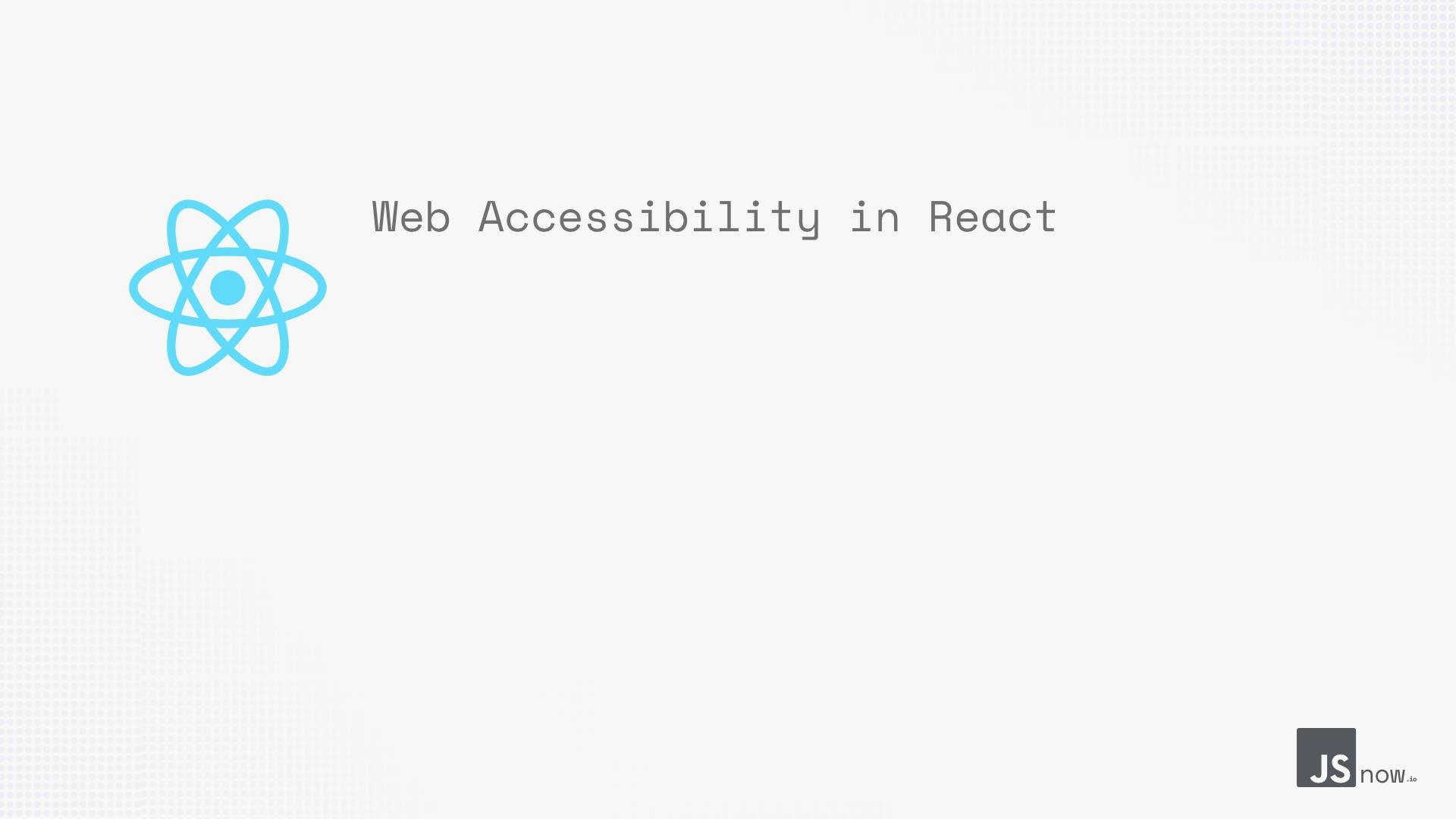
Web accessibility is the design and creation of websites that everyone can use. Accessibility support is necessary to allow assistive technology to interpret web pages primarily for people with disabilities.
Why Web Accessibility is Important
Web accessibility provides equal access to everyone, especially people with disabilities. In addition, web accessibility enhances usability and often results in a more intuitive user experience. Therefore, online content that meets accessibility requirements is likely user-friendly for everyone. Also, It is a requirement by the law to make the web accessible to everyone.
This article will dive deeper into how React makes the web-accessible.
Making tags accessible
Title
When your react app is rendered correctly, The <title> element is the first thing the user sees. The title tag is usually found in the public/index.html file. This is because React apps are single-page applications (SPAs), you'll see the same browser title every time, and it's not optimal. You can assign a document title property that will work globally inside the componentWillMount() lifecycle method to set the title element's text dynamically. This will function once the page has fully loaded. When your react app is rendered correctly, The <title> element is the first thing the user sees. The title tag is usually found in the public/index.html file.
Because React apps are single-page applications (SPAs), you'll see the same browser title every time, and it's not optimal. You can assign a document title property that will work globally inside the componentWillMount() lifecycle method to set the title element's text dynamically. This function will run once the page has fully loaded.
componentWillMount() {
document.title = "Home";
}
Another option is to set the document title by using the useEffect hook. useEffect hook executes at the initial stage when the component is mounted. useEffect also runs when the components state changes or the component re-renders.
We can simply set the document title inside the useEffect hook, as shown below.
import React, { useEffect } from "react";
export default const App = () => {
useEffect(() => {
document.title = "Home";
}, []);
return (
<div className="App">
<h1>Hello React App</h1>
</div>
);
}
div vs fragments in React
In a React component, it is necessary to have a parent tag that holds all other elements inside. One way to achieve this is by wrapping them inside a div or span tag. Of course, you can use these tags, but the code will become non-semantic, making the app less accessible. React has added a feature called fragments to deal with this issue. React Fragments are similar to HTML tags like span and div, which wraps child components inside without adding extra nodes to the DOM.
Here's an example of how to use fragments
const App = () => (
<React.Fragment>
<Component></Component>
</React.Fragment>
);
export default App;
Fragments also come with a convenient shorthand representation.
const App = () => (
<>
<Header />
<Navigation />
<Main />
<Footer />
</>
);
export default App
ARIA (Accessible Rich Internet Applications) is a set of attributes intended for web applications that make them accessible to individuals with disabilities.
Consider the following component that displays an input element.
const App = () => (
<label>
First Name
<input type="text" />
</label>
);
export default App;
The aria-label attribute can be used to make this input element more accessible.
const App = () => (
<label>
First Name
<input type="text" aria-label="first name" />
</label>
);
export default App;
The aria-label improves the readability of your code for both developers and screen readers.
Keyboard Focus
Keyboard focus refers to the DOM element that gets selected when it receives a keyboard input by a user.
When it comes to boosting a web page's accessibility, using it with a keyboard is a must.
React has a Refs feature that allows you to manage keyboard focus events. Refs are analogous to keys in React. Refs feature can be used when you want to change the value of a child component without passing props from the parent component.
Let's have a look at how the ref function in React works.
We have a NameInput component that renders an input field.
class NameInput extends Component {
render() {
return (
<div>
<input type="text" />
</div>
);
}
}
Now we can add a constructor with the createRef() method in the component.
constructor(props) {
super(props);
this.textRef = React.createRef();
}
Then we can pass the ref attribute to the input element. We've got a reference to the input now.
<input type="text" ref={this.textRef} />
Finally, we will add the focus() method to our input element inside the componetDidMount method.
componentDidMount() {
this.textRef.current.focus()
}
Here's our complete input class component.
class NameInput extends Component {
constructor(props) {
super(props);
this.textRef = React.createRef();
}
componentDidMount() {
this.textRef.current.focus();
}
render() {
return (
<div>
<input type="text" ref={ this.textRef } />
</div>
);
}
}
export default NameInput;
Now when you hit refresh, the input area will automatically be focused.
That is how the focus() method improves the component's accessibility.
React Hooks makes the job easy by providing several ready-to-use keyboard events to implement.
Using The alt Attribute With Images
The alt attribute is the alternate text for an image element if the image can't be retrieved for any reason. This improves the user experience since even if the src attribute fails to load an image, the user will still be able to see an alternate text in place of the image, preventing any confusion.
function User() {
return (
<div>
<img src="ball.png" alt="ball" />
</div>
)
}
export default User;
Using The role Attribute
The role attribute helps in enhancing the semantic behaviour of HTML elements. The role attribute is commonly used to assist screen readers in determining what a feature does and how it works.
Let's say we're using the HTML link element as a button on our website. To specify that the link acts as a button in your application, the role attribute should be used.
function Contact() {
return (
<div>
<a href="contact" role="button"> Contact Me </a>
</div>
)
}
export default Contact;
Conclusion
Web accessibility means that websites, tools, and technologies are designed and developed so people with disabilities can use them. More specifically, people can: perceive, understand, navigate, and interact with the web and contribute to the web.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK