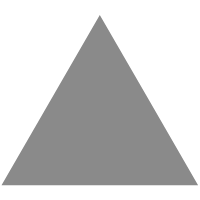
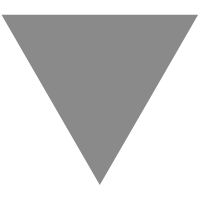
PHP 7 - Upload Files & Images with Example Tutorials
source link: https://www.laravelcode.com/post/php-7-upload-files-images-with-example-tutorials
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
PHP 7 - Upload Files & Images with Example Tutorials
This is a step by step PHP 7 file uploading and storing tutorial. In this tutorial, we will learn how to upload files and images in MySQL Database. and how to implement file upload validation afore sending it to a web server.
The Cyber World has never been a secure place; an army of maleficent hackers may capitalize on your code that can bring astringent damages to your php application.
Afore uploading the file to the server, we must ascertain the compulsory file validation is done correctly. If we do not consider security factors, we might get into trouble.
PHP 7 File Upload Tutorial Objective:
- Display image preview before uploading
- Place all the uploaded images in a specific folder
- Store images path in the MySQL database
Along with that we will also cover the following image validation using PHP 7:
- Check if the real image is uploaded
- Allow only specific file extension such as .jpg, .jpeg or .png
- Make sure file size should not exceed 2MB
- Check if the file already exists
Getting Started
Start MAMP or XAMPP, create the following folder and file structure in htdocs directory.
\-- php-file-upload
|-- config
|--- database.php
|-- img_dir
|--- image-1
|--- image-2
|-- file-upload.php
|-- index.php
Create Database & Table
Create database `database_name`
.
Create `table_name`
inside the database.
You can execute the following command to create the table columns to store the images in the database.
CREATE TABLE `user` (
`id` int(11) NOT NULL,
`file_path` varchar(255) NOT NULL
) ENGINE=InnoDB DEFAULT CHARSET=utf8;
Create File Uploading Form
Create an HTML form that permits our users to select the image from their local device the same image which they want to store onto the server.
The input type should be set to file along with the name property.
Also, define the method=”post” and enctype=”multipart/form-data” tags.
<form action="" method="post" enctype="multipart/form-data" class="mb-3">
<h3 class="text-center mb-5">Upload File in PHP 7</h3>
<div class="user-image mb-3 text-center">
<div style="width: 100px; height: 100px; overflow: hidden; background: #cccccc; margin: 0 auto">
<img src="..." class="figure-img img-fluid rounded" id="imgPlaceholder" alt="">
</div>
</div>
<div class="custom-file">
<input type="file" name="fileUpload" class="custom-file-input" id="chooseFile">
<label class="custom-file-label" for="chooseFile">Select file</label>
</div>
<button type="submit" name="submit" class="btn btn-primary btn-block mt-4">
Upload File
</button>
Display Image Preview
You can show image preview before uploading it to the server with the help of jQuery.
Import jQuery CDN link before the closing body tag.
<!-- jQuery -->
<script src="https://code.jquery.com/jquery-3.5.1.slim.min.js"></script>
We have already declared the image tag with the blank src=”” tag.
<img src="..." id="imgPlaceholder" alt="">
The following function converts the image string and places the base64 url in the HTML img
tag when the user selects the image.
Place the following code right after the jQuery CDN script tag.
<script>
function readURL(input) {
if (input.files && input.files[0]) {
var reader = new FileReader();
reader.onload = function (e) {
$('#imgPlaceholder').attr('src', e.target.result);
}
// base64 string conversion
reader.readAsDataURL(input.files[0]);
}
}
$("#chooseFile").change(function () {
readURL(this);
});
</script>
Database Configuration
Add following code in config/database.php file to make the PHP 7 connection with MySQL database using PDO method.
// config/database.php
<?php
$hostname = "localhost";
$username = "root";
$password = "";
try {
$conn = new PDO("mysql:host=$hostname;dbname=php_crud", $username, $password);
// set the PDO error mode to exception
$conn->setAttribute(PDO::ATTR_ERRMODE, PDO::ERRMODE_EXCEPTION);
//echo "Database connected successfully";
} catch(PDOException $e) {
echo "Database connection failed: " . $e->getMessage();
}
?>
File/Image Upload Validation in PHP 7
Let us add our first validation with the help of the file_exists() PHP method. Display error message to the user if he does not select any image.
To address the errors, we set up a $resMessage
array with status and message properties. We will display the messages to the user based on the response on the front-end.
if (!file_exists($_FILES["fileUpload"]["tmp_name"])) {
$resMessage = array(
"status" => "alert-danger",
"message" => "Select image to upload."
);
}
The following code applies the validation that allows specific file type such as .jpg, jpeg and .png to be uploaded on the server. You won’t be allowed other file types, and will be displayed error message if you do so.
// Get file extension
$imageExt = strtolower(pathinfo($target_file, PATHINFO_EXTENSION));
// Allowed file types
$allowd_file_ext = array("jpg", "jpeg", "png");
else if (!in_array($imageExt, $allowd_file_ext)) {
$resMessage = array(
"status" => "alert-danger",
"message" => "Allowed file formats .jpg, .jpeg and .png."
);
}
Set up image file size limit using the following code. A user can not allow more than 2MB size of image to upload.
else if ($_FILES["fileUpload"]["size"] > 2097152) {
$resMessage=array("status"=> "alert-danger",
"message"=> "File is too large. File size should be less than 2 megabytes."
);
}
The given below code checks if the current file is already uploaded.
else if (file_exists($target_file)) {
$resMessage = array(
"status" => "alert-danger",
"message" => "File already exists."
);
}
Create img_dir folder in the root of your PHP upload file project, here we will store all the uploaded images.
<?php
// Database connection
include("config/database.php");
if(isset($_POST["submit"])) {
// Set image placement folder
$target_dir = "img_dir/";
// Get file path
$target_file = $target_dir . basename($_FILES["fileUpload"]["name"]);
if (!file_exists($_FILES["fileUpload"]["tmp_name"])) {
// Validation goes here
} else {
if (move_uploaded_file($_FILES["fileUpload"]["tmp_name"], $target_file)) {
$sql = "INSERT INTO user (file_path) VALUES ('$target_file')";
$stmt = $conn->prepare($sql);
if($stmt->execute()){
$resMessage = array(
"status" => "alert-success",
"message" => "Image uploaded successfully."
);
}
} else {
$resMessage = array(
"status" => "alert-danger",
"message" => "Image coudn't be uploaded."
);
}
}
}
?>
Complete PHP 7 File Upload Example
Complete PHP file upload code example can be found in the file-upload.php file.
// file-upload.php
<?php
// Database connection
include("config/database.php");
if(isset($_POST["submit"])) {
// Set image placement folder
$target_dir = "img_dir/";
// Get file path
$target_file = $target_dir . basename($_FILES["fileUpload"]["name"]);
// Get file extension
$imageExt = strtolower(pathinfo($target_file, PATHINFO_EXTENSION));
// Allowed file types
$allowd_file_ext = array("jpg", "jpeg", "png");
if (!file_exists($_FILES["fileUpload"]["tmp_name"])) {
$resMessage = array(
"status" => "alert-danger",
"message" => "Select image to upload."
);
} else if (!in_array($imageExt, $allowd_file_ext)) {
$resMessage = array(
"status" => "alert-danger",
"message" => "Allowed file formats .jpg, .jpeg and .png."
);
} else if ($_FILES["fileUpload"]["size"] > 2097152) {
$resMessage = array(
"status" => "alert-danger",
"message" => "File is too large. File size should be less than 2 megabytes."
);
} else if (file_exists($target_file)) {
$resMessage = array(
"status" => "alert-danger",
"message" => "File already exists."
);
} else {
if (move_uploaded_file($_FILES["fileUpload"]["tmp_name"], $target_file)) {
$sql = "INSERT INTO user (file_path) VALUES ('$target_file')";
$stmt = $conn->prepare($sql);
if($stmt->execute()){
$resMessage = array(
"status" => "alert-success",
"message" => "Image uploaded successfully."
);
}
} else {
$resMessage = array(
"status" => "alert-danger",
"message" => "Image coudn't be uploaded."
);
}
}
}
?>
Include the file-upload.php in the index.html file here we have defined the file upload form along with the message array.
<?php include("file-upload.php"); ?>
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1, shrink-to-fit=no">
<link rel="stylesheet" href="https://stackpath.bootstrapcdn.com/bootstrap/4.5.0/css/bootstrap.min.css">
<title>PHP 7 Image Upload Example</title>
<style>
.container {
max-width: 450px;
}
</style>
</head>
<body>
<div class="container mt-5">
<form action="" method="post" enctype="multipart/form-data" class="mb-3">
<h3 class="text-center mb-5">Upload File in PHP 7</h3>
<div class="user-image mb-3 text-center">
<div style="width: 100px; height: 100px; overflow: hidden; background: #cccccc; margin: 0 auto">
<img src="..." class="figure-img img-fluid rounded" id="imgPlaceholder" alt="">
</div>
</div>
<div class="custom-file">
<input type="file" name="fileUpload" class="custom-file-input" id="chooseFile">
<label class="custom-file-label" for="chooseFile">Select file</label>
</div>
<button type="submit" name="submit" class="btn btn-primary btn-block mt-4">
Upload File
</button>
</form>
<!-- Display response messages -->
<?php if(!empty($resMessage)) {?>
<div class="alert <?php echo $resMessage['status']?>">
<?php echo $resMessage['message']?>
</div>
<?php }?>
</div>
<!-- jQuery -->
<script src="https://code.jquery.com/jquery-3.5.1.slim.min.js"></script>
<script>
function readURL(input) {
if (input.files && input.files[0]) {
var reader = new FileReader();
reader.onload = function (e) {
$('#imgPlaceholder').attr('src', e.target.result);
}
reader.readAsDataURL(input.files[0]); // convert to base64 string
}
}
$("#chooseFile").change(function () {
readURL(this);
});
</script>
</body>
</html>
i hope you like this tutorials.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK